Developing Secure Web Applications with PHP
In today’s digital landscape, security is of paramount importance when it comes to web application development. Cyberattacks, data breaches, and other security incidents have become all too common, leaving both businesses and users vulnerable to exploitation. As a PHP developer, it is essential to understand the potential risks and adopt best practices to develop secure web applications. In this blog, we will explore various security considerations, techniques, and code samples to help you build robust and secure PHP web applications.
1. Understanding the Web Application Security Landscape
Before diving into the specifics of PHP application security, it’s crucial to grasp the broader security landscape. Threats to web applications can range from simple attacks like cross-site scripting (XSS) and SQL injection to more sophisticated ones, such as cross-site request forgery (CSRF) and remote code execution. Developers must be aware of these risks to effectively protect their applications and users.
2. Common Web Application Vulnerabilities
Cross-Site Scripting (XSS): Attackers inject malicious scripts into web pages, enabling them to steal sensitive information, hijack user sessions, or deface the website.
- SQL Injection: Attackers exploit poorly sanitized input to execute unauthorized SQL queries, potentially gaining unauthorized access to the database.
- Cross-Site Request Forgery (CSRF): Attackers trick users into performing unintended actions on a website where they are authenticated, leading to unauthorized operations.
- Remote Code Execution (RCE): Vulnerabilities that allow attackers to execute malicious code on the web server, compromising the entire system.
- Session Hijacking and Fixation: Attackers steal or manipulate session identifiers to impersonate legitimate users.
- Security Misconfigurations: Improperly configured servers, databases, or applications that inadvertently expose sensitive information.
3. Best Practices for Developing Secure PHP Web Applications
To build secure web applications with PHP, it’s essential to follow a set of best practices and guidelines:
3.1. Input Validation and Sanitization
Always validate and sanitize user input to prevent common attacks like XSS and SQL injection. Use PHP filtering functions, regex, and input validation libraries to ensure that data is clean and safe to use.
php // Input validation example $username = $_POST['username']; if (!preg_match('/^[a-zA-Z0-9]+$/', $username)) { // Handle invalid username }
3.2. Parameterized Queries
Use parameterized queries (prepared statements) instead of direct SQL queries to avoid SQL injection vulnerabilities.
php // Prepared statement example $stmt = $pdo->prepare('SELECT * FROM users WHERE username = :username'); $stmt->bindParam(':username', $username); $stmt->execute();
3.3. Cross-Site Scripting (XSS) Prevention
Escape output using PHP functions like htmlspecialchars to prevent XSS attacks by rendering malicious scripts harmless.
php // Output escaping example echo htmlspecialchars($userInput, ENT_QUOTES, 'UTF-8');
3.4. Cross-Site Request Forgery (CSRF) Protection
Implement CSRF tokens to verify the authenticity of requests and protect against CSRF attacks.
php // CSRF token generation and validation example $token = bin2hex(random_bytes(32)); $_SESSION['csrf_token'] = $token; // In the form <input type="hidden" name="csrf_token" value="<?php echo $token; ?>"> // In the processing script if (!hash_equals($_SESSION['csrf_token'], $_POST['csrf_token'])) { // Handle CSRF attack }
3.5. Password Security
Store passwords securely using modern hashing algorithms like bcrypt. Never store plain text passwords in the database.
php // Password hashing example $hashedPassword = password_hash($password, PASSWORD_BCRYPT);
3.6. Limit Privileges and Use Least Privilege Principle
Ensure that your web application’s database user has the minimum required privileges. Avoid using the root user or granting unnecessary permissions.
3.7. Error Handling and Logging
Implement proper error handling and logging mechanisms to catch and address potential issues without exposing sensitive information to attackers.
php // Error logging example error_log('Error message', 3, '/path/to/error_log');
3.8. HTTPS and SSL/TLS
Always use HTTPS and SSL/TLS to encrypt data transmitted between the client and server, ensuring data integrity and confidentiality.
3.9. Regularly Update Libraries and Components
Keep all PHP libraries, frameworks, and components up-to-date to avoid known vulnerabilities.
