Harnessing the Power of PHP’s JSON Functions
In the world of web development, efficient data handling and manipulation are paramount. PHP, being one of the most popular server-side scripting languages, provides a robust set of functions for working with JSON data. JSON (JavaScript Object Notation) has become a standard format for data interchange due to its simplicity and ease of use. PHP’s JSON functions empower developers to seamlessly encode, decode, and manipulate JSON data, opening up a world of possibilities for creating dynamic and responsive web applications.
1. Understanding JSON: A Brief Overview
Before delving into the intricacies of PHP’s JSON functions, let’s have a quick refresher on JSON. JSON is a lightweight data interchange format that is both human-readable and machine-parseable. It is composed of key-value pairs, similar to associative arrays in PHP, and supports various data types, including strings, numbers, booleans, arrays, and even nested objects. JSON’s simplicity and compatibility with a wide range of programming languages have contributed to its widespread adoption for data exchange in web applications and APIs.
2. Encoding Data to JSON
PHP’s json_encode() function is your gateway to convert PHP data structures into JSON format. Whether you’re working with arrays, objects, or a combination of both, json_encode() takes care of the conversion process effortlessly. Let’s take a look at a basic example:
php $data = array( "name" => "John Doe", "age" => 28, "is_student" => false, "hobbies" => array("reading", "coding", "gaming") ); $json_data = json_encode($data); echo $json_data;
In this example, an associative array containing various types of data is encoded into a JSON string. The resulting JSON output would look like:
json { "name": "John Doe", "age": 28, "is_student": false, "hobbies": ["reading", "coding", "gaming"] }
3. Decoding JSON Data
Converting JSON data back into a PHP data structure is equally straightforward using the json_decode() function. This function takes a JSON string and returns an appropriate PHP data structure. Here’s an example:
php $json_data = '{ "name": "Jane Smith", "age": 32, "is_student": true, "hobbies": ["painting", "traveling"] }'; $data = json_decode($json_data, true); // The second parameter converts objects to arrays print_r($data);
By passing the JSON data to json_decode(), we retrieve the original PHP array:
php Array ( [name] => Jane Smith [age] => 32 [is_student] => 1 [hobbies] => Array ( [0] => painting [1] => traveling ) )
4. Handling JSON Errors
When working with JSON data, it’s essential to handle potential errors that might arise during encoding or decoding processes. PHP’s json_last_error() and json_last_error_msg() functions come in handy for diagnosing issues. For instance:
php $json_data = 'invalid json'; $data = json_decode($json_data); if (json_last_error() !== JSON_ERROR_NONE) { echo "JSON decoding error: " . json_last_error_msg(); }
By utilizing these functions, you can quickly identify and address any JSON-related errors that might occur in your code.
5. Manipulating JSON Data
