Introduction to PHP’s GD Library: Handling Images
In today’s digital age, images play a vital role in web development and content creation. From simple profile pictures to stunning graphics, images enhance the visual appeal of websites and applications. As a PHP developer, you can harness the power of the GD Library to manipulate and process images seamlessly. The GD Library, short for “Graphics Draw,” is a powerful extension in PHP that enables dynamic image creation, editing, and manipulation.
In this blog, we’ll introduce you to the PHP GD Library and explore its essential features. We’ll walk you through the basics, such as installing the GD Library, loading and saving images, and various image operations like resizing, cropping, and adding text overlays. By the end, you’ll have a solid understanding of how to leverage this powerful tool to enhance your image-handling capabilities in PHP.
1. Getting Started with GD Library
Before we dive into the exciting world of GD Library, let’s ensure that you have the extension installed and ready to use. GD Library comes bundled with most PHP installations, but it’s essential to verify its presence and enable it if necessary.
To check if GD Library is installed, create a simple PHP file (e.g., gd_check.php) with the following code:
php <?php // Check if GD Library is enabled if (extension_loaded('gd') && function_exists('gd_info')) { echo "GD Library is enabled on this server."; } else { echo "GD Library is not enabled."; }
Upload this file to your server and access it via your web browser. If GD Library is enabled, you should see the message indicating its presence.
If GD Library is not installed or enabled, you may need to configure PHP to include the GD extension. Refer to your server’s documentation for instructions on enabling GD Library.
2. Image Creation and Loading
The GD Library allows you to create images from scratch or load existing images from various formats like PNG, JPEG, GIF, and more. Let’s start by creating a simple image and saving it to the server.
2.1. Creating a New Image
To create a new image, you need to use the imagecreatetruecolor() function, which returns a new true-color image resource.
php <?php // Set image dimensions $width = 400; $height = 200; // Create a blank image $image = imagecreatetruecolor($width, $height); // Set the background color (white in this case) $bgColor = imagecolorallocate($image, 255, 255, 255); // Fill the image with the background color imagefill($image, 0, 0, $bgColor); // Save the image to a file $imageFile = 'new_image.png'; imagepng($image, $imageFile); // Free up memory imagedestroy($image); echo "Image created and saved: $imageFile";
In this code snippet, we create a blank true-color image with a width of 400 pixels and a height of 200 pixels. We set the background color to white (RGB value: 255, 255, 255) and fill the image with this color. The resulting image is then saved as “new_image.png” in the same directory as the PHP script. Finally, we release the image resource using imagedestroy() to free up memory.
2.2. Loading and Displaying an Existing Image
To load an existing image from a file and display it on a webpage, you can use the following code:
php <?php // Load the existing image $imageFile = 'example.jpg'; $image = imagecreatefromjpeg($imageFile); // Output the image directly to the browser header('Content-Type: image/jpeg'); imagejpeg($image); // Free up memory imagedestroy($image);
In this example, we load an image from the file “example.jpg” using imagecreatefromjpeg(). We then set the appropriate header to output the image directly to the browser, which will be displayed on the webpage.
3. Image Manipulation and Editing
Now that we’ve covered image creation and loading, let’s move on to the exciting part: image manipulation and editing. GD Library provides a wide range of functions to modify images according to your needs.
3.1. Resizing Images
Resizing images is a common requirement in web development. GD Library allows you to resize images while maintaining their aspect ratio.
php <?php // Load the original image $originalFile = 'original.jpg'; $originalImage = imagecreatefromjpeg($originalFile); // Get the original image dimensions $originalWidth = imagesx($originalImage); $originalHeight = imagesy($originalImage); // Set the desired width for the resized image $newWidth = 300; // Calculate the proportional height for the resized image $newHeight = ($originalHeight / $originalWidth) * $newWidth; // Create a new true-color image with the desired dimensions $resizedImage = imagecreatetruecolor($newWidth, $newHeight); // Perform the image resizing imagecopyresampled($resizedImage, $originalImage, 0, 0, 0, 0, $newWidth, $newHeight, $originalWidth, $originalHeight); // Save the resized image $resizedFile = 'resized.jpg'; imagejpeg($resizedImage, $resizedFile); // Free up memory imagedestroy($originalImage); imagedestroy($resizedImage); echo "Image resized and saved: $resizedFile";
In this code snippet, we load an original image and then calculate the proportional height for the new width of 300 pixels. The imagecreatetruecolor() function creates a new true-color image with the desired dimensions. We then use imagecopyresampled() to perform the actual resizing, and finally, the resized image is saved as “resized.jpg.”
