Implementing PHP’s getdate() Function in Your Projects
Working with dates and times is a common task in web development. Whether you’re building a blog, an e-commerce platform, or a scheduling application, you’ll likely need to handle dates and times. PHP, being a versatile server-side scripting language, offers a wide range of functions and libraries to manage date and time-related operations. One such function is getdate(), which allows you to retrieve detailed information about a given timestamp. In this blog post, we’ll explore how to implement PHP’s getdate() function in your projects effectively.
Table of Contents
1. Understanding the getdate() Function
Before diving into implementation, let’s gain a clear understanding of what the getdate() function does. In PHP, getdate() is a built-in function that returns an associative array containing various date and time components for the given timestamp or the current date and time if no timestamp is provided.
1.1. Syntax
The basic syntax of the getdate() function is as follows:
php getdate([int $timestamp = time()])
Here’s a breakdown of the parameters:
- $timestamp (optional): The Unix timestamp for which you want to retrieve date and time information. If omitted, the current timestamp (obtained using the time() function) is used.
1.2. Returned Array
The getdate() function returns an associative array with the following elements:
- “seconds”: Seconds (0-59)
- “minutes”: Minutes (0-59)
- “hours”: Hours (0-23)
- “mday”: Day of the month (1-31)
- “wday”: Day of the week (0-6, where 0 represents Sunday)
- “mon”: Month (1-12)
- “year”: Year (e.g., 2023)
- “yday”: Day of the year (0-365)
- “weekday”: Full textual representation of the day of the week (e.g., “Sunday”)
- “month”: Full textual representation of the month (e.g., “January”)
- “0”: Unix timestamp representing the date and time
Now that we understand the basics of the getdate() function, let’s explore how to use it in practice.
2. Implementing getdate() in Your Projects
2.1. Retrieving Current Date and Time
The simplest way to use getdate() is to retrieve the current date and time. You can do this by calling the function without any arguments:
php $dateInfo = getdate();
This code will populate the $dateInfo variable with an associative array containing the current date and time components. You can then access individual components as needed:
php $day = $dateInfo["mday"]; $month = $dateInfo["month"]; $year = $dateInfo["year"]; $hour = $dateInfo["hours"]; $minute = $dateInfo["minutes"]; $second = $dateInfo["seconds"];
2.2. Working with Specific Timestamps
If you need to retrieve date and time information for a specific timestamp, you can pass that timestamp as an argument to the getdate() function. For example, to get date and time information for a timestamp representing September 13, 2023, at 3:30 PM, you can do the following:
php $timestamp = strtotime("2023-09-13 15:30:00"); $dateInfo = getdate($timestamp);
Now, the $dateInfo array will contain the date and time components for the specified timestamp.
2.3. Formatting the Output
While the getdate() function provides a comprehensive set of date and time components, you may often need to format the output for display. For example, you might want to display the date in a more human-readable format. You can achieve this by using the various components of the $dateInfo array.
php $formattedDate = "{$dateInfo['month']} {$dateInfo['mday']}, {$dateInfo['year']}"; $formattedTime = "{$dateInfo['hours']}:{$dateInfo['minutes']}:{$dateInfo['seconds']}";
Now, you have formattedDate and formattedTime variables containing the date and time in a more readable format.
2.4. Working with Timezones
When dealing with dates and times in web applications, it’s essential to consider timezones. PHP provides the date_default_timezone_set() function to set the default timezone for your application. You can use this function in conjunction with getdate() to ensure that the displayed date and time align with your application’s timezone settings.
php date_default_timezone_set('America/New_York'); // Set the timezone to New York $dateInfo = getdate();
By setting the timezone, you can ensure that the date and time components returned by getdate() are adjusted accordingly.
3. Real-world Use Cases
Now that you know how to implement PHP’s getdate() function let’s explore some real-world use cases where it can be beneficial.
