Practical Uses of PHP: Building a Simple Chat Application
PHP (Hypertext Preprocessor) is a versatile server-side scripting language widely used for web development. Its ease of use, extensive community support, and seamless integration with HTML make it a popular choice for building dynamic web applications. In this blog, we will explore the practical uses of PHP by creating a simple chat application from scratch. Building a chat application is an excellent way to understand the core concepts of PHP and real-time communication, and it serves as a foundation for more complex projects like messaging platforms or social networks.
Before diving into the development process, let’s discuss the essential features of our chat application:
- Real-Time Messaging: The chat application should facilitate real-time messaging between users, enabling them to exchange messages instantly.
- User Authentication: To ensure the privacy of conversations, users must log in to the chat application before participating in any discussions.
- Message History: Users should be able to view their previous conversations and history, ensuring they don’t miss any important messages.
- Online Status: Displaying the online status of users will provide valuable context and help others identify active participants.
Now that we have a clear understanding of our objectives let’s proceed to build our simple chat application step by step.
Step 1: Setting Up the Environment
Before starting, ensure you have a working environment with PHP, a web server (like Apache or Nginx), and a database (MySQL, for instance) installed. For this tutorial, we will use PHP 7.4 and MySQL.
Step 2: Creating the Database
Our chat application will require a database to store user information, messages, and their timestamps. Begin by creating a database named “chat_app” and two tables, “users” and “messages.”
sql CREATE DATABASE chat_app; USE chat_app; CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, username VARCHAR(50) NOT NULL, password VARCHAR(255) NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ); CREATE TABLE messages ( id INT AUTO_INCREMENT PRIMARY KEY, sender_id INT NOT NULL, receiver_id INT NOT NULL, message TEXT NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
Step 3: User Registration and Login
Implement user registration and login functionalities to ensure secure access to the chat application.
User Registration (register.php)
php <?php // Include the database connection file here if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST["username"]; $password = password_hash($_POST["password"], PASSWORD_DEFAULT); // Insert user data into the 'users' table $sql = "INSERT INTO users (username, password) VALUES ('$username', '$password')"; // Execute the SQL query here // Redirect the user to the login page after successful registration header("Location: login.php"); exit; } ?> <!DOCTYPE html> <html> <head> <title>Chat App - Register</title> </head> <body> <h1>Register</h1> <form method="post" action=""> <label for="username">Username:</label> <input type="text" name="username" required> <br> <label for="password">Password:</label> <input type="password" name="password" required> <br> <input type="submit" value="Register"> </form> </body> </html>
User Login (login.php)
php <?php // Include the database connection file here if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST["username"]; $password = $_POST["password"]; // Fetch user data from the 'users' table based on the username $sql = "SELECT id, username, password FROM users WHERE username = '$username'"; // Execute the SQL query here // Check if the user exists and the provided password matches the stored password if ($row = /* Fetch the result row here */) { if (password_verify($password, $row["password"])) { // Start a session and store user information session_start(); $_SESSION["user_id"] = $row["id"]; $_SESSION["username"] = $row["username"]; // Redirect the user to the chat page after successful login header("Location: chat.php"); exit; } else { $error = "Invalid password"; } } else { $error = "User not found"; } } ?> <!DOCTYPE html> <html> <head> <title>Chat App - Login</title> </head> <body> <h1>Login</h1> <form method="post" action=""> <label for="username">Username:</label> <input type="text" name="username" required> <br> <label for="password">Password:</label> <input type="password" name="password" required> <br> <input type="submit" value="Login"> </form> <?php if (isset($error)): ?> <p><?php echo $error; ?></p> <?php endif; ?> </body> </html>
Step 4: Creating the Chat Interface
Now that we have user registration and login in place, let’s move on to the main part of our chat application—the chat interface.
