PHP File Handling: Read, Write and Manipulate Files
File handling is a crucial aspect of web development, as it enables interaction with external files to store and retrieve data. PHP, being a server-side scripting language, offers robust file handling capabilities, allowing developers to read, write, and manipulate files effortlessly. In this blog, we will delve into the world of PHP file handling, exploring various techniques and functions to perform file operations effectively.
1. Understanding File Handling in PHP:
File handling in PHP refers to the process of interacting with files stored on the server or any external storage medium. PHP offers various functions and modes to perform file operations securely and efficiently. Let’s start by understanding the concept of file handling and the different modes of file access in PHP.
1.1 What is File Handling?
File handling involves reading, writing, creating, and deleting files in PHP. It enables developers to store data persistently on the server and retrieve it as needed. Common scenarios include saving user data, logging application events, and working with configuration files.
1.2 Modes of File Access:
PHP supports different modes of file access, allowing developers to define how the file should be opened and manipulated. The most commonly used modes are:
- “r”: Read mode – Opens the file for reading only. The file pointer is placed at the beginning of the file.
- “w”: Write mode – Opens the file for writing only. If the file does not exist, it creates a new file. If the file exists, it truncates it to zero length.
- “a”: Append mode – Opens the file for writing only. If the file does not exist, it creates a new file. If the file exists, it appends data to the end of the file.
- “x”: Exclusive mode – Creates a new file for writing only. If the file already exists, fopen() returns FALSE.
- “b”: Binary mode – Enables binary file handling.
2. Reading Files with PHP:
Reading files is a fundamental operation when working with file handling in PHP. PHP provides several functions to read data from files, depending on the specific requirements.
2.1 Using fopen() Function:
The fopen() function is the starting point for reading files. It takes two parameters – the file name (along with the path) and the mode in which to open the file. Let’s see how to use fopen() to read files:
php $filename = 'example.txt'; $file = fopen($filename, 'r'); if ($file) { while (!feof($file)) { $line = fgets($file); // Process the line (e.g., echo or store in an array) } fclose($file); } else { echo "Unable to open file."; }
2.2 Reading Line-by-Line:
The above example reads the entire file, but sometimes you may want to read the file line-by-line to process each line separately. The fgets() function allows you to read one line at a time:
php $filename = 'data.csv'; $file = fopen($filename, 'r'); if ($file) { while (($line = fgets($file)) !== false) { // Process the line (e.g., parse CSV data) } fclose($file); } else { echo "Unable to open file."; }
2.3 Reading Entire File at Once:
If you need to read the entire file content into a single variable, you can use the file_get_contents() function:
php $filename = 'example.txt'; $fileContent = file_get_contents($filename); if ($fileContent !== false) { // Process the entire file content } else { echo "Unable to read file."; }
3. Writing to Files with PHP:
Writing to files is crucial for storing data and updating files on the server. PHP provides methods to create new files and append data to existing ones.
3.1 Creating New Files:
To create a new file and write data into it, use the “w” mode while opening the file with fopen():
php $filename = 'new_file.txt'; $file = fopen($filename, 'w'); if ($file) { $data = "This is the content to be written in the new file."; fwrite($file, $data); fclose($file); echo "File created and data written successfully."; } else { echo "Unable to create file."; }
3.2 Appending Data to Existing Files:
If you want to add data to an existing file without overwriting its content, use the “a” mode:
php $filename = 'existing_file.txt'; $file = fopen($filename, 'a'); if ($file) { $data = "This data will be appended to the existing file."; fwrite($file, $data); fclose($file); echo "Data appended successfully."; } else { echo "Unable to open file."; }
3.3 Writing Data Line-by-Line:
To write data line-by-line to a file, use the file_put_contents() function with the “FILE_APPEND” flag:
php $filename = 'data.txt'; $dataArray = array("Line 1", "Line 2", "Line 3"); foreach ($dataArray as $line) { file_put_contents($filename, $line . PHP_EOL, FILE_APPEND); }
4. Manipulating Files with PHP:
Apart from reading and writing, PHP offers various file manipulation functions to perform tasks like copying, renaming, and deleting files.
4.1 Copying Files:
The copy() function allows you to duplicate a file with a new name and location:
php $sourceFile = 'source.txt'; $destinationFile = 'destination.txt'; if (copy($sourceFile, $destinationFile)) { echo "File copied successfully."; } else { echo "Unable to copy file."; }
4.2 Renaming Files:
To rename a file, use the rename() function:
php $oldName = 'old_file.txt'; $newName = 'new_file.txt'; if (rename($oldName, $newName)) { echo "File renamed successfully."; } else { echo "Unable to rename file."; }
4.3 Deleting Files:
The unlink() function is used to delete files:
php $filename = 'file_to_delete.txt'; if (unlink($filename)) { echo "File deleted successfully."; } else { echo "Unable to delete file."; }
Conclusion
PHP file handling is a powerful feature that enables developers to read, write, and manipulate files with ease. In this blog, we covered various aspects of PHP file handling, including reading files line-by-line, writing data to files, and performing file manipulations. By mastering file handling techniques, you can enhance your web development skills and create applications that effectively interact with external files for data storage and retrieval.
Table of Contents
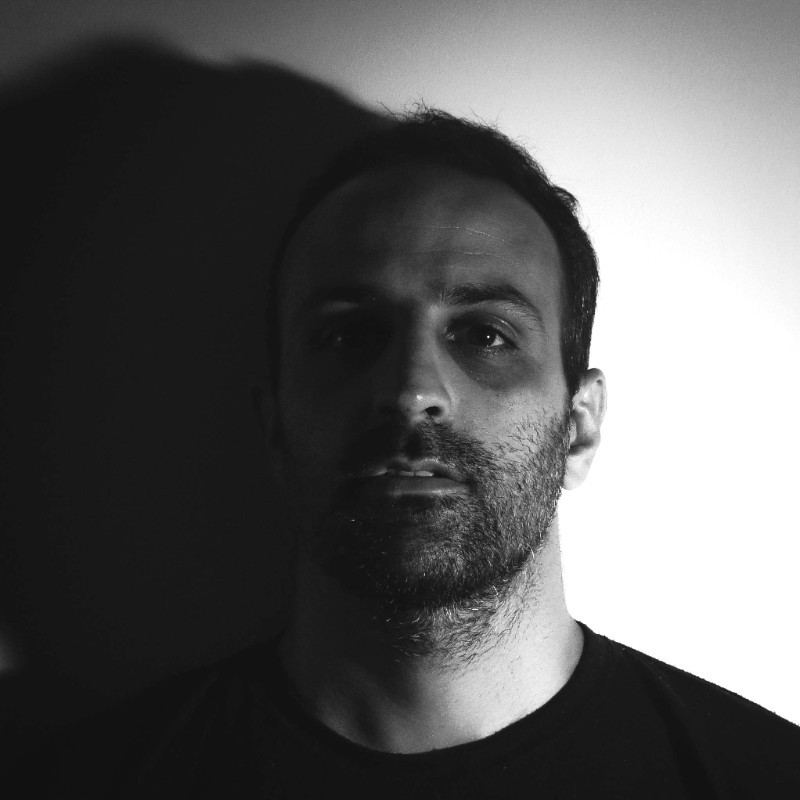
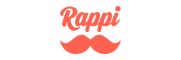