The Fundamentals of PHP’s count() Function
In the world of web development, PHP stands as one of the most widely used scripting languages. Its versatility and power allow developers to create dynamic and interactive web applications. One of the essential functions in PHP is count(), which plays a pivotal role in array manipulation. In this article, we’ll delve into the fundamentals of the count() function, exploring its syntax, use cases, and providing code samples to illustrate its versatility.
1. Understanding the Basics
At its core, the count() function in PHP serves a simple yet crucial purpose: to determine the number of elements in an array. This function enables developers to dynamically analyze the size of arrays, facilitating informed decision-making when dealing with data manipulation.
1.1. Syntax of count()
The syntax of the count() function is straightforward:
php count(array $array, int $mode = COUNT_NORMAL): int
Here’s what each part of the syntax means:
- array $array: This is the array you want to count the elements of.
- int $mode = COUNT_NORMAL: The optional $mode parameter specifies the behavior of the function. It can take two values: COUNT_NORMAL (default) and COUNT_RECURSIVE. The former counts only the elements of the top-level array, while the latter counts all elements recursively, including those nested in sub-arrays.
2. Use Cases of count()
The applications of the count() function span a wide range of scenarios in web development. Let’s explore some common use cases to understand its significance.
2.1. Counting Items in a Shopping Cart
Consider an e-commerce website with a shopping cart feature. The items selected by a user are stored in an array. The count() function can be employed to determine the total number of items in the cart, allowing the application to display an accurate item count to the user.
php $shoppingCart = ['item1', 'item2', 'item3']; $itemCount = count($shoppingCart); echo "You have $itemCount items in your cart.";
2.2. Validating Input Length
When dealing with user input, such as form submissions, it’s crucial to ensure that certain fields do not exceed a specific length. By utilizing the count() function, developers can effortlessly verify the length of input arrays, preventing data that surpasses predefined limits from being processed.
php $maxNameLength = 50; $userInputName = $_POST['username']; if (count($userInputName) <= $maxNameLength) { // Process the input } else { echo "Username is too long."; }
2.3. Pagination Calculations
In scenarios where data needs to be displayed in pages, such as search results or a list of blog posts, the count() function proves invaluable. It helps in calculating the total number of pages required, given a specific number of items per page.
php $itemsPerPage = 10; $totalItems = count($allItems); $totalPages = ceil($totalItems / $itemsPerPage);
3. Exploring Count with Multi-dimensional Arrays
The count() function can also handle multi-dimensional arrays seamlessly, thanks to the optional $mode parameter. This aspect enhances its utility in scenarios involving complex data structures.
Consider the following example:
php $multiArray = [ 'fruits' => ['apple', 'banana', 'orange'], 'colors' => ['red', 'green', 'blue'], 'numbers' => [1, 2, 3] ]; $totalItems = count($multiArray, COUNT_RECURSIVE); echo "Total items in the multi-dimensional array: $totalItems";
In this instance, the COUNT_RECURSIVE mode ensures that elements within nested arrays are counted as well.
4. Pitfalls and Considerations
While the count() function is undeniably powerful, developers should be aware of certain considerations to avoid potential pitfalls.
4.1. Numeric and Associative Keys
The count() function treats arrays with numeric and associative keys differently. It counts the number of elements in numeric arrays but only the number of top-level elements in associative arrays. For instance:
php $numericArray = [1, 2, 3]; $associativeArray = ['first' => 1, 'second' => 2, 'third' => 3]; $countNumeric = count($numericArray); // Returns 3 $countAssociative = count($associativeArray); // Returns 3
4.2. Nesting Levels
When using COUNT_RECURSIVE mode, keep in mind that nested arrays will contribute to the total count. This might lead to unexpected results if you’re not accounting for nested structures properly.
4.3. Object Counting
While count() primarily operates on arrays, it can also work with objects that implement the Countable interface. This means that custom objects with a count() method can be used with the function.
Conclusion
The count() function in PHP is undoubtedly a cornerstone of array manipulation. Its ability to accurately determine the number of elements within arrays makes it an essential tool for developers across various domains. Whether you’re building e-commerce platforms, validating user input, or calculating pagination, understanding how to utilize the count() function effectively can greatly enhance your coding prowess. Armed with this knowledge, you’re well-equipped to handle array-related challenges and create more efficient and robust PHP applications.
Table of Contents
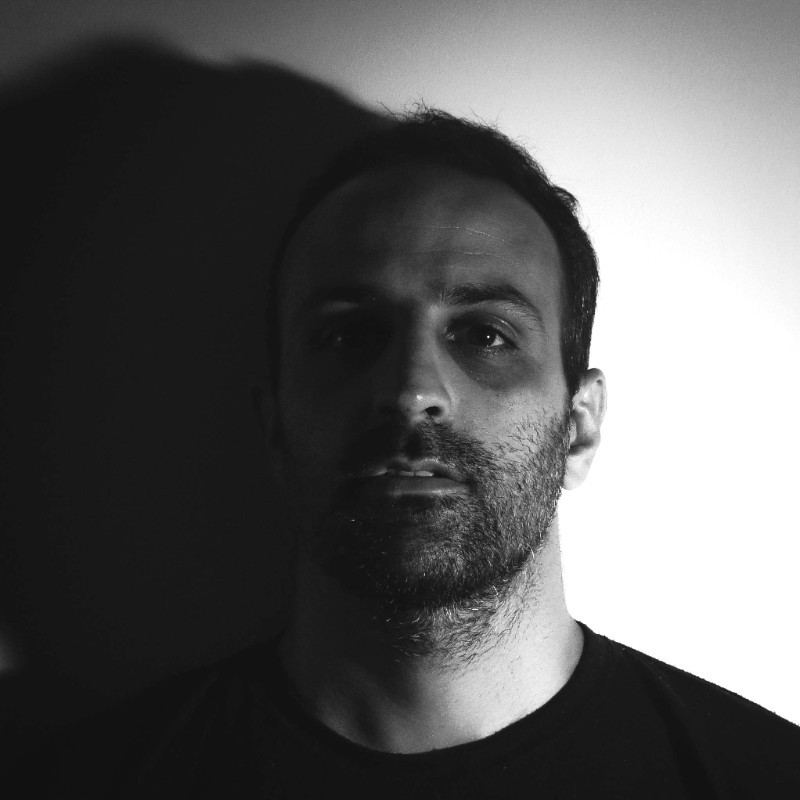
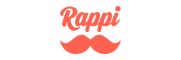