Getting Started with PHP’s preg_split() Function
When it comes to manipulating and parsing strings in PHP, the preg_split() function is a powerful tool that allows you to split strings using regular expressions. Whether you need to break down a complex string into smaller components or extract specific information from it, preg_split() can help you achieve your goal. In this blog post, we’ll dive into the world of preg_split(), exploring its syntax, usage, and providing practical examples along the way.
Table of Contents
1. What is preg_split()?
preg_split() is a PHP function that performs a regular expression-based split on a given string. It takes two main parameters: the regular expression pattern to match and the input string to split. The function then returns an array of substrings created by splitting the input string at the points where the regular expression pattern matches.
1.1. Basic Syntax
Here’s the basic syntax of the preg_split() function:
php preg_split($pattern, $subject);
- $pattern: This is the regular expression pattern you want to match.
- $subject: This is the input string that you want to split based on the pattern.
2. Splitting a String by Space
Let’s start with a simple example. Suppose you have a string containing words separated by spaces, and you want to split it into an array of individual words. You can achieve this using preg_split() and a regular expression pattern that matches spaces.
php $string = "Hello World PHP"; $words = preg_split('/\s+/', $string); print_r($words);
In this example, we use the regular expression pattern /\s+/ to match one or more whitespace characters (spaces or tabs). The preg_split() function then splits the $string variable wherever this pattern is found, resulting in the following output:
csharp Array ( [0] => Hello [1] => World [2] => PHP )
As you can see, the input string has been successfully split into an array of individual words.
3. Using Flags
preg_split() also allows you to specify flags that modify its behavior. One commonly used flag is the PREG_SPLIT_NO_EMPTY flag, which excludes empty elements from the resulting array.
Let’s modify our previous example to exclude empty elements:
php $string = "Hello World PHP"; $words = preg_split('/\s+/', $string, -1, PREG_SPLIT_NO_EMPTY); print_r($words);
In this updated code, we’ve added the PREG_SPLIT_NO_EMPTY flag as the fourth parameter of preg_split(). As a result, any consecutive spaces in the input string are treated as a single delimiter, and empty elements are removed from the resulting array:
csharp Array ( [0] => Hello [1] => World [2] => PHP )
4. Splitting by Punctuation
You can use preg_split() to split strings based on more complex patterns, such as punctuation marks. Let’s say you have a string containing a sentence, and you want to split it into an array of words while preserving punctuation marks. Here’s how you can do it:
php $string = "Hello, my name is John. I enjoy programming!"; $words = preg_split('/(\w+|\p{P})/', $string, -1, PREG_SPLIT_NO_EMPTY | PREG_SPLIT_DELIM_CAPTURE); print_r($words);
In this example, we use the regular expression pattern ‘/(\w+|\p{P})/’ to match either one or more word characters (\w+) or a Unicode punctuation character (\p{P}). The PREG_SPLIT_DELIM_CAPTURE flag is used to capture the delimiters (punctuation marks) as well. This results in the following output:
csharp Array ( [0] => Hello [1] => , [2] => my [3] => name [4] => is [5] => John [6] => . [7] => I [8] => enjoy [9] => programming [10] => ! )
As you can see, the string has been successfully split into an array of words and punctuation marks.
5. Limiting the Number of Splits
By default, preg_split() splits the input string as many times as possible based on the pattern. However, you can limit the number of splits by specifying a third parameter, which represents the maximum number of splits to perform.
php $string = "apple,banana,cherry,date"; $fruits = preg_split('/,/', $string, 2); print_r($fruits);
In this example, we want to split the string $string using a comma as the delimiter, but we specify 2 as the third parameter. As a result, only the first two occurrences of the delimiter are used for splitting, and the rest of the string remains intact:
csharp Array ( [0] => apple [1] => banana,cherry,date )
6. Handling Complex Patterns
Sometimes, you may need to split strings based on more complex patterns that involve special characters. In such cases, you can use the preg_quote() function to escape any potentially problematic characters in your pattern.
Here’s an example where we want to split a string based on the . character, which is a special character in regular expressions. We’ll use preg_quote() to safely escape it:
php $string = "example.com is a website. google.com is a search engine."; $domains = preg_split('/' . preg_quote('.', '/') . '/', $string); print_r($domains);
In this code, we use preg_quote(‘.’, ‘/’) to escape the . character, ensuring that it is treated as a literal character in the regular expression pattern. The resulting output is as follows:
csharp Array ( [0] => example [1] => com is a website [2] => google [3] => com is a search engine )
Conclusion
PHP’s preg_split() function is a versatile tool for splitting strings using regular expressions. Whether you need to break down strings into words, extract information, or handle more complex splitting requirements, preg_split() can help you accomplish your tasks effectively. By understanding its syntax, flags, and practical usage, you can harness the power of regular expressions to manipulate strings in PHP with ease.
Table of Contents
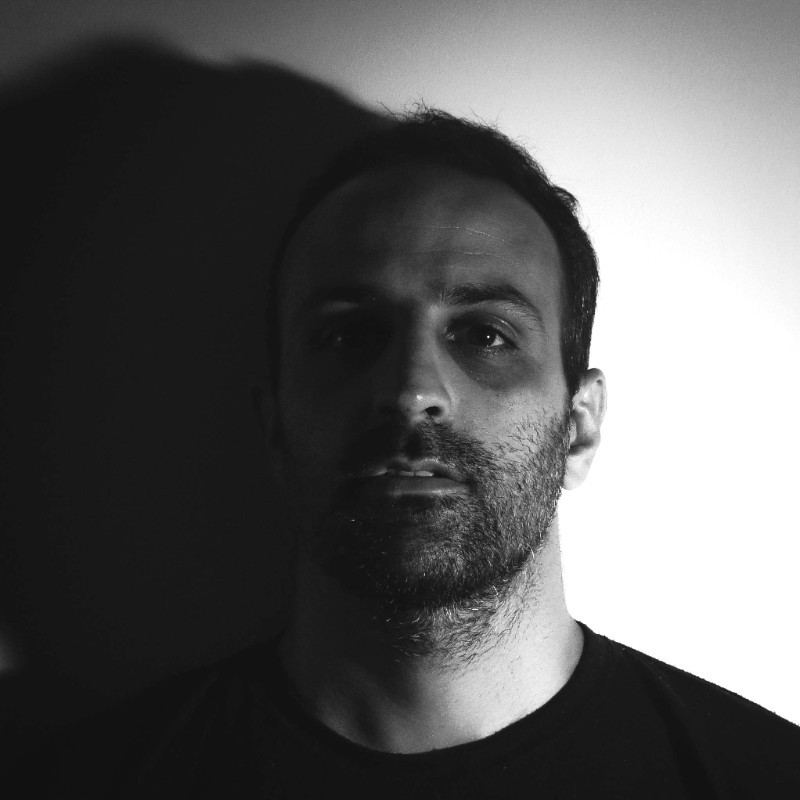
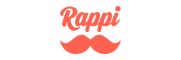