A Deep Dive into PHP’s parse_url() Function
In the realm of web development, dealing with URLs is an inevitable part of the journey. Whether you’re building a web application, creating a link management system, or simply manipulating URLs, you need a robust tool to dissect and handle them effectively. PHP’s parse_url() function is one such tool that simplifies the process of working with URLs. In this blog post, we’ll embark on a deep dive into parse_url(), dissecting its features, exploring its usage, and discovering practical applications. By the end, you’ll have a profound understanding of how to wield this function to your advantage.
Table of Contents
1. Understanding URLs
Before we delve into the intricacies of PHP’s parse_url() function, let’s briefly recap what URLs are and why they are essential in web development.
1.1. What is a URL?
URL stands for Uniform Resource Locator, and it is a string of characters used to address resources on the web. A URL typically consists of several components, including:
- Scheme: Indicates the protocol used to access the resource (e.g., http, https, ftp).
- Host: Specifies the domain or IP address where the resource is located.
- Port: If necessary, defines the port number for the connection.
- Path: Points to the specific resource or file on the server.
- Query: Contains optional parameters for the resource.
- Fragment: Refers to a specific section within the resource.
Here’s an example of a URL broken down into its components:
bash URL: https://www.example.com:8080/path/to/resource?param1=value1¶m2=value2#section2
- Scheme: https
- Host: www.example.com
- Port: 8080
- Path: /path/to/resource
- Query: param1=value1¶m2=value2
- Fragment: section2
Understanding these components is crucial for various web-related tasks, such as URL validation, building custom URL handlers, or extracting information from URLs.
2. PHP’s parse_url() Function
PHP offers a powerful function called parse_url() that simplifies the task of dissecting URLs into their constituent parts. This function takes a URL as input and returns an associative array containing the parsed components.
2.1. Basic Usage
Let’s start with the basics. Here’s how you can use parse_url():
php $url = "https://www.example.com:8080/path/to/resource?param1=value1¶m2=value2#section2"; $parsed_url = parse_url($url); print_r($parsed_url);
The output will be:
php Array ( [scheme] => https [host] => www.example.com [port] => 8080 [path] => /path/to/resource [query] => param1=value1¶m2=value2 [fragment] => section2 )
As you can see, parse_url() breaks down the URL into its components and stores them in an associative array. This array structure makes it easy to access and manipulate individual parts of the URL.
2.2. Handling Relative URLs
parse_url() excels at parsing absolute URLs, but what about relative URLs? Fortunately, it can handle those as well. When you provide a relative URL as input, the function still returns an array, but with fewer components:
php $relative_url = "/images/pic.jpg"; $parsed_relative_url = parse_url($relative_url); print_r($parsed_relative_url);
The output for a relative URL will look like this:
php Array ( [path] => /images/pic.jpg )
In this case, parse_url() extracts the path component, which is the only part available in a relative URL. This can be helpful when working with links or resources within your own website.
3. Exploring parse_url() Components
Now that we’ve covered the basics of using parse_url(), let’s take a closer look at each component it can extract from a URL.
3.1. Scheme
The scheme component represents the protocol used to access the resource. It’s often used to determine whether the URL points to a secure (https) or non-secure (http) resource. Let’s see how to access the scheme component:
php $url = "https://www.example.com"; $parsed_url = parse_url($url); $scheme = $parsed_url['scheme']; echo "Scheme: $scheme";
In this example, $scheme will contain the string “https.”
3.2. Host
The host component specifies the domain or IP address where the resource is located. It’s crucial for identifying the server that hosts the resource. Here’s how to access the host component:
php $url = "https://www.example.com"; $parsed_url = parse_url($url); $host = $parsed_url['host']; echo "Host: $host";
The output will be “Host: www.example.com.”
3.3. Port
Sometimes, a URL may specify a port number for the connection. The port component, if present, contains this information. Here’s how to access the port component:
php $url = "https://www.example.com:8080"; $parsed_url = parse_url($url); $port = $parsed_url['port']; echo "Port: $port";
If the URL does not include a port, the $port variable will be empty.
3.4. Path
The path component points to the specific resource or file on the server. It indicates the hierarchical location of the resource within the server’s directory structure. To access the path component, use the following code:
php $url = "https://www.example.com/path/to/resource"; $parsed_url = parse_url($url); $path = $parsed_url['path']; echo "Path: $path";
The output will be “Path: /path/to/resource.”
3.5. Query
The query component contains optional parameters for the resource. These parameters are typically used in GET requests to pass data to the server. To access the query component, use the following code:
php $url = "https://www.example.com/resource?param1=value1¶m2=value2"; $parsed_url = parse_url($url); $query = $parsed_url['query']; echo "Query: $query";
The output will be “Query: param1=value1¶m2=value2.”
3.6. Fragment
The fragment component, also known as the “hash,” refers to a specific section within the resource. It is often used in conjunction with anchor links to navigate to a specific part of a webpage. To access the fragment component, use the following code:
php $url = "https://www.example.com/page#section2"; $parsed_url = parse_url($url); $fragment = $parsed_url['fragment']; echo "Fragment: $fragment";
The output will be “Fragment: section2.”
4. Practical Applications of parse_url()
Now that we have a firm grasp of how parse_url() works and how to access its components, let’s explore some practical applications of this versatile function.
