Understanding PHP’s microtime() Function: Timing Your Scripts
In the world of web development, performance optimization is paramount. Whether you’re building a simple webpage or a complex web application, ensuring that your code runs efficiently is essential. One crucial aspect of performance optimization is measuring the execution time of your PHP scripts. This is where PHP’s microtime() function comes into play. In this blog, we’ll delve into the microtime() function and explore how it can help you fine-tune your PHP applications.
Table of Contents
1. Introduction to microtime()
1.1. What Is microtime()?
microtime() is a built-in PHP function that allows you to measure the current Unix timestamp with microsecond precision. This function is particularly useful when you need to benchmark your code, identify performance bottlenecks, or simply measure the time taken for the execution of specific parts of your script.
1.2. Why Is Timing Important?
Timing your PHP scripts can provide valuable insights into their performance. By measuring how long various portions of your code take to execute, you can identify areas that may be causing slowdowns and address them effectively. This information is critical for optimizing your applications and ensuring a smooth user experience.
2. Basic Usage of microtime()
To get started with microtime(), you need to understand its basic usage. The function can be called with one or two arguments, which determine the format of the returned timestamp. Here’s how it works:
2.1. Syntax
php microtime(bool $asFloat = false): string|float
$asFloat (optional): If set to true, the function returns a floating-point number. If false (default), it returns a string with microsecond precision.
Example 1: Using microtime() without Arguments
php $start = microtime(); // Code to benchmark $end = microtime(); $elapsed = $end - $start; echo "Elapsed time: $elapsed seconds";
In this example, we record the start and end times using microtime(), calculate the elapsed time, and then display it. By default, microtime() returns a string, so we can subtract the two timestamps to get the time taken for the code to execute.
Example 2: Using microtime() with $asFloat Argument
php $start = microtime(true); // Code to benchmark $end = microtime(true); $elapsed = $end - $start; echo "Elapsed time: $elapsed seconds";
By passing true as the argument to microtime(), we receive a floating-point number representing the timestamp. This makes it easier to perform calculations like elapsed time directly.
3. Benchmarking Your Code
Now that you know how to use microtime(), let’s explore how you can use it to benchmark your PHP code effectively.
3.1. Measure the Entire Script Execution Time
Sometimes, you may want to measure the time it takes for your entire PHP script to run. This can help you get a high-level overview of your script’s performance. Here’s an example:
php $start = microtime(true); // Code for your entire script $end = microtime(true); $elapsed = $end - $start; echo "Total execution time: $elapsed seconds";
This code snippet captures the start and end times, calculates the total execution time, and displays it. You can place this code at the beginning and end of your script to measure the script’s overall performance.
3.2. Measure Specific Code Blocks
In many cases, you’ll want to focus on specific parts of your code to identify bottlenecks or areas for improvement. By measuring the execution time of individual code blocks, you can pinpoint performance issues more precisely.
php $start = microtime(true); // Code block 1 $end1 = microtime(true); // Code block 2 $end2 = microtime(true); // Code block 3 $end3 = microtime(true); $elapsed1 = $end1 - $start; $elapsed2 = $end2 - $end1; $elapsed3 = $end3 - $end2; echo "Code block 1 execution time: $elapsed1 seconds\n"; echo "Code block 2 execution time: $elapsed2 seconds\n"; echo "Code block 3 execution time: $elapsed3 seconds\n";
In this example, we measure the execution time of three different code blocks within our script. This granularity helps you identify which part of your code requires optimization.
3.3. Compare Alternative Implementations
When optimizing your code, you might have multiple ways to achieve the same result. microtime() can assist you in comparing the performance of different implementations to determine which one is more efficient.
php $start = microtime(true); // Implementation A $endA = microtime(true); // Implementation B $endB = microtime(true); $elapsedA = $endA - $start; $elapsedB = $endB - $start; if ($elapsedA < $elapsedB) { echo "Implementation A is faster by " . ($elapsedB - $elapsedA) . " seconds"; } else { echo "Implementation B is faster by " . ($elapsedA - $elapsedB) . " seconds"; }
In this scenario, we measure the execution time of two different implementations, A and B. Based on the results, we can determine which implementation performs better and choose the more efficient one.
4. Practical Applications
Let’s explore some practical applications of using microtime() to time your PHP scripts.
4.1. Database Query Performance
When working with databases, you may need to execute complex queries or perform multiple database operations in your PHP code. Timing these database-related tasks can help you optimize query performance and reduce database load.
php $start = microtime(true); // Execute a database query $queryResult = $db->query("SELECT * FROM users"); $end = microtime(true); $elapsed = $end - $start; echo "Database query execution time: $elapsed seconds";
Measuring the execution time of database queries allows you to identify slow queries that require optimization.
