The Art of PHP’s serialize() Function
In the realm of web development, PHP has stood the test of time as a versatile and powerful scripting language. One of its lesser-known but incredibly useful functions is serialize(). This function plays a crucial role in data storage and transfer within PHP applications. In this article, we will delve into the art of using the serialize() function effectively, exploring its features, use cases, and potential pitfalls.
Table of Contents
1. Understanding Serialization
1.1. What is Serialization?
Serialization refers to the process of converting complex data structures, such as arrays and objects, into a format that can be easily stored or transmitted. This format is often a string that contains all the necessary information to reconstruct the original data structure. In PHP, the serialize() function accomplishes this task seamlessly.
1.2. Why Use Serialization in PHP?
Serialization serves as a bridge between the structured data within your PHP application and the need to store or transmit this data. It allows you to retain the integrity of the data while making it compatible with storage mechanisms like databases or transfer protocols like HTTP.
2. The serialize() Function
2.1. Syntax and Usage
The serialize() function in PHP has a simple syntax:
php string serialize(mixed $value);
Here, $value can be any variable or data structure that you want to serialize. It could be an array, an object, or a scalar value.
To use the serialize() function, you simply pass the data to be serialized as an argument, and it returns a string representation of the serialized data.
2.2. Serialized Data Structure
The serialized data produced by the serialize() function is a compact string that encapsulates the original data structure. It includes type information and data values. For instance, a serialized array might look like this:
css A:3:{i:0;s:5:"apple";i:1;s:6:"banana";i:2;s:5:"orange";}
Here, a:3 indicates an array with three elements, i:0, i:1, and i:2 are keys, and s:5 and s:6 represent string lengths followed by the string value.
3. Serialization of Basic Data Types
3.1. Strings
Strings are a fundamental data type in PHP, and serializing them is straightforward. For example:
php $data = "Hello, Serialize!"; $serialized_data = serialize($data);
3.2. Integers and Floats
Numeric values can also be serialized without any issues:
php $integer = 42; $float = 3.14; $serialized_integer = serialize($integer); $serialized_float = serialize($float);
3.3. Booleans
Booleans, representing true or false, can be serialized as well:
php $is_active = true; $serialized_boolean = serialize($is_active);
3.4. Null Values
Even null values can be serialized:
php $empty_value = null; $serialized_null = serialize($empty_value);
4. Serializing Complex Data Structures
4.1. Arrays
Arrays are a staple of PHP programming, and serializing them retains their structure:
php $array_data = [1, 2, 3, 4, 5]; $serialized_array = serialize($array_data);
4.2. Associative Arrays
Associative arrays, where keys are strings, can be serialized just like indexed arrays:
php $assoc_array = ['name' => 'John', 'age' => 30, 'city' => 'New York']; $serialized_assoc_array = serialize($assoc_array);
4.3. Objects
Objects can also be serialized, provided their class implements the Serializable interface:
php class Person implements Serializable { private $name; public function __construct($name) { $this->name = $name; } public function serialize() { return serialize($this->name); } public function unserialize($data) { $this->name = unserialize($data); } } $person = new Person("Alice"); $serialized_person = serialize($person);
5. Storing Serialized Data
5.1. Database Storage
Serialized data can be stored in databases as strings. However, be cautious about searching within serialized data, as it can be complex and inefficient.
5.2. Caching
Caching systems often use serialization to store and retrieve complex data structures quickly. This is particularly useful for minimizing database queries.
6. Deserialization with unserialize()
6.1. Reconstructing Serialized Data
To reverse the serialization process, PHP provides the unserialize() function:
php $original_data = unserialize($serialized_data);
6.2. Handling Data Discrepancies
When unserializing, ensure that the classes and structures used in serialization are available. Changes to class definitions can lead to errors during deserialization.
7. Use Cases of Serialization
7.1. Session Management
Serialized data is commonly used to manage user sessions, storing and retrieving session information efficiently.
7.2. Data Transfer
Serialization is vital when transmitting data between different systems or components, making it easy to send structured data over HTTP or other protocols.
7.3. Configuration Storage
Storing configuration settings in a serialized format can simplify the process of reading and writing configuration files.
8. Potential Security Concerns
8.1. Code Injection
Deserializing untrusted data can lead to code injection attacks. Validate and sanitize serialized data before deserialization.
8.2. Secure Usage of unserialize()
Avoid using unserialize() on data from untrusted sources. Implement proper input validation and use alternatives like JSON when appropriate.
9. Best Practices
9.1. Data Validation
Validate serialized data before deserializing to prevent security vulnerabilities and data corruption.
9.2. Versioning
Consider versioning serialized data to accommodate changes in data structure over time.
9.3. Alternatives to Serialization
For certain use cases, consider alternatives like JSON or XML for data interchange, as they offer better human-readability and are less prone to security risks.
Conclusion
In conclusion, PHP’s serialize() function is a powerful tool in a developer’s arsenal. It enables efficient data storage, transfer, and manipulation, but it should be used with caution, especially when dealing with untrusted data. By understanding its nuances and adhering to best practices, you can harness the true potential of serialization while keeping your PHP applications secure and efficient.
Table of Contents
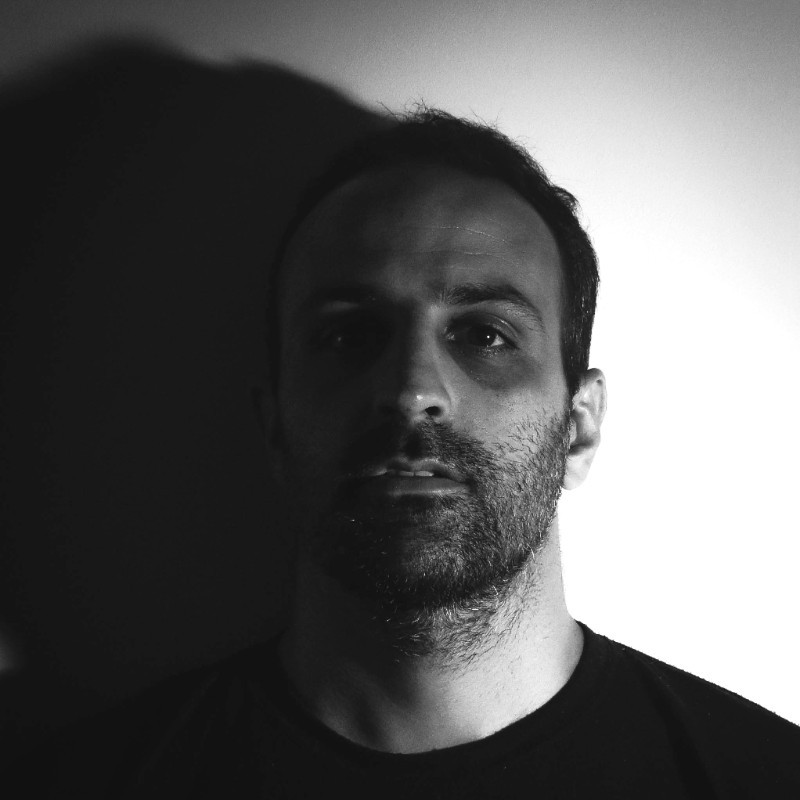
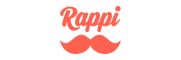