PHP’s strlen() Function: Measuring String Lengths
When working with PHP, it’s common to deal with strings of various lengths. Whether you’re handling user input, processing data from databases, or manipulating text, knowing the length of a string is often essential. PHP provides a handy function called strlen() that allows you to determine the length of a string quickly and easily. In this blog post, we’ll dive into the details of PHP’s strlen() function, explore its usage, and cover best practices.
Table of Contents
1. What is strlen()?
The strlen() function in PHP is a built-in function that returns the length of a given string. It counts the number of bytes in the string, which means it’s not necessarily the same as the number of characters. This is an important distinction, especially when dealing with multibyte character encodings like UTF-8, where a single character can consist of multiple bytes.
1.1. Syntax
The basic syntax of strlen() is as follows:
php strlen(string $string): int
Here’s a breakdown of the parameters and return value:
- $string: The input string whose length you want to determine.
- Return Value: An integer representing the length of the input string in bytes.
2. Using strlen()
Now that we understand the syntax of strlen(), let’s look at some practical examples of how to use it.
Example 1: Basic Usage
php $string = "Hello, World!"; $length = strlen($string); echo "The length of the string is: $length"; // Output: The length of the string is: 13
In this example, we create a string, “Hello, World!” and use strlen() to determine its length. The length of this string is 13 characters, including spaces and punctuation.
Example 2: Handling Multibyte Characters
When working with multibyte character encodings like UTF-8, strlen() may not give you the expected results because it counts bytes, not characters. To accurately count characters in such cases, you should use mb_strlen() from the mbstring extension.
php $string = "?????????"; // Japanese greeting $length = mb_strlen($string, 'UTF-8'); echo "The length of the string is: $length"; // Output: The length of the string is: 7
In this example, we have a string in Japanese, and using mb_strlen() with the UTF-8 encoding correctly returns the character count as 7.
Example 3: Handling Empty Strings
When dealing with strings, it’s important to consider edge cases like empty strings. Here’s how strlen() behaves with empty strings:
php $emptyString = ""; $length = strlen($emptyString);
echo “The length of the empty string is: $length”; // Output: The length of the empty string is: 0
As demonstrated, strlen() correctly returns a length of 0 for an empty string.
3. Best Practices
While strlen() is a straightforward function, there are some best practices to keep in mind when using it in your PHP code:
3.1. Encoding Awareness
Always be aware of the character encoding used in your strings. If you’re working with multibyte character encodings, consider using mb_strlen() for accurate character counts.
3.2. Error Handling
In some cases, you might encounter situations where the input is not a string. To avoid errors, you can use the is_string() function to check if the input is a valid string before calling strlen().
php if (is_string($string)) { $length = strlen($string); } else { // Handle the case where $string is not a string. }
3.3. Null and Empty Strings
Handle null and empty strings gracefully in your code. Use conditional statements to check for these cases before calling strlen() to avoid unexpected results.
php if (!empty($string)) { $length = strlen($string); } else { // Handle the case of null or empty string. }
3.4. Performance Considerations
For very long strings, calling strlen() can be a performance bottleneck. If you only need to check if a string is empty or not, you can use empty() or simply check the string’s length against 0 without calling strlen().
php if (strlen($string) > 0) { // String is not empty. }
4. Common Use Cases
Understanding how to use strlen() effectively can be valuable in various scenarios. Here are some common use cases:
4.1. Form Validation
When building web forms, you often need to validate user input, including checking if a string exceeds a certain length limit.
php if (strlen($username) > 20) { // Username is too long. // Display an error message. }
4.2. Truncating Text
You may want to truncate long text to fit within a specified length, such as when displaying excerpts or summaries.
php $maxLength = 100; if (strlen($text) > $maxLength) { $truncatedText = substr($text, 0, $maxLength) . '...'; // Display $truncatedText. }
4.3. Password Strength Checking
When users create passwords, you can use strlen() to enforce minimum and maximum length requirements.
php if (strlen($password) < 8) { // Password is too short. // Display an error message. }
4.4. Generating Random Strings
When generating random strings for various purposes, you may need to ensure a specific length.
php $randomString = generateRandomString(10); // Generate a 10-character random string.
Conclusion
PHP’s strlen() function is a fundamental tool for working with strings in your web applications. It provides a quick and easy way to determine the length of a string, making it essential for tasks like form validation, text truncation, and more. However, it’s crucial to be aware of character encodings and handle edge cases gracefully to ensure your code functions correctly. By following best practices and understanding its limitations, you can leverage strlen() effectively in your PHP projects, saving time and reducing errors.
Table of Contents
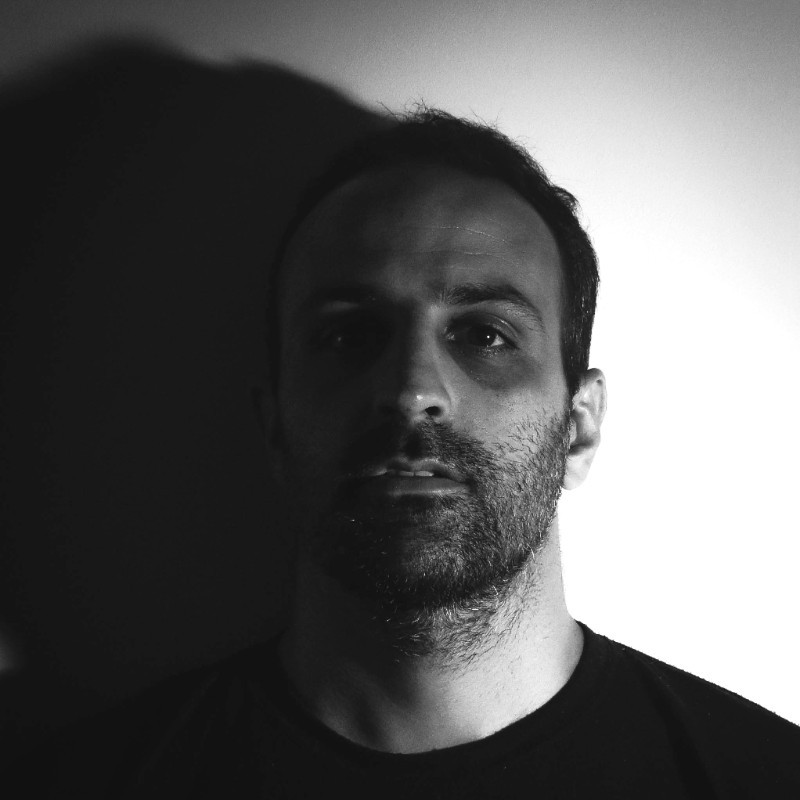
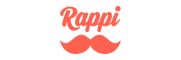