4.2. Page Load Time
For web applications, page load time is a critical performance metric. You can use microtime() to measure the time it takes to generate and render a web page.
php $start = microtime(true); // Generate and render the web page // ... $end = microtime(true); $elapsed = $end - $start; echo "Page load time: $elapsed seconds";
By tracking page load time, you can ensure that your web application provides a responsive and seamless user experience.
4.3. Algorithm Efficiency
When implementing algorithms or data processing tasks, it’s essential to optimize their efficiency. microtime() can help you evaluate different algorithmic approaches and choose the most efficient one.
php $start = microtime(true); // Implement algorithm A $endA = microtime(true); // Implement algorithm B $endB = microtime(true); $elapsedA = $endA - $start; $elapsedB = $endB - $start; if ($elapsedA < $elapsedB) { echo "Algorithm A is faster by " . ($elapsedB - $elapsedA) . " seconds"; } else { echo "Algorithm B is faster by " . ($elapsedA - $elapsedB) . " seconds"; }
By comparing the execution times of different algorithms, you can select the one that performs optimally for your specific use case.
5. Tips for Effective Timing
While microtime() is a valuable tool for measuring script execution time, it’s essential to use it effectively. Here are some tips to ensure accurate timing results:
5.1. Isolate Code Blocks
When measuring specific code blocks, make sure to isolate them from the rest of your script. Avoid interference from unrelated code that could affect timing accuracy.
5.2. Use Averages
For more accurate results, consider running the same code multiple times and calculating the average execution time. This helps account for variations in system load and ensures consistent measurements.
5.3. Monitor System Resources
Keep an eye on your server’s CPU and memory usage while benchmarking. High system load can impact timing results, so it’s essential to conduct tests under consistent conditions.
5.4. Profile Your Code
Consider using PHP profiling tools in conjunction with microtime() to gain deeper insights into your code’s performance. Profilers provide detailed information about function calls and resource usage.
Conclusion
PHP’s microtime() function is a powerful tool for measuring script execution time and optimizing your PHP applications. Whether you’re identifying performance bottlenecks, comparing alternative implementations, or fine-tuning database queries, microtime() can provide valuable insights into your code’s performance. By using it effectively and following best practices, you can ensure that your PHP scripts run efficiently and deliver a smooth user experience. So, start using microtime() today and take your PHP development to the next level by optimizing for performance.
Table of Contents
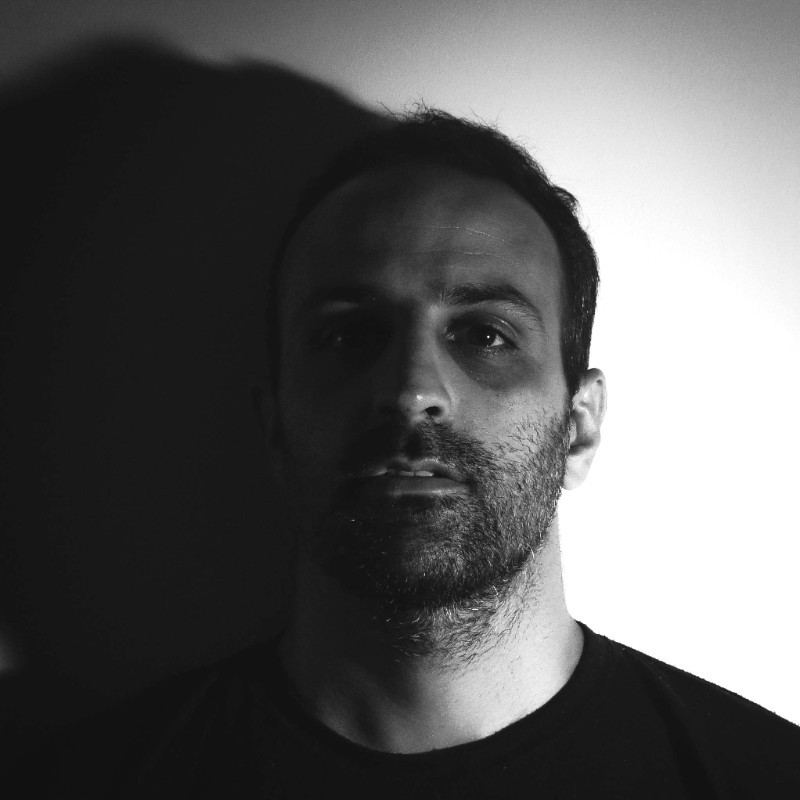
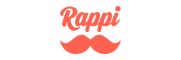