PHP’s str_shuffle() Function: Creating Randomness in Strings
In the world of web development, creating randomness in strings is a common requirement. Whether you’re building a password generator, a CAPTCHA system, or just adding a touch of unpredictability to your data, PHP’s str_shuffle() function is your go-to tool. In this blog, we’ll dive deep into this versatile function, exploring its capabilities, use cases, and providing you with practical examples.
Table of Contents
1. Understanding the Basics of str_shuffle()
At its core, the str_shuffle() function is designed to shuffle the characters of a given string randomly. This can be incredibly useful when you want to generate random passwords or add an element of surprise to your web applications.
1.1. Syntax
Here’s the basic syntax of the str_shuffle() function:
php str_shuffle(string $string): string
- string: The input string you want to shuffle.
- Return Value: A shuffled version of the input string.
Now, let’s take a closer look at how this function works and how you can harness its power.
2. Shuffling a String
Shuffling a string with str_shuffle() is straightforward. You provide the function with the string you want to shuffle, and it returns a shuffled version of that string. Here’s a simple example:
php $inputString = "Hello, World!"; $shuffledString = str_shuffle($inputString); echo $shuffledString;
In this case, the output could be something like “d,leH olroW!”. Each time you run the script, you’ll get a different result, showcasing the randomness introduced by str_shuffle().
3. Generating Random Passwords
One of the most common use cases for str_shuffle() is generating random passwords. Let’s see how you can create a random password generator using this function:
php function generateRandomPassword($length = 12) { $characters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789'; $password = ''; for ($i = 0; $i < $length; $i++) { $password .= $characters[rand(0, strlen($characters) - 1)]; } return $password; } $randomPassword = generateRandomPassword(); echo $randomPassword;
In this code snippet, we define a function generateRandomPassword() that takes an optional $length parameter, which determines the length of the generated password. We also define a set of characters from which the password will be composed. Inside the loop, we use str_shuffle() to shuffle the characters randomly, and the result is a secure, random password.
4. Adding Randomness to Data
str_shuffle() isn’t limited to just shuffling letters and numbers. You can use it to add randomness to any kind of data, such as filenames, IDs, or even text for games. Let’s explore how you can use it in practical scenarios.
4.1. Shuffling a List of Items
Imagine you have a list of items, and you want to display them in random order each time a user visits your website. You can easily achieve this with str_shuffle():
php $items = ['Item 1', 'Item 2', 'Item 3', 'Item 4', 'Item 5']; $shuffledItems = str_shuffle(implode(',', $items)); $shuffledItemsArray = explode(',', $shuffledItems); foreach ($shuffledItemsArray as $item) { echo $item . '<br>'; }
In this example, we start with an array of items and shuffle them by converting the array into a comma-separated string, shuffling it, and then converting it back into an array. The result is a randomized list that you can display on your website.
4.2. Creating Random IDs
When working with databases or managing resources, generating unique and random IDs is crucial. str_shuffle() can help you create random IDs that are hard to predict. Here’s a simple example of generating random IDs for user profiles:
php function generateRandomId($length = 10) { $characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'; $randomId = str_shuffle($characters); return substr($randomId, 0, $length); } $userId = generateRandomId(8); echo 'User ID: ' . $userId;
In this code, we create a function generateRandomId() that generates random IDs using a combination of letters and numbers. By specifying the length of the ID you want, you can control how long and random the IDs will be.
5. Practical Considerations
While str_shuffle() is a handy function for generating randomness in strings, there are some important considerations to keep in mind:
5.1. Limited Randomness
The randomness provided by str_shuffle() is limited to the input string’s characters. If you shuffle a string composed entirely of ‘A’s, you’ll still get a string with only ‘A’sβit’ll just be shuffled differently each time. To achieve true randomness, you need to provide a diverse set of characters in your input string.
5.2. Performance
For very long strings, shuffling with str_shuffle() can be a performance concern, as it requires creating a new string of the same length and shuffling the characters. If you’re working with extremely long strings, consider alternative approaches to achieve the desired randomness.
5.3. Cryptographically Secure Randomness
If you need to generate random data for security purposes, such as generating secure tokens or passwords, consider using PHP’s random_bytes() or random_int() functions, which provide cryptographically secure randomness. These functions are designed to generate random data suitable for encryption and authentication.
Conclusion
PHP’s str_shuffle() function is a valuable tool in your web development arsenal when you need to introduce randomness to strings. Whether you’re creating random passwords, shuffling lists, or generating unique IDs, str_shuffle() offers a straightforward way to achieve your goals. Just remember to consider the limitations and performance implications when using this function and opt for more secure methods when dealing with sensitive data. With the right approach, you can add a touch of unpredictability to your web applications and enhance their functionality and security. Happy shuffling!
Table of Contents
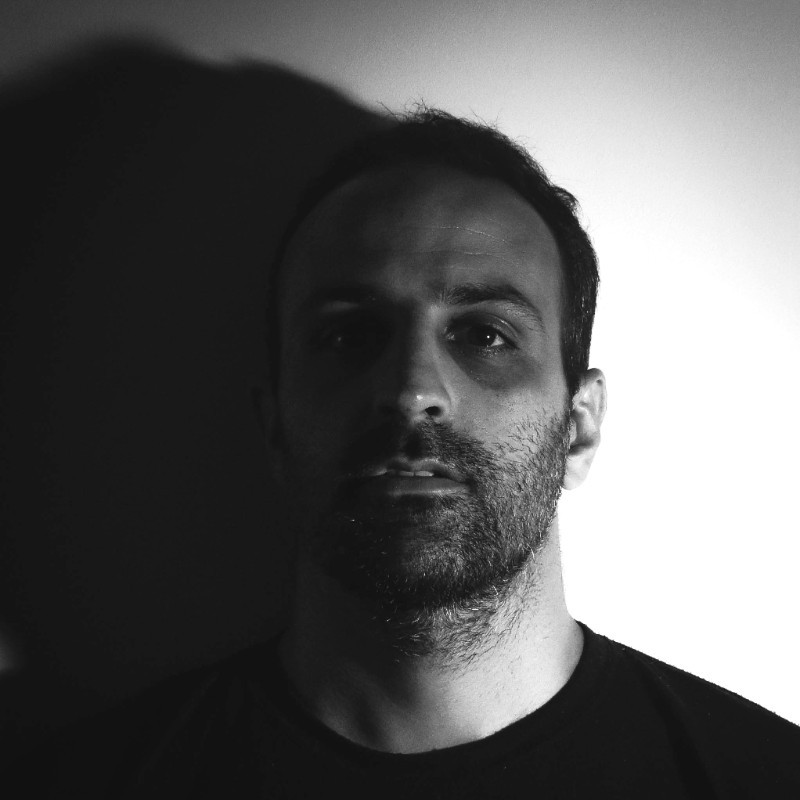
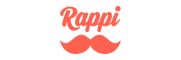