PHP’s JSON functions extend beyond simple encoding and decoding. You can also manipulate JSON data to update, add, or remove elements within the structure. Consider the following example:
php $json_data = '{ "product": "Laptop", "price": 999.99, "specs": { "cpu": "Intel i7", "ram": "16GB" } }'; $data = json_decode($json_data, true); // Adding a new key-value pair $data["discount_percentage"] = 10; // Modifying an existing value $data["price"] = 899.99; // Removing a key along with its value unset($data["specs"]["ram"]); // Converting back to JSON $updated_json = json_encode($data, JSON_PRETTY_PRINT); echo $updated_json;
In this example, the code modifies the JSON structure by adding a discount percentage, updating the price, and removing the RAM specification. The resulting JSON output reflects these changes:
json { "product": "Laptop", "price": 899.99, "specs": { "cpu": "Intel i7" }, "discount_percentage": 10 }
6. Dealing with Nested JSON
JSON’s hierarchical nature allows for nesting objects and arrays within one another. PHP’s JSON functions seamlessly handle such complexities. Suppose you have JSON data representing a collection of books, each with multiple authors:
php $books_json = '[ { "title": "The Great Gatsby", "authors": ["F. Scott Fitzgerald"] }, { "title": "To Kill a Mockingbird", "authors": ["Harper Lee"] } ]'; $books = json_decode($books_json, true); // Adding an author to the first book $books[0]["authors"][] = "John Doe"; // Converting back to JSON $updated_books_json = json_encode($books, JSON_PRETTY_PRINT); echo $updated_books_json;
By manipulating the nested JSON structure, the code adds an additional author to the first book:
json [ { "title": "The Great Gatsby", "authors": ["F. Scott Fitzgerald", "John Doe"] }, { "title": "To Kill a Mockingbird", "authors": ["Harper Lee"] } ]
7. JSON Options for Customization
PHP’s JSON functions provide various options to tailor the encoding and decoding process to your specific needs. These options, specified as additional parameters in json_encode() and json_decode(), allow you to control indentation, handle special characters, and more. For instance:
php $data = array( "message" => "Hello, <world>!", "timestamp" => time() ); $json_options = JSON_PRETTY_PRINT | JSON_UNESCAPED_UNICODE; $json_data = json_encode($data, $json_options); echo $json_data;
Here, the JSON_PRETTY_PRINT option adds indentation for a more human-readable format, while JSON_UNESCAPED_UNICODE preserves non-ASCII characters as-is.
Conclusion
In the realm of web development, efficiently managing and manipulating data is a fundamental aspect. PHP’s JSON functions provide a powerful toolkit for encoding, decoding, and manipulating JSON data seamlessly. With these functions, you can effortlessly convert between PHP data structures and JSON, handle errors gracefully, manipulate nested JSON structures, and customize the encoding process to suit your requirements. Whether you’re building APIs, handling user input, or integrating with third-party services, harnessing the power of PHP’s JSON functions empowers you to create dynamic, and responsive web pages.
Table of Contents
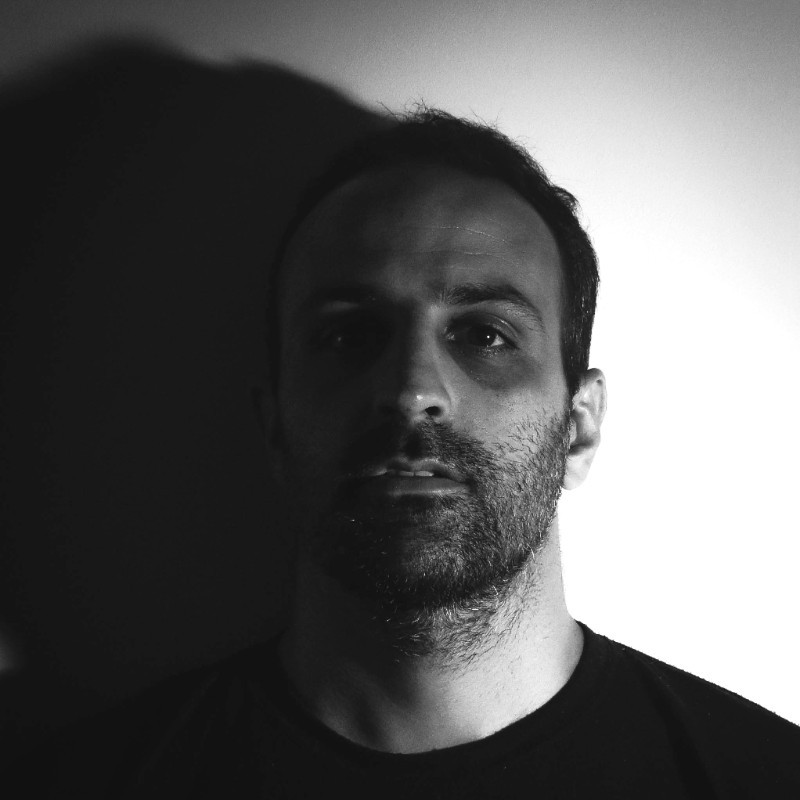
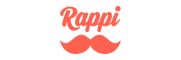