Getting Started with PHP’s filter_var() Function
In the ever-evolving landscape of web development, ensuring the security and reliability of your applications is paramount. One essential tool in the PHP developer’s arsenal is the filter_var() function. This versatile function empowers developers to validate and sanitize user input with ease, reducing the risk of vulnerabilities and enhancing overall application robustness. In this guide, we’ll take you through the fundamentals of using filter_var(), its various filtering options, and provide hands-on code samples to kickstart your journey towards more secure and dependable PHP applications.
1. Understanding the Need for Input Validation
1.1. The Importance of Data Validation
Input validation is a crucial step in building robust web applications. It involves verifying that the data provided by users adheres to the expected format and values. Failing to validate user input can lead to a host of security vulnerabilities, ranging from SQL injection and cross-site scripting (XSS) to data integrity issues.
1.2. Vulnerabilities Caused by Insufficient Validation
Consider a scenario where a user submits a form without proper validation, and the application directly incorporates that input into a database query. An attacker could exploit this vulnerability by injecting malicious SQL code, potentially gaining unauthorized access to the database. Similarly, insufficient validation of user-generated content can result in the execution of harmful scripts on other users’ browsers, compromising their security.
2. Introducing the filter_var() Function
2.1. Purpose and Benefits
filter_var() is a built-in PHP function designed to simplify input validation and data sanitization. It provides a straightforward way to validate and filter various types of input, helping developers prevent vulnerabilities and ensure that only clean, safe data enters their applications.
2.2. Supported Filter Types
filter_var() supports a wide range of filter types, each tailored to validate or sanitize specific types of data. These include:
- FILTER_VALIDATE_INT: Validates an integer.
- FILTER_VALIDATE_FLOAT: Validates a floating-point number.
- FILTER_VALIDATE_BOOLEAN: Validates a boolean value (true or false).
- FILTER_VALIDATE_EMAIL: Validates an email address.
- FILTER_VALIDATE_URL: Validates a URL.
- And many more…
3. Basic Usage of filter_var()
3.1. Validating Scalars: Strings, Integers, and Floats
Let’s start with some basic examples of using filter_var() for input validation:
php $input_string = "Hello123"; if (filter_var($input_string, FILTER_VALIDATE_INT)) { echo "Valid integer input."; } else { echo "Invalid integer input."; } $input_integer = "42"; if (filter_var($input_integer, FILTER_VALIDATE_INT)) { echo "Valid integer input."; } else { echo "Invalid integer input."; } $input_float = "3.14"; if (filter_var($input_float, FILTER_VALIDATE_FLOAT)) { echo "Valid float input."; } else { echo "Invalid float input."; }
3.2. Sanitizing Data
In addition to validation, filter_var() can be used to sanitize data, removing potentially harmful characters or tags:
php $input_html = "<script>alert('XSS attack');</script>"; $sanitized_html = filter_var($input_html, FILTER_SANITIZE_STRING); echo "Sanitized HTML: " . $sanitized_html;
4. Advanced Filtering Techniques
4.1. Filtering with Options
The filter_var() function supports additional options to fine-tune filtering behavior. For example, you can specify options for minimum and maximum values when validating integers:
php $input_age = 25; $options = array( "options" => array( "min_range" => 18, "max_range" => 60 ) ); if (filter_var($input_age, FILTER_VALIDATE_INT, $options) !== false) { echo "Valid age input."; } else { echo "Invalid age input."; }
4.2. Custom Filter Callbacks
For more complex validation scenarios, you can define custom filter callbacks:
php function custom_filter($value) { // Custom validation logic here return $valid ? $value : false; } $input_custom = "custom_value"; if (filter_var($input_custom, FILTER_CALLBACK, array("options" => "custom_filter"))) { echo "Valid input according to custom filter."; } else { echo "Invalid input according to custom filter."; }
5. Handling Common Use Cases
5.1. Validating Email Addresses
A common task is validating email addresses using the FILTER_VALIDATE_EMAIL filter:
php $input_email = "user@example.com"; if (filter_var($input_email, FILTER_VALIDATE_EMAIL)) { echo "Valid email address."; } else { echo "Invalid email address."; }
5.2. Sanitizing URLs
You can use the FILTER_SANITIZE_URL filter to sanitize URLs:
php $input_url = "https://example.com<script>"; $sanitized_url = filter_var($input_url, FILTER_SANITIZE_URL); echo "Sanitized URL: " . $sanitized_url;
6. Dealing with Filtering Failure
6.1. Handling Errors and False Positives
When filter_var() encounters invalid input, it returns false. To differentiate between an invalid input and a filtering error, use the === operator:
php $input_invalid = "invalid"; if (filter_var($input_invalid, FILTER_VALIDATE_INT) === false) { echo "Invalid input."; } else { echo "Valid input."; }
7. Best Practices for Secure Filtering
7.1. Input Validation vs. Output Escaping
While input validation is essential, it’s equally important to remember that input validation alone is not sufficient for security. Output escaping, which involves encoding data before displaying it, is crucial to prevent XSS attacks.
