Server-side Scripting with PHP: An Overview
In the realm of web development, the dynamic nature of modern websites and applications has necessitated powerful tools and technologies that enable the creation of interactive and responsive user experiences. Server-side scripting plays a pivotal role in achieving this by allowing developers to process data, manage databases, and generate dynamic content on the server before delivering it to the client’s browser. Among the various scripting languages, PHP stands out as a versatile and widely-used option. In this article, we will delve into the world of server-side scripting with PHP, exploring its fundamental concepts, benefits, and how to get started.
1. Understanding Server-side Scripting
At its core, server-side scripting involves executing scripts on a web server to generate dynamic content that is then sent to the client’s browser. This approach contrasts with client-side scripting, where scripts run directly in the browser and are responsible for enhancing the user interface and experience. Server-side scripting is particularly useful for handling tasks that involve data manipulation, database interactions, and server-level operations.
2. The Role of PHP
PHP, which stands for Hypertext Preprocessor, is a popular server-side scripting language designed for web development. It was created to simplify the process of generating dynamic web pages and applications. PHP scripts are executed on the server before the content is sent to the client’s browser, enabling the inclusion of dynamic data and logic within web pages.
3. Benefits of Server-side Scripting with PHP
Utilizing PHP for server-side scripting offers several benefits that contribute to the efficiency and effectiveness of web development projects:
- Dynamic Content Generation: PHP allows developers to create web pages with content that can change based on various factors, such as user input or database information. This dynamic nature enhances user experiences and facilitates personalized content delivery.
- Database Interaction: PHP provides robust support for interacting with databases, enabling developers to fetch, store, and manipulate data. This is essential for creating applications that rely on user accounts, e-commerce functionalities, and content management systems.
- Code Reusability: PHP supports modular programming through functions and classes, allowing developers to create reusable code components. This reduces redundancy and simplifies maintenance, as changes made to one module can propagate throughout the application.
- Security: Server-side scripting helps maintain security by keeping sensitive operations and data on the server, away from the client’s browser. PHP offers various security features and functions to protect against common vulnerabilities.
4. Getting Started with PHP Server-side Scripting
To begin working with PHP for server-side scripting, follow these steps:
4.1. Setting Up the Environment:
Ensure you have a web server (e.g., Apache), PHP interpreter, and a database system (e.g., MySQL) installed on your machine. Alternatively, you can use pre-packaged solutions like XAMPP or WAMP that provide a complete environment.
4.2. Writing Your First PHP Script:
Create a new file with a .php extension in your web server’s document root directory. You can use a plain text editor or an integrated development environment (IDE) for this purpose. Let’s start with a simple “Hello, PHP!” example:
php <!DOCTYPE html> <html> <head> <title>My First PHP Script</title> </head> <body> <?php echo "Hello, PHP!"; ?> </body> </html>
Save the file and access it through your web browser using the appropriate URL (e.g., http://localhost/yourfile.php). You should see the “Hello, PHP!” message displayed on the page.
4.3. Embedding PHP in HTML:
PHP code can be embedded within HTML using the <?php ?> tags. This allows you to mix dynamic content generation with static HTML elements. For example:
php <!DOCTYPE html> <html> <head> <title>Dynamic Content Example</title> </head> <body> <h1>Welcome, <?php echo "User"; ?>!</h1> <p>Today is <?php echo date("Y-m-d"); ?>.</p> </body> </html>
In this example, the PHP code inside the <?php ?> tags is executed on the server and the results are embedded in the HTML output.
5. Advanced PHP Concepts for Server-side Scripting
As you become more comfortable with the basics of PHP server-side scripting, you can explore advanced concepts that enhance your development capabilities:
5.1. Working with Forms:
PHP is commonly used to process form submissions. When a user submits a form, the data is sent to the server, where PHP scripts can validate, sanitize, and process the information. Here’s a simplified example:
php <!DOCTYPE html> <html> <head> <title>Contact Form</title> </head> <body> <form method="post" action="process.php"> <input type="text" name="name" placeholder="Your Name"> <input type="email" name="email" placeholder="Your Email"> <textarea name="message" placeholder="Your Message"></textarea> <button type="submit">Submit</button> </form> </body> </html>
In this example, the form data is submitted to a PHP script named process.php for handling.