Chat Interface (chat.php)
php <?php // Include the database connection file here // Check if the user is logged in; otherwise, redirect to the login page session_start(); if (!isset($_SESSION["user_id"])) { header("Location: login.php"); exit; } // Fetch the user's information from the database $user_id = $_SESSION["user_id"]; $sql = "SELECT * FROM users WHERE id = '$user_id'"; // Execute the SQL query here // Display the chat interface ?> <!DOCTYPE html> <html> <head> <title>Chat App - Chat</title> <script> // JavaScript code for real-time message exchange will go here </script> </head> <body> <h1>Welcome, <?php echo $_SESSION["username"]; ?></h1> <div id="chat-box"> <!-- The chat messages will be displayed here --> </div> <form method="post" action=""> <input type="text" name="message" required> <input type="submit" value="Send"> </form> </body> </html>
Step 5: Implementing Real-Time Messaging
To enable real-time messaging, we’ll use JavaScript with PHP. We’ll create a script to fetch and display new messages without requiring a page refresh.
javascript // JavaScript (chat.js) // Function to fetch new messages and update the chat-box function fetchMessages() { // Use AJAX to send a request to a PHP script to fetch new messages // and update the chat-box } // Function to send a new message function sendMessage() { // Use AJAX to send a request to a PHP script to store the new message in the database // and update the chat-box } // Call fetchMessages() to load initial messages fetchMessages(); // Set an interval to call fetchMessages() every few seconds for real-time updates setInterval(fetchMessages, 3000);
Chat Interface with Real-Time Messaging (chat.php)
php <!DOCTYPE html> <html> <head> <title>Chat App - Chat</title> <script src="chat.js"></script> </head> <body> <h1>Welcome, <?php echo $_SESSION["username"]; ?></h1> <div id="chat-box"> <!-- The chat messages will be displayed here --> </div> <form onsubmit="sendMessage(); return false;"> <input type="text" name="message" required> <input type="submit" value="Send"> </form> </body> </html>
Conclusion
Congratulations! You’ve successfully built a simple chat application using PHP. Throughout this tutorial, we’ve covered essential concepts like user registration, login, database integration, and real-time messaging. This chat application can serve as a foundation for more advanced projects, and you can enhance it further by adding features like group chats, file sharing, and message notifications. Happy coding!
Remember, building a chat application is just one of the many practical uses of PHP. Its flexibility and extensive community support open the door to various web development opportunities. Whether you’re working on personal projects, web applications, or e-commerce platforms, PHP is a valuable tool to consider in your tech stack. Happy coding and exploring the vast possibilities with PHP!
Table of Contents
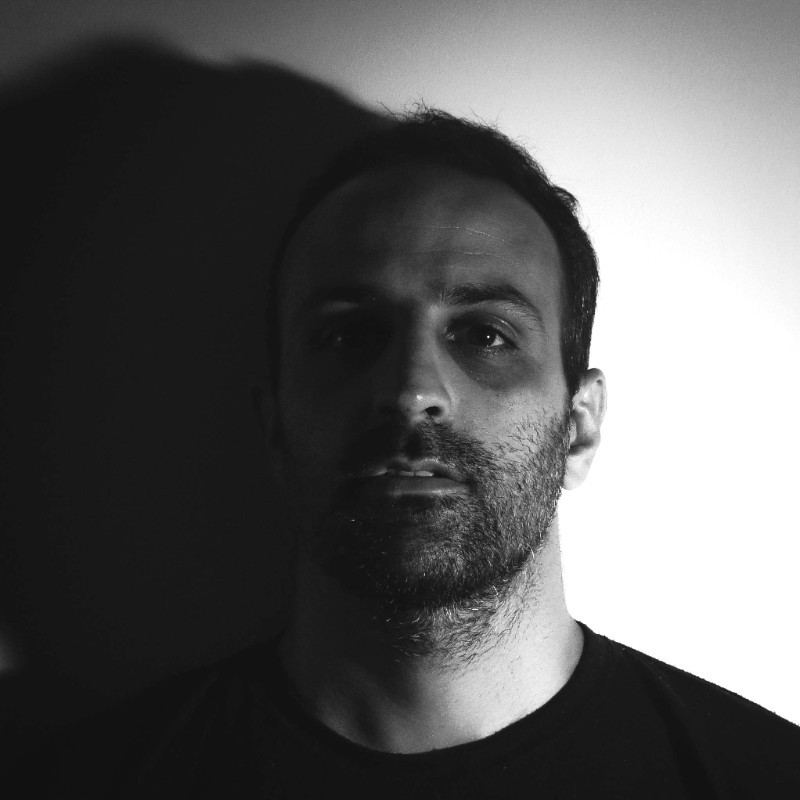
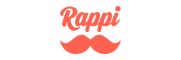