4. Secure Session Management
Proper session management is critical for web application security. Here are some best practices to ensure secure session handling:
Use secure cookies with the “httponly” and “secure” flags to prevent XSS attacks and ensure cookies are transmitted only over encrypted connections.
php // Setting secure cookies example setcookie('session_cookie', $value, time() + 3600, '/', 'example.com', true, true);
Set session timeout to automatically log out inactive users and prevent session fixation attacks.
php // Set session timeout example ini_set('session.gc_maxlifetime', 3600); session_set_cookie_params(3600); session_start();
Regenerate session IDs after successful login to protect against session fixation.
php // Regenerate session ID example session_regenerate_id(true);
5. Data Encryption and Hashing
Encrypt sensitive data such as user passwords, credit card information, and other private data before storing it in the database.
php // Data encryption example using OpenSSL function encryptData($data, $key) { $iv = random_bytes(16); $encrypted = openssl_encrypt($data, 'AES-256-CBC', $key, 0, $iv); return base64_encode($iv . $encrypted); }
Additionally, use secure hashing algorithms like bcrypt to hash passwords.
php // Password hashing example using bcrypt $hashedPassword = password_hash($password, PASSWORD_BCRYPT);
6. Web Application Firewall (WAF)
Implement a Web Application Firewall (WAF) to filter and monitor HTTP requests, blocking malicious traffic and attacks.
7. Regular Security Audits and Penetration Testing
Perform regular security audits and penetration testing to identify vulnerabilities and weaknesses in your web application. Fix any issues promptly to maintain a secure environment.
Conclusion
Developing secure web applications with PHP is not an option; it’s an obligation. By understanding the common threats and adhering to best practices, you can fortify your applications against potential cyberattacks. Remember to validate and sanitize user input, utilize parameterized queries, prevent XSS and CSRF attacks, and enforce secure session management. Additionally, encrypt sensitive data, use secure hashing for passwords, and stay vigilant with regular security audits and updates. By implementing these measures and staying informed about emerging threats, you can confidently develop robust and secure PHP web applications, safeguarding your users and their data from harm. Stay secure, and happy coding!
Table of Contents
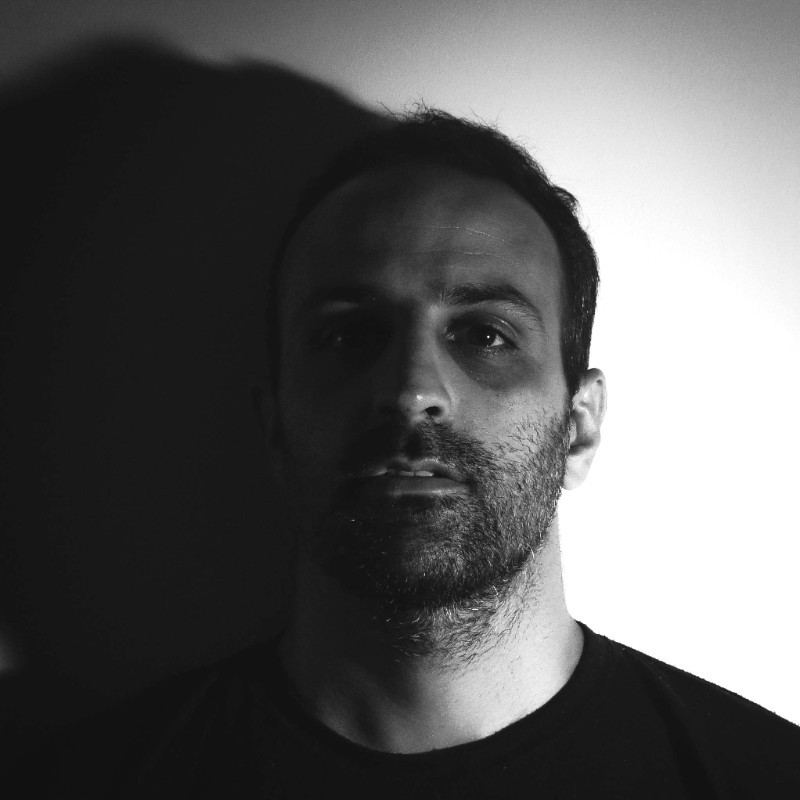
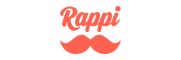