5.2. Database Connectivity:
PHP’s database support enables interaction with databases to retrieve, insert, update, and delete data. To connect to a MySQL database, use functions like mysqli_connect() and mysqli_query(). Here’s a basic example:
php <?php $connection = mysqli_connect("localhost", "username", "password", "database_name"); if (!$connection) { die("Database connection failed: " . mysqli_connect_error()); } $query = "SELECT * FROM users"; $result = mysqli_query($connection, $query); while ($row = mysqli_fetch_assoc($result)) { echo "Username: " . $row["username"] . "<br>"; } mysqli_close($connection); ?>
5.3. Object-Oriented Programming (OOP):
PHP supports object-oriented programming, allowing you to create classes and objects. OOP enhances code organization and reusability. Here’s a simple class example:
php <?php class Car { public $brand; public $model; function __construct($brand, $model) { $this->brand = $brand; $this->model = $model; } function displayInfo() { echo "Car: {$this->brand} {$this->model}"; } } $myCar = new Car("Toyota", "Camry"); $myCar->displayInfo(); ?>
Conclusion
Server-side scripting with PHP empowers developers to create dynamic, interactive, and data-driven web applications. By leveraging PHP’s capabilities, you can process user input, manage databases, and generate personalized content. Whether you’re building a simple contact form or a complex e-commerce platform, PHP’s flexibility and extensive community support make it a valuable tool in the web development arsenal. As you continue your journey in server-side scripting, remember that practice, experimentation, and a commitment to learning are key to mastering the art of creating robust and efficient web applications.
Table of Contents
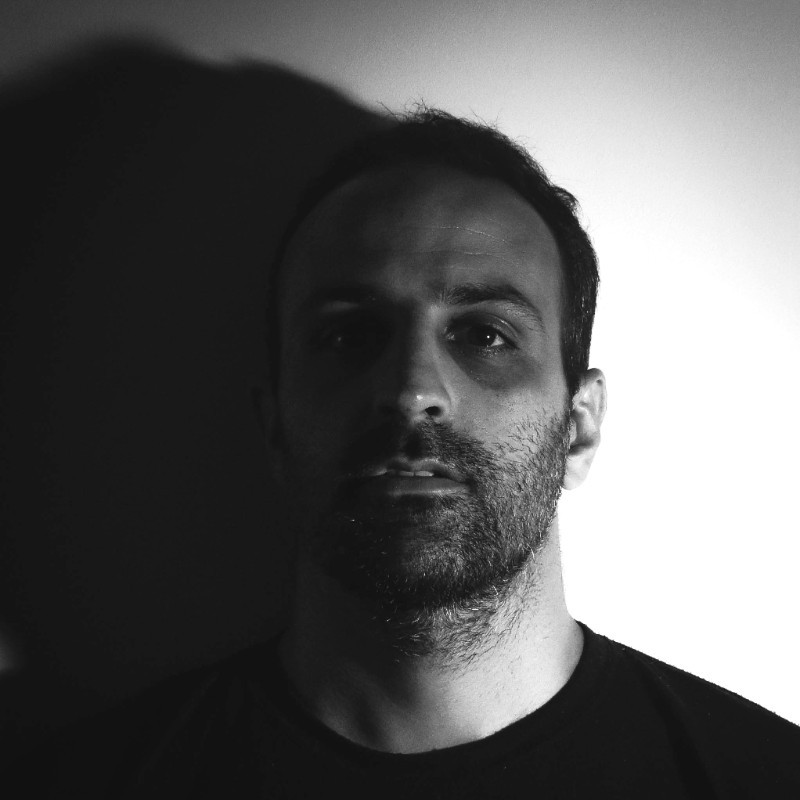
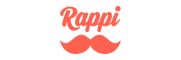