Mastering PHP’s echo and print Statements
When it comes to working with PHP, handling output is an essential skill every developer should master. The ability to display content on a web page or in the command line is fundamental to creating dynamic and interactive applications. In this guide, we’ll dive deep into PHP’s echo and print statements, exploring their differences, best practices, and various use cases. By the end of this tutorial, you’ll have a solid understanding of how to effectively utilize these statements to enhance your PHP programming skills.
1. Understanding Output in PHP
Outputting content is a core aspect of web development. Whether it’s displaying text, variables, or HTML elements, PHP provides several ways to achieve this. The most common methods are the echo and print statements. These statements allow you to send data to the browser or the console, enabling you to interact with your users or debug your code efficiently.
1.1. The echo Statement
The echo statement is one of the simplest ways to display content in PHP. It can handle multiple arguments and doesn’t require parentheses when used with parentheses.
php $name = "John"; echo "Hello, " . $name . "!"; // Output: Hello, John!
You can also use the echo statement with HTML tags to generate dynamic HTML content:
php $color = "blue"; echo "<p style='color: " . $color . ";'>This text is blue.</p>";
1.2. The print Statement
Similar to echo, the print statement is used to output data. However, it has some differences in terms of usage and return value. Unlike echo, the print statement only takes one argument, and it always returns 1, making it slightly slower than echo.
php $age = 25; print "Age: " . $age; // Output: Age: 25
1.3. Key Differences Between echo and print
While both echo and print serve the same purpose, there are a few distinctions to consider:
- Usage: echo can handle multiple arguments separated by commas, while print can only take a single argument.
- Speed: echo is generally faster than print due to its ability to process multiple arguments simultaneously.
- Return Value: echo doesn’t return a value, whereas print returns 1 after successfully outputting its argument.
2. Best Practices for Using echo and print
When using echo and print statements in your PHP code, there are some best practices to keep in mind to ensure clean and efficient output.
2.1. Concatenate Instead of Comma Separation
While both echo and print allow you to separate arguments with commas, it’s recommended to use concatenation for better readability and consistency.
php // Not recommended echo "Hello", " ", "World!"; // Recommended echo "Hello" . " " . "World!";
2.2. Escape Characters
When outputting strings that contain special characters, be sure to use escape characters to maintain proper formatting and prevent unexpected behavior.
php $quote = "This is \"quoted\" text."; echo $quote; // Output: This is "quoted" text.
2.3. Use Short Tags for Variables
When embedding variables within strings, you can use short tags to improve code readability.
php $fruit = "apple"; echo "I love {$fruit}s."; // Output: I love apples.
2.4. HTML and PHP Separation
To ensure clean and maintainable code, it’s advisable to separate PHP logic from HTML content. This makes your codebase more organized and easier to manage.
php // Not recommended echo "<p>Welcome, " . $name . "!</p>"; // Recommended ?> <p>Welcome, <?php echo $name; ?>!</p>
3. Practical Use Cases
Let’s explore some practical use cases for echo and print statements that highlight their versatility and importance in PHP development.
3.1. Dynamic Web Content
PHP’s output statements are extensively used to generate dynamic web content. Whether you’re displaying user-specific information, generating lists from arrays, or embedding variables in HTML templates, echo and print play a pivotal role.
php $userName = "Alice"; $userAge = 30; echo "Welcome, " . $userName . "! You are " . $userAge . " years old.";
3.2. Form Validation and User Feedback
When dealing with forms, providing feedback to users about successful submissions or errors is crucial. echo and print statements allow you to dynamically display messages based on form validation results.
php if ($formValid) { echo "Thank you for submitting the form!"; } else { echo "There was an error in your submission. Please check the form and try again."; }
3.3. Debugging and Development
During development, output statements are valuable for debugging purposes. You can use them to inspect variable values, trace program flow, and identify logical errors.
php $debugValue = "Debug me!"; echo "Debug value: " . $debugValue; // Output: Debug value: Debug me!
3.4. Command Line Applications
PHP isn’t limited to web development; it’s also a capable scripting language. In command line applications, you can use echo and print to display information and interact with users.
php $commandOutput = "Processing complete."; print $commandOutput;
Conclusion
Mastering the echo and print statements in PHP is essential for every developer aiming to build dynamic and interactive web applications. Through this guide, you’ve gained a solid understanding of how these output statements work, their differences, best practices for usage, and various practical scenarios where they shine. With this knowledge in your toolkit, you’re well-equipped to enhance your PHP programming skills and create robust, engaging applications. So go ahead, start implementing echo and print effectively, and elevate your PHP development journey. Happy coding!
Table of Contents
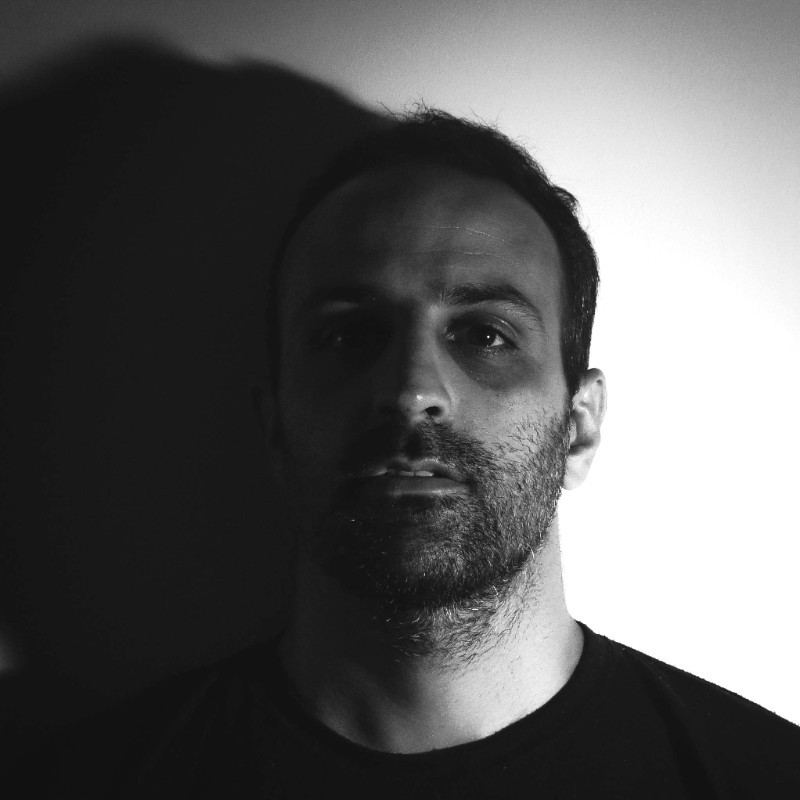
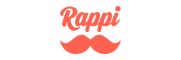