Discovering the Power of PHP’s str_replace()
In the world of web development, the ability to manipulate strings efficiently is a fundamental skill. Whether you’re building a simple website or a complex web application, working with strings is inevitable. This is where PHP’s str_replace() function comes to the rescue. With its versatility and power, str_replace() allows developers to perform advanced string replacements and transformations effortlessly. In this guide, we’ll delve into the capabilities of str_replace(), exploring its usage, examples, and providing you with valuable tips to harness its full potential.
1. Understanding str_replace()
str_replace() is a built-in PHP function designed to replace all occurrences of a search string with a replacement string within a given string. This might sound like a straightforward task, but the function’s flexibility extends far beyond simple search and replace operations. Its basic syntax is as follows:
php str_replace($search, $replace, $subject);
- $search: The string or array of strings to search for.
- $replace: The string or array of strings to replace with.
- $subject: The input string or an array of input strings where replacements will be performed.
2. Basic Usage
Let’s start with a basic example to demonstrate how str_replace() works. Imagine you have a sentence with a placeholder that you want to replace with a specific word:
php $originalSentence = "Hello, [name]! How are you doing, [name]?"; $replacement = "John"; $modifiedSentence = str_replace("[name]", $replacement, $originalSentence); echo $modifiedSentence;
In this example, the function replaces both occurrences of [name] with “John,” resulting in the output:
sql Hello, John! How are you doing, John?
3. Replacing Case-Insensitively
By default, str_replace() is case-sensitive, meaning it will only replace strings that match the case of the search string. However, you can easily perform case-insensitive replacements by using the str_ireplace() function instead:
php $text = "PHP is popular, php is versatile, and PHP is fun."; $search = "php"; $replace = "PHP"; $modifiedText = str_ireplace($search, $replace, $text); echo $modifiedText;
In this case, all occurrences of “php” (regardless of case) will be replaced with “PHP.”
4. Handling Arrays
str_replace() can also work with arrays of search and replace strings. This opens up opportunities for batch replacements and more complex transformations. Consider the following example:
php $keywords = ["apple", "banana", "orange"]; $text = "I like apple, but bananas are my favorite."; $replacements = ["fruit", "fruit", "fruit"]; $modifiedText = str_replace($keywords, $replacements, $text); echo $modifiedText;
The output will be:
sql I like fruit, but fruits are my favorite.
5. Managing Multiple Replacements
What if you want to perform multiple replacements in a single call? str_replace() has you covered. You can pass arrays of search and replace strings, and the function will perform all the replacements:
php $search = ["apple", "banana", "orange"]; $replace = ["fruit", "fruit", "fruit"]; $text = "I like apple, banana, and orange."; $modifiedText = str_replace($search, $replace, $text); echo $modifiedText;
The result will be:
css I like fruit, fruit, and fruit.
6. Handling Nested Replacements
In some scenarios, you might want to perform replacements based on the previous replacements. Let’s say you have a text containing placeholders for both names and dates:
php $text = "Hello [name], today is [date]."; $replacements = [ "[name]" => "Alice", "[date]" => "August 19, 2023", ]; $modifiedText = str_replace(array_keys($replacements), array_values($replacements), $text); echo $modifiedText;
By using associative arrays and array_keys() and array_values(), you can achieve nested replacements, resulting in the output:
csharp Hello Alice, today is August 19, 2023.
7. Tips for Effective Use
- Use Arrays for Bulk Replacements: If you need to perform multiple replacements, especially when dealing with large amounts of text, using arrays can greatly enhance your efficiency.
- Escape Special Characters: When working with search strings that contain special characters (e.g., $, .), remember to escape them using the preg_quote() function. This ensures that the search operates correctly without unintended consequences.
- Order of Replacements Matters: The order in which you list the search strings in an array can affect the final output. Be mindful of the sequence to achieve the desired result.
- Use strtr() for Single-Character Replacements: If you only need to replace single characters, strtr() might be more efficient than str_replace(), as it’s optimized for this specific use case.
- Consider Using Regular Expressions: For advanced and complex replacements, regular expressions offer even greater flexibility. The preg_replace() function provides support for pattern-based replacements.
Conclusion
PHP’s str_replace() function is a versatile tool that empowers developers to manipulate strings effectively. From basic search and replace tasks to intricate transformations, its capabilities are extensive. By mastering its usage and understanding its nuances, you can streamline your string manipulation tasks and create more efficient and dynamic web applications. Remember the tips and techniques outlined in this guide to make the most out of str_replace() and elevate your web development skills to new heights.
Table of Contents
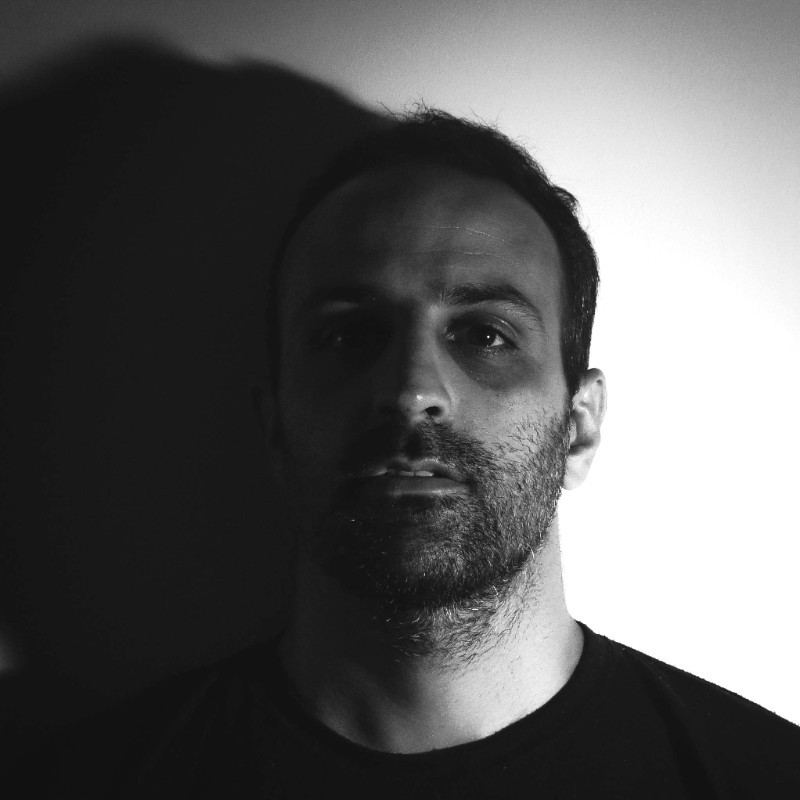
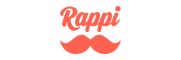