PHP’s mail() Function: Sending Emails Made Easy
In the realm of web development, effective communication is essential for user engagement and interaction. One of the primary modes of communication on the web is through emails. Whether it’s sending user notifications, password resets, newsletters, or order confirmations, emails play a pivotal role in maintaining a seamless connection between websites and users. PHP, being one of the most widely used programming languages for web development, offers a built-in function called mail() that simplifies the process of sending emails. In this blog post, we’ll delve into the world of PHP’s mail() function, exploring its features, syntax, and practical implementation through code examples.
1. Introduction to the mail() Function
The mail() function in PHP is a powerful tool that enables developers to send emails directly from their web applications. This function provides a straightforward way to incorporate email functionality without the need for external libraries or complex setups. It operates by utilizing the Simple Mail Transfer Protocol (SMTP), a standard protocol for sending emails across the internet.
2. Understanding the Syntax
Before we dive into examples, let’s take a look at the basic syntax of the mail() function:
php mail( string $to, string $subject, string $message, string $additional_headers = "", string $additional_parameters = "" ): bool
- $to: The recipient’s email address.
- $subject: The subject of the email.
- $message: The body of the email.
- $additional_headers (optional): Additional headers for the email.
- $additional_parameters (optional): Additional parameters, such as the sendmail command.
3. Exploring the Parameters
- Recipient’s Email Address ($to): This parameter specifies the email address of the recipient. You can provide a single address or a comma-separated list of addresses if you’re sending the email to multiple recipients.
- Subject ($subject): Here, you define the subject line of the email. Make sure it’s concise and relevant to the content of the email.
- Message ($message): This parameter contains the actual content of the email. You can include HTML tags for formatting or keep it plain text.
- Additional Headers ($additional_headers): If you need to include additional headers in the email, such as ‘From’ or ‘Reply-To’ addresses, you can specify them here. These headers are added to the email’s headers.
- Additional Parameters ($additional_parameters): This parameter allows you to pass additional parameters to the mail server, such as the sendmail command or specific configuration options.
4. Sending Emails with mail() – Practical Examples
Now, let’s explore a few practical examples to illustrate how to use the mail() function effectively.
Example 1: Sending a Basic Text Email
php $to = "recipient@example.com"; $subject = "Hello from PHP Mail"; $message = "This is a test email sent using PHP's mail() function."; $headers = "From: sender@example.com"; if (mail($to, $subject, $message, $headers)) { echo "Email sent successfully!"; } else { echo "Email delivery failed."; }
In this example, we’ve defined the recipient’s email address, subject, message, and a ‘From’ header. The mail() function is called with these parameters, and based on its return value, we display a success or failure message.
Example 2: Sending HTML-formatted Email
php $to = "recipient@example.com"; $subject = "HTML Email Example"; $message = "<html><body><h1>Hello from PHP Mail</h1><p>This is a <strong>test email</strong> sent using HTML formatting.</p></body></html>"; $headers = "From: sender@example.com\r\n"; $headers .= "Content-Type: text/html; charset=UTF-8\r\n"; if (mail($to, $subject, $message, $headers)) { echo "HTML email sent successfully!"; } else { echo "Email delivery failed."; }
In this example, the email’s message is formatted using HTML. We set the ‘Content-Type’ header to inform the recipient’s email client that the content is in HTML format.
Example 3: Sending Attachments
php $to = "recipient@example.com"; $subject = "Email with Attachment"; $message = "Check out the attached file!"; $headers = "From: sender@example.com"; $attachment_path = "path/to/attachment.pdf"; $attachment_content = file_get_contents($attachment_path); $attachment_encoded = base64_encode($attachment_content); $headers .= "\r\nMIME-Version: 1.0\r\n"; $headers .= "Content-Type: multipart/mixed; boundary=\"boundary\"\r\n"; $headers .= "--boundary\r\n"; $headers .= "Content-Type: application/pdf; name=\"attachment.pdf\"\r\n"; $headers .= "Content-Transfer-Encoding: base64\r\n"; $headers .= "Content-Disposition: attachment; filename=\"attachment.pdf\"\r\n\r\n"; $headers .= "$attachment_encoded\r\n"; $headers .= "--boundary--"; if (mail($to, $subject, $message, $headers)) { echo "Email with attachment sent successfully!"; } else { echo "Email delivery failed."; }
This example demonstrates how to send an email with an attachment. We’ve encoded the attachment using base64 and added the appropriate headers to define the attachment’s properties.