7.2. Validation Order
Validate input as early as possible in your application’s workflow to minimize potential vulnerabilities. Start by rejecting obviously invalid input before performing more detailed validation.
8. Real-world Examples
8.1. Registration Form Validation
Consider a user registration form. By applying filter_var(), you can validate and sanitize various fields such as usernames, passwords, and email addresses, safeguarding your application against potential attacks.
8.2. Filtering User-Generated Content
If your application allows users to submit content, using filter_var() can help ensure that the content doesn’t contain malicious scripts or harmful code that could compromise other users’ experiences.
Conclusion
In conclusion, the filter_var() function in PHP is a powerful tool that every developer should have in their toolkit. It empowers you to build more secure and reliable applications by validating and sanitizing user input effectively. By understanding the different filter types, options, and best practices, you can enhance the overall robustness of your PHP applications and contribute to a safer online environment. So go ahead, integrate filter_var() into your development process and take a proactive stance against security vulnerabilities. Your users and your application will thank you for it. Happy coding!
Remember, security is an ongoing effort, and keeping up with the latest security practices is essential. By using filter_var() and continuously improving your understanding of web security, you can stay ahead in the ever-evolving landscape of web development.
Table of Contents
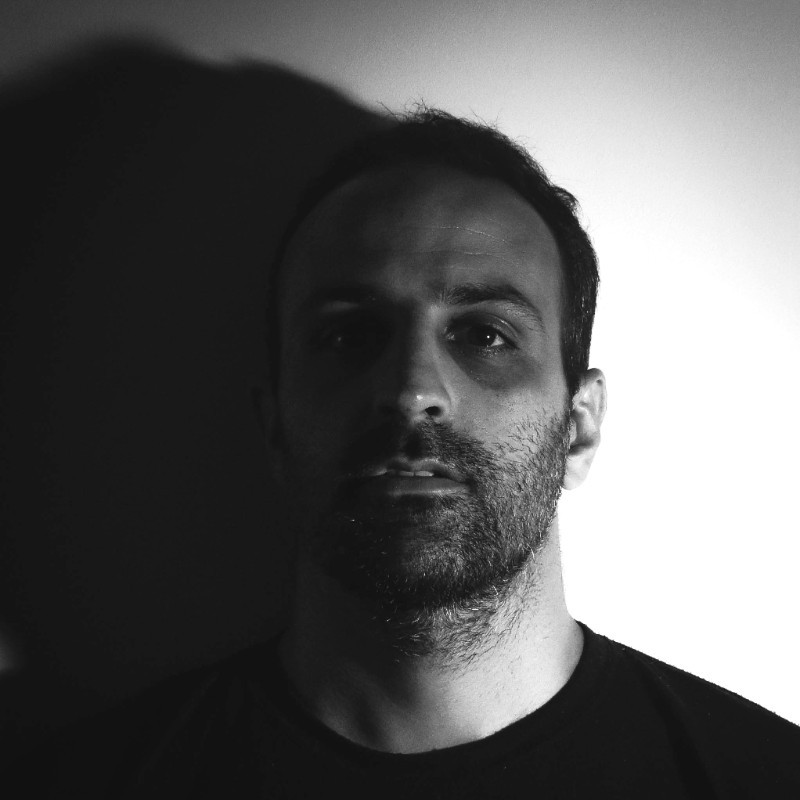
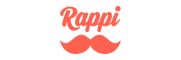