4.1. URL Validation
One common use case for parse_url() is URL validation. When users input URLs, it’s essential to ensure that the URLs are well-formed and safe to use. By using parse_url(), you can quickly verify whether the provided URL has the required components (e.g., scheme and host) and is not a relative URL.
Here’s an example of how to perform basic URL validation using parse_url():
php function validateUrl($url) { $parsed_url = parse_url($url); if (isset($parsed_url['scheme']) && isset($parsed_url['host'])) { return true; // Valid URL } else { return false; // Invalid URL } } $url1 = "https://www.example.com"; $url2 = "/images/pic.jpg"; if (validateUrl($url1)) { echo "URL 1 is valid.<br>"; } else { echo "URL 1 is invalid.<br>"; } if (validateUrl($url2)) { echo "URL 2 is valid."; } else { echo "URL 2 is invalid."; }
In this example, validateUrl() checks whether a URL contains both the scheme and host components. URL 1 is valid, while URL 2 is invalid since it’s a relative URL.
4.2. Building Custom URL Handlers
In some cases, you may need to build custom URL handlers for your web application. For instance, you might want to create a URL routing system that maps URLs to specific controller actions. parse_url() can help you extract relevant information from the URL to make routing decisions.
Here’s a simplified example of how you can use parse_url() in a URL routing system:
php $request_url = $_SERVER['REQUEST_URI']; $parsed_url = parse_url($request_url); $path = $parsed_url['path']; // Define routes and corresponding controller actions $routes = [ '/home' => 'HomeController@index', '/about' => 'AboutController@index', '/contact' => 'ContactController@index', ]; if (isset($routes[$path])) { $controller_action = $routes[$path]; list($controller, $action) = explode('@', $controller_action); // Call the controller action $controllerInstance = new $controller(); $controllerInstance->$action(); } else { // Handle 404 Not Found http_response_code(404); echo "404 Not Found"; }
In this example, parse_url() helps extract the path component from the request URL, allowing you to route the request to the appropriate controller action.
4.3. Modifying URLs
parse_url() also comes in handy when you need to modify specific components of a URL. For instance, you might want to change the query parameters of a URL or update the scheme to ensure secure connections. With parse_url(), you can easily manipulate these components and reconstruct the modified URL.
Here’s an example of modifying a URL’s query parameters using parse_url():
php $url = "https://www.example.com/resource?param1=value1¶m2=value2"; $parsed_url = parse_url($url); // Modify the query parameters $parsed_url['query'] = "param3=newValue3"; // Reconstruct the modified URL $modified_url = $parsed_url['scheme'] . '://' . $parsed_url['host'] . $parsed_url['path'] . '?' . $parsed_url['query']; echo "Modified URL: $modified_url";
In this example, we change the value of param2 to newValue3 in the query parameters and reconstruct the URL.
Conclusion
In this deep dive into PHP’s parse_url() function, we’ve uncovered its capabilities and explored various practical applications. Whether you’re validating URLs, building custom URL handlers, or modifying URLs on the fly, parse_url() is a valuable tool in your web development arsenal. Its ability to break down URLs into their components simplifies complex tasks, making it an indispensable function for web developers. As you continue your web development journey, remember that understanding and harnessing the power of parse_url() can significantly enhance your ability to work with URLs effectively.
Table of Contents
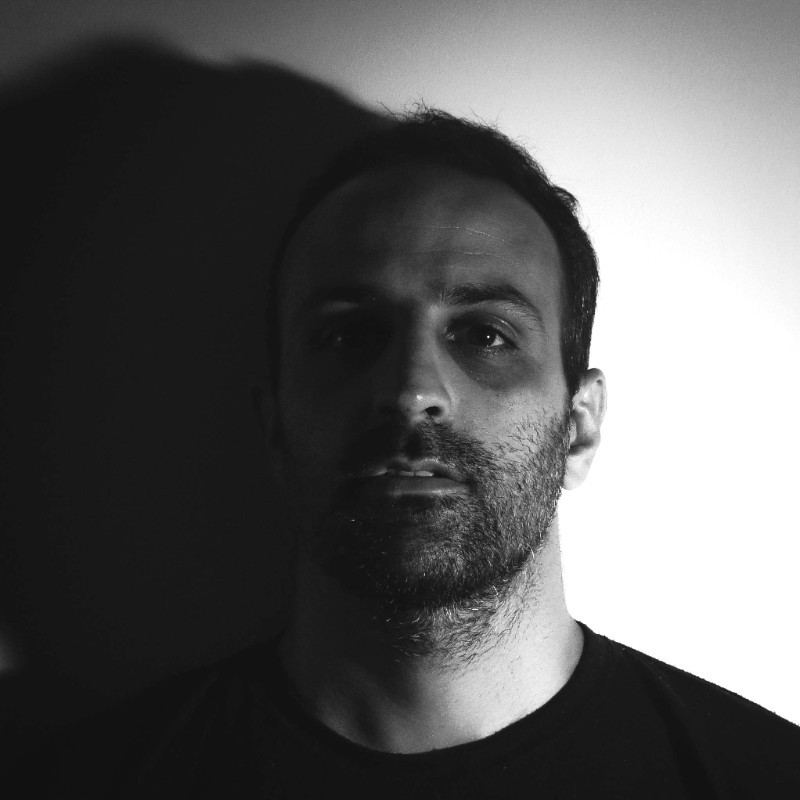
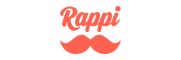