5. Best Practices for Using the mail() Function
- Sanitize User Input: When using user-provided input in email content or headers, ensure that you properly sanitize and validate the data to prevent security vulnerabilities like header injection.
- Avoid Sending Spam: Use the mail() function responsibly. Sending unsolicited emails or spam can lead to your emails being flagged as spam or blacklisted.
- Check Return Values: The mail() function returns a boolean value indicating whether the email was accepted for delivery by the mail server. Make sure to handle failures gracefully and provide appropriate feedback to users.
- Use Headers Wisely: Headers like ‘From’ and ‘Reply-To’ influence how recipients perceive your emails. Make sure to set them correctly to enhance trust and engagement.
Conclusion
The mail() function in PHP empowers developers to integrate email communication seamlessly into their web applications. With its simple syntax and versatile capabilities, it’s an efficient way to send emails for various purposes. From basic text emails to HTML-formatted messages and attachments, the mail() function offers a wide range of possibilities.
However, while the mail() function is useful, it’s important to remember that more complex email sending requirements might benefit from using specialized email libraries or services that offer advanced features and better deliverability.
By understanding the nuances of the mail() function and following best practices, you can harness its potential to enhance user engagement and provide effective communication within your web projects. Whether you’re sending notifications, newsletters, or crucial updates, the mail() function is your gateway to simplifying email communication in PHP.
Table of Contents
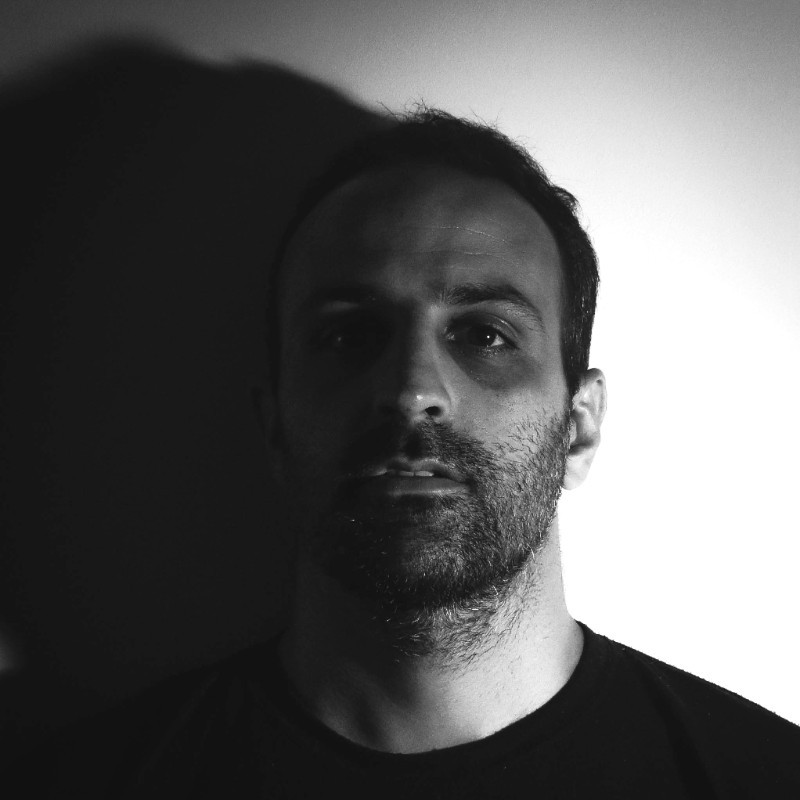
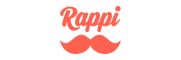