3.2. Cropping Images
Cropping an image allows you to remove unwanted portions or focus on a specific area of the image. Here’s an example of how to crop an image using GD Library:
php <?php // Load the original image $originalFile = 'original.jpg'; $originalImage = imagecreatefromjpeg($originalFile); // Set the crop area dimensions $cropX = 50; $cropY = 50; $cropWidth = 200; $cropHeight = 150; // Create a new true-color image with the desired crop dimensions $croppedImage = imagecreatetruecolor($cropWidth, $cropHeight); // Perform the image cropping imagecopy($croppedImage, $originalImage, 0, 0, $cropX, $cropY, $cropWidth, $cropHeight); // Save the cropped image $croppedFile = 'cropped.jpg'; imagejpeg($croppedImage, $croppedFile); // Free up memory imagedestroy($originalImage); imagedestroy($croppedImage); echo "Image cropped and saved: $croppedFile";
In this code snippet, we load the original image and define the crop area’s dimensions (starting coordinates and width/height). The imagecreatetruecolor() function creates a new true-color image with the specified crop dimensions, and imagecopy() performs the actual cropping. The resulting cropped image is saved as “cropped.jpg.”
3.3. Adding Text Overlays
The GD Library enables you to add text overlays to images, which is useful for adding watermarks, captions, or dynamic content to images.
php <?php // Load the image $imageFile = 'image.jpg'; $image = imagecreatefromjpeg($imageFile); // Set the text to be added $text = 'Hello, PHP GD Library!'; // Set the font size and color $fontSize = 20; $fontColor = imagecolorallocate($image, 255, 255, 255); // White color // Set the position of the text $textX = 10; $textY = 30; // Add the text overlay to the image imagettftext($image, $fontSize, 0, $textX, $textY, $fontColor, 'arial.ttf', $text); // Save the modified image $modifiedFile = 'modified_image.jpg'; imagejpeg($image, $modifiedFile); // Free up memory imagedestroy($image); echo "Text overlay added and image saved: $modifiedFile";
In this example, we load an image and define the text to be added, its font size, and color. The imagettftext() function adds the text overlay to the image using the specified TrueType font file (in this case, “arial.ttf”). The resulting image with the text overlay is saved as “modified_image.jpg.”
Conclusion
The PHP GD Library is a powerful tool for handling and manipulating images dynamically. With the ability to create images from scratch, load existing images, and perform various image operations like resizing, cropping, and text overlays, you can elevate the visual experience of your web applications and websites.
In this blog post, we covered the basics of working with GD Library, including image creation and loading, as well as fundamental image manipulation techniques. Armed with this knowledge, you can now explore more advanced features of GD Library and unlock a world of possibilities for image processing in PHP.
Whether you’re building an image-heavy gallery, an e-commerce platform, or a social media app, the GD Library will be an indispensable ally in enhancing the visual appeal and user experience of your projects. So go ahead, experiment, and create stunning images with PHP’s GD Library! Happy coding!
Table of Contents
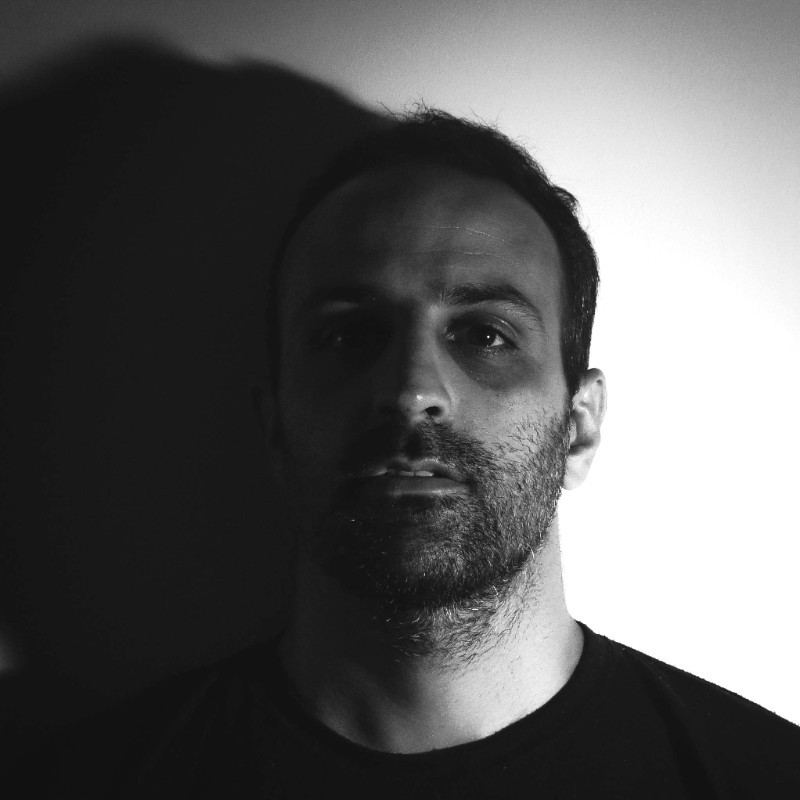
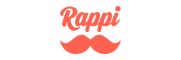