3.1. Event Scheduling
Imagine you’re building an event scheduling application. You can use getdate() to manage event dates, display countdown timers, and calculate the time remaining until an event starts.
php $eventTimestamp = strtotime("2023-10-15 19:00:00"); // Event date and time $currentTimestamp = time(); // Current date and time $eventInfo = getdate($eventTimestamp); $currentInfo = getdate($currentTimestamp); $daysRemaining = $eventInfo["yday"] - $currentInfo["yday"]; $hoursRemaining = $eventInfo["hours"] - $currentInfo["hours"]; $minutesRemaining = $eventInfo["minutes"] - $currentInfo["minutes"];
With this information, you can create a countdown timer that displays the remaining days, hours, and minutes until the event.
3.2. Blog Posts with Timestamps
If you’re developing a blog platform, you can use getdate() to display publication dates in a user-friendly format. You can also order blog posts by their publication dates.
php $posts = [ [ "title" => "Introduction to PHP", "timestamp" => strtotime("2023-08-01 10:00:00"), ], [ "title" => "Building Dynamic Websites", "timestamp" => strtotime("2023-08-10 15:30:00"), ], // Add more posts here ]; usort($posts, function ($a, $b) { return $a["timestamp"] - $b["timestamp"]; }); foreach ($posts as $post) { $postDate = getdate($post["timestamp"]); $formattedDate = "{$postDate['month']} {$postDate['mday']}, {$postDate['year']}"; echo "<h2>{$post['title']}</h2>"; echo "<p>Published on {$formattedDate}</p>"; // Display the rest of the post content }
In this example, the usort() function is used to sort the blog posts by their timestamps, ensuring that they are displayed in chronological order.
Conclusion
PHP’s getdate() function is a valuable tool for working with dates and times in your web development projects. Whether you need to retrieve current date and time information, work with specific timestamps, format date and time output, or manage timezones, getdate() provides the flexibility and functionality you need. By incorporating this function into your projects, you can create more dynamic and user-friendly applications that effectively handle date and time-related tasks. So, go ahead and implement getdate() in your PHP projects to streamline your date and time operations and enhance the overall user experience.
Table of Contents
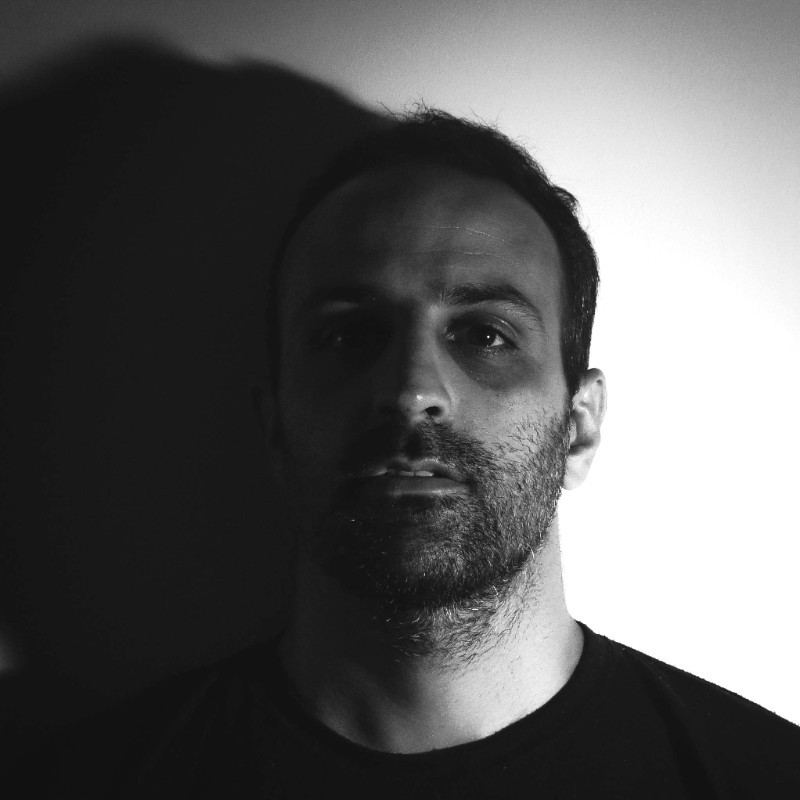
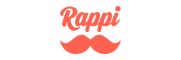