PHP Data Types: A Detailed Exploration
PHP is a versatile and popular programming language used extensively for web development. Understanding data types is crucial for writing efficient and bug-free code. In this blog, we will take a detailed exploration of PHP data types, including strings, integers, floats, arrays, and more. We’ll delve into their properties, usage, and explore code samples to demonstrate their practical applications. By the end of this article, you’ll have a comprehensive understanding of PHP data types and how to utilize them effectively in your projects.
String Data Type
A string is a sequence of characters enclosed in single quotes (”) or double quotes (“”). It allows storing and manipulating textual data. PHP offers numerous functions and operations to work with strings. Let’s explore some code samples to illustrate their usage:
php // Declaring and assigning a string variable $name = "John Doe"; // Concatenation of two strings $greeting = "Hello, " . $name . "!"; // Accessing individual characters in a string $firstCharacter = $name[0]; // Getting the length of a string $length = strlen($name); // Converting a string to uppercase $uppercaseName = strtoupper($name); // Checking if a string contains a specific substring if (strpos($name, "Doe") !== false) { echo "The string contains 'Doe'."; }
Integer Data Type
Integers represent whole numbers without decimal points. They can be positive, negative, or zero. PHP allows performing arithmetic operations, comparisons, and conversions on integer data types. Consider the following code examples:
php // Declaring and assigning an integer variable $age = 25; // Basic arithmetic operations $sum = $age + 5; $difference = $age - 10; $product = $age * 2; $quotient = $age / 5; $remainder = $age % 7; // Increment and decrement operations $age++; $age--; // Comparing two integers if ($age > 18) { echo "You are an adult."; } // Converting a string to an integer $number = intval("123");
Float Data Type
Floats, also known as floating-point numbers or doubles, represent decimal numbers. They are useful for handling mathematical calculations that require precision. Let’s examine some code snippets that demonstrate working with float data types:
php // Declaring and assigning a float variable $price = 9.99; // Basic arithmetic operations $total = $price * 3; $discountedPrice = $price - 2.5; // Rounding a float value $roundedPrice = round($price, 2); // Comparing two floats if ($price > 10.0) { echo "The price is higher than $10."; }
Boolean Data Type
Booleans represent logical values, either true or false. They are commonly used in conditional statements and control flow structures. Here are some examples of working with boolean data types in PHP:
php // Declaring and assigning a boolean variable $isLoggedIn = true; // Conditional statements if ($isLoggedIn) { echo "Welcome, user!"; } else { echo "Please log in to continue."; } // Logical operations $hasPermission = true; $isAdmin = false; if ($hasPermission && !$isAdmin) { echo "Access granted."; } else { echo "Access denied."; }
Array Data Type
Arrays in PHP are versatile and allow storing multiple values in a single variable. They can contain elements of different data types and are incredibly useful for organizing and manipulating data. Let’s explore various operations performed on arrays:
php // Declaring and assigning an array $fruits = array("apple", "banana", "orange"); // Accessing array elements $firstFruit = $fruits[0]; // Adding an element to an array $fruits[] = "grape"; // Updating an element in an array $fruits[1] = "pineapple"; // Removing an element from an array unset($fruits[2]); // Looping through an array foreach ($fruits as $fruit) { echo $fruit . " "; } // Associative arrays $person = array("name" => "John", "age" => 30, "city" => "New York"); echo $person["name"];
Null Data Type
Null represents the absence of a value. It is often used to indicate that a variable has no assigned value. Here’s an example of using the null data type:
php // Declaring and assigning a null variable $car = null; if ($car === null) { echo "No car available."; } else { echo "Car: " . $car; }
Conclusion
In this blog post, we explored the various data types available in PHP, including strings, integers, floats, booleans, arrays, and null. We learned about their properties and saw practical code examples demonstrating their usage. Understanding data types is essential for writing robust and efficient PHP code, and with the knowledge gained from this article, you’ll be better equipped to handle data in your projects. So go ahead, leverage the power of PHP data types, and create amazing web applications!
Table of Contents
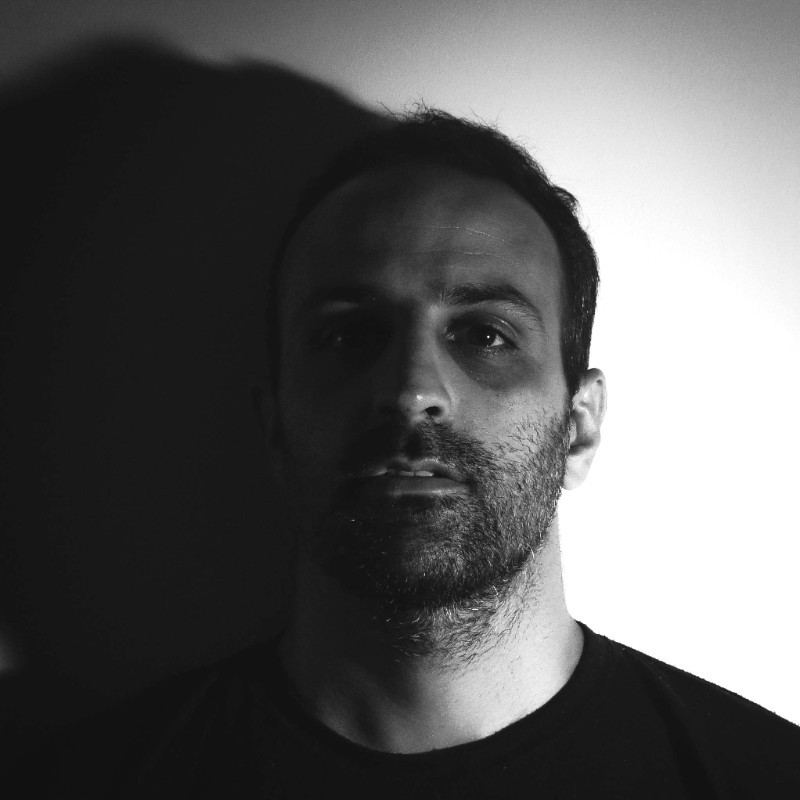
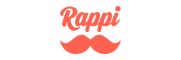