Unleashing the Power of PHP’s file_get_contents()
In the world of web development, PHP stands as a cornerstone of server-side scripting languages. It empowers developers to create dynamic and interactive web applications. One of the often-overlooked functions in PHP’s expansive toolbox is file_get_contents(). This unassuming function, however, holds immense power when harnessed correctly. In this blog, we’ll dive into the capabilities of file_get_contents(), exploring its various applications through real-world examples and code snippets.
1. Understanding file_get_contents()
At its core, file_get_contents() is a PHP function designed to simplify reading files, particularly when dealing with file-based resources and HTTP requests. It allows developers to retrieve the contents of a file as a string, making it a versatile tool for various tasks. Its ease of use and integration into various contexts make it a valuable asset in a developer’s toolkit.
2. Reading Local Files
2.1. Basic Usage
Reading a local file using file_get_contents() is straightforward. Simply provide the function with the file’s path, and it will return the file’s content as a string. Let’s consider an example where we have a text file named “sample.txt.”
php $fileContent = file_get_contents('sample.txt'); echo $fileContent;
In this case, the entire content of the “sample.txt” file will be read and displayed on the screen.
2.2. Handling Errors
It’s essential to consider error handling while using file_get_contents(). If the function encounters an error, it returns false. To avoid unexpected behavior, you should check the return value for errors before proceeding.
php $fileContent = file_get_contents('nonexistent.txt'); if ($fileContent === false) { echo "Error reading the file."; } else { echo $fileContent; }
By checking the return value, you can gracefully handle situations where the file is not found or inaccessible.
3. Retrieving Data from URLs
3.1. Fetching Web Pages
One of the most powerful applications of file_get_contents() is fetching data from URLs. This allows you to retrieve the content of web pages directly in your PHP script. Let’s take a look at how to fetch the content of a webpage:
php $url = 'https://www.example.com'; $pageContent = file_get_contents($url); echo $pageContent;
By executing this code, you’ll retrieve and display the HTML content of the specified URL. This feature is particularly useful when you want to scrape data from websites or create custom APIs.
3.2. Handling HTTP Headers
When fetching content from URLs, you might also need to handle HTTP headers. By default, file_get_contents() does not provide direct access to response headers. However, you can use the stream_context_create() function to create a context that allows you to retrieve these headers.
php $url = 'https://www.example.com'; $context = stream_context_create([ 'http' => [ 'method' => 'GET', 'header' => 'User-Agent: MyBot', ], ]); $pageContent = file_get_contents($url, false, $context); $headers = $http_response_header; echo $pageContent;
In this example, a custom User-Agent header is added to the request. The response headers are then available in the $http_response_header variable, allowing you to access information such as status codes and content types.
4. Processing Remote Resources
4.1. Parsing JSON Responses
Fetching JSON data from a remote API is a common scenario in modern web development. With file_get_contents(), you can easily retrieve JSON responses and parse them into usable data structures.
php $url = 'https://api.example.com/data.json'; $jsonData = file_get_contents($url); $data = json_decode($jsonData, true); print_r($data);
In this code, the JSON response from the API is fetched and decoded using json_decode(). The second argument, true, ensures that the JSON is decoded into an associative array.
4.2. Downloading Files
file_get_contents() can also be used to download files from remote sources. This is particularly useful when you want to save files to your server or manipulate them before storage.
php $fileUrl = 'https://www.example.com/images/image.jpg'; $fileContents = file_get_contents($fileUrl); $localFilePath = 'downloaded_image.jpg'; file_put_contents($localFilePath, $fileContents); echo "File downloaded and saved as $localFilePath";
In this example, the contents of an image file are fetched from a URL and then saved to the server using file_put_contents().
5. Advanced Options
5.1. Context Parameters
The third parameter of file_get_contents() allows you to provide a context for the request. This context can contain various options, such as headers, authentication details, and more. For instance, you can set a timeout for the request using the timeout option:
php $url = 'https://www.example.com'; $context = stream_context_create([ 'http' => [ 'timeout' => 10, ], ]); $pageContent = file_get_contents($url, false, $context); echo $pageContent;
5.2. Stream Contexts
Stream contexts provide a powerful way to customize the behavior of file_get_contents() even further. You can configure various aspects of the request, such as SSL options, proxies, and more. This level of customization is particularly useful when dealing with complex scenarios.
php $contextOptions = [ 'ssl' => [ 'verify_peer' => true, 'verify_peer_name' => true, ], ]; $context = stream_context_create(['http' => $contextOptions]); $url = 'https://www.example.com'; $pageContent = file_get_contents($url, false, $context); echo $pageContent;
In this example, SSL options are specified within the context to ensure secure connections.
Conclusion
The file_get_contents() function is a versatile and powerful tool in PHP that simplifies reading files, fetching web content, and processing remote resources. Its ease of use, combined with its ability to handle various scenarios, makes it a valuable asset for developers. Whether you’re reading local files, retrieving data from URLs, parsing JSON responses, or customizing requests with advanced options, file_get_contents() has the potential to streamline your development process. By exploring the examples and concepts in this blog, you can harness the full power of file_get_contents() and take your PHP applications to the next level.
Table of Contents
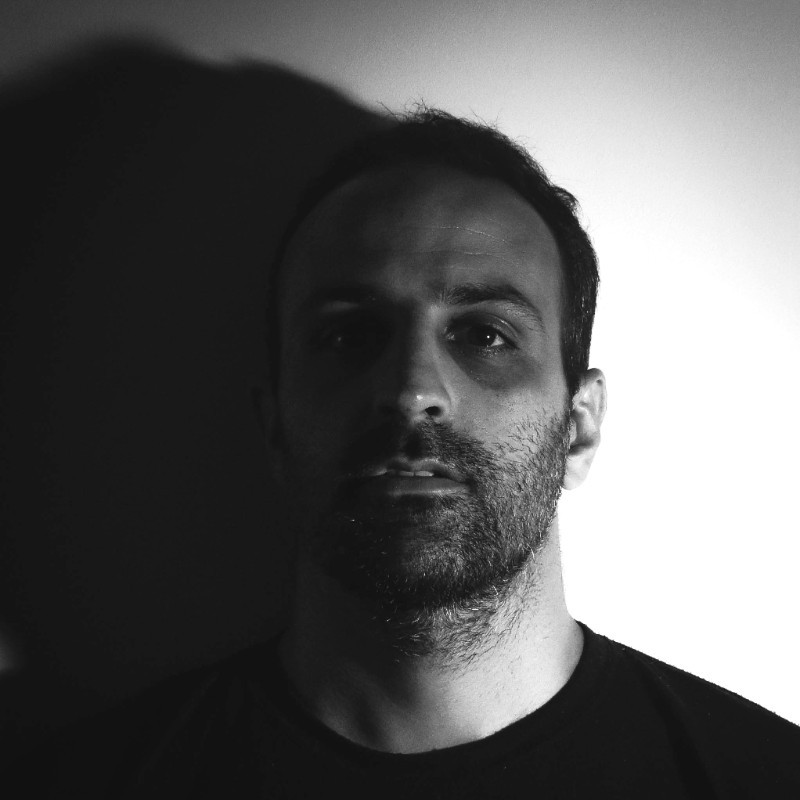
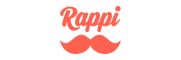