Understanding PHP’s strip_tags() Function
When it comes to handling user-generated content on your website or application, security and data integrity are of paramount importance. Users can submit a variety of content, including text, images, and more. However, this content may contain potentially harmful HTML or JavaScript code that can jeopardize your site’s security or disrupt its layout.
Table of Contents
This is where PHP’s strip_tags() function comes into play. It is a versatile and crucial tool for cleaning and securing user-generated content. In this comprehensive guide, we will explore the strip_tags() function in-depth, covering its usage, benefits, and potential pitfalls.
1. What is strip_tags()?
strip_tags() is a built-in PHP function designed to remove HTML and PHP tags from a given string. Its primary purpose is to sanitize user input or any text containing markup tags to prevent code injection, cross-site scripting (XSS) attacks, and other security vulnerabilities.
The basic syntax of the strip_tags() function is as follows:
php strip_tags(string $str, string $allowed_tags = null): string
- $str: The input string containing HTML or PHP tags.
- $allowed_tags (optional): A list of tags that you want to allow in the output. All other tags will be removed.
2. Using strip_tags() for Basic HTML Sanitization
Let’s start with a simple example to illustrate how strip_tags() works for basic HTML sanitization. Consider the following PHP code:
php $input = '<p>Hello, <strong>world</strong>!</p>'; $cleaned = strip_tags($input); echo $cleaned;
In this example, the $input variable contains an HTML string with a paragraph (<p>) and a strong (<strong>) tag. When we apply strip_tags() to this input, the function will remove all HTML tags, resulting in the following output:
Hello, world!
As you can see, strip_tags() effectively strips away the HTML tags, leaving only the plain text. This is useful when you want to display user-generated content in a safe and controlled manner, ensuring that any potentially harmful tags are removed.
3. Allowing Specific Tags
While removing all HTML tags is useful in many scenarios, there might be cases where you want to allow certain tags to preserve the formatting and structure of the text. You can achieve this by specifying the allowed tags as the second argument to strip_tags().
Let’s say you want to allow the <strong> tag in addition to plain text. Here’s how you can do it:
php $input = '<p>Hello, <strong>world</strong>!</p>'; $cleaned = strip_tags($input, '<strong>'); echo $cleaned;
With the <strong> tag allowed, the output will be:
css Hello, <strong>world</strong>!
In this example, the <strong> tag is retained while all other tags are removed. This selective approach allows you to maintain some HTML structure while still ensuring security.
4. Removing Attributes
In addition to removing HTML tags, strip_tags() can also remove attributes associated with those tags. This can be particularly useful when you want to eliminate any potentially harmful attributes from user-generated content.
Let’s consider an example:
php $input = '<a href="https://example.com" onclick="alert(\'Hello, world!\')">Visit Example</a>'; $cleaned = strip_tags($input); echo $cleaned;
In this case, the $input variable contains an anchor (<a>) tag with both href and onclick attributes. When we apply strip_tags() to this input, it will not only remove the <a> tag but also strip away the attributes, resulting in the following output:
Visit Example
By default, strip_tags() removes both tags and their associated attributes, enhancing the security of your application.
5. Advanced Usage: Custom Tag Handling
While strip_tags() is handy for most basic sanitization tasks, you may encounter scenarios where you need more control over tag handling. For such cases, you can define custom handling of specific tags using a callback function.
Here’s an example:
php function customTagHandler($tag, $content) { if ($tag === 'a') { return '<a href="' . strip_tags($content) . '">Link</a>'; } return strip_tags($content); } $input = '<p>Hello, <a href="https://example.com">world</a>!</p>'; $cleaned = strip_tags($input, '<a>', 'customTagHandler'); echo $cleaned;
In this example, we define a custom tag handler function customTagHandler(). When strip_tags() encounters an anchor (<a>) tag, it calls this function to process the tag. In this case, we modify the anchor tag by removing any nested tags while retaining the link itself. All other tags are processed as usual.
The output will be:
javascript Hello, <a href="https://example.com">world</a>!
Custom tag handling provides you with fine-grained control over how specific tags are processed, allowing you to tailor the sanitization process to your specific requirements.
6. Common Use Cases
Now that you have a solid understanding of how strip_tags() works and its various capabilities, let’s explore some common use cases where this function can be incredibly helpful.
6.1. Cleaning User-Generated Content
When users can submit content to your website, forum, or blog, there’s a risk that they might include malicious scripts or HTML tags. By using strip_tags(), you can ensure that user-generated content is sanitized and safe to display.
6.2. Preventing Cross-Site Scripting (XSS) Attacks
XSS attacks occur when malicious code is injected into web pages and executed in users’ browsers. strip_tags() can help prevent XSS attacks by removing or neutralizing potentially harmful scripts and tags.
6.3. Formatting and Displaying Content
In some cases, you may want to allow certain HTML tags for formatting purposes while still ensuring security. For example, you can allow <em> and <strong> tags to preserve italic and bold formatting in user-generated text.
6.4. Email Content Sanitization
If your application allows users to send emails with HTML content, it’s crucial to sanitize the HTML to prevent email clients from rendering malicious code. strip_tags() can be used to clean HTML email content effectively.
7. Potential Pitfalls
While strip_tags() is a powerful tool for sanitizing user-generated content, it’s important to be aware of its limitations and potential pitfalls.
7.1. Limited XSS Protection
While strip_tags() can help mitigate XSS attacks, it’s not a foolproof solution. Determined attackers can find ways to bypass it or use other techniques to execute malicious code. To enhance security, consider using additional security measures such as input validation and output encoding.
7.2. Loss of Formatting
When you remove HTML tags with strip_tags(), you may lose some formatting and styling from the original content. If preserving formatting is essential, you may need to allow specific formatting tags while still being cautious about security.
7.3. Context Matters
The effectiveness of strip_tags() depends on the context in which it’s used. Different content and situations may require different sanitization approaches. Always consider the specific needs of your application when using this function.
Conclusion
PHP’s strip_tags() function is a valuable tool for sanitizing and securing user-generated content. It allows you to remove HTML and PHP tags, preventing potential security vulnerabilities while still maintaining the integrity of the text. By understanding its usage, benefits, and potential pitfalls, you can make informed decisions about how to use this function effectively in your PHP applications. Remember to combine it with other security practices to ensure a robust defense against web-based threats.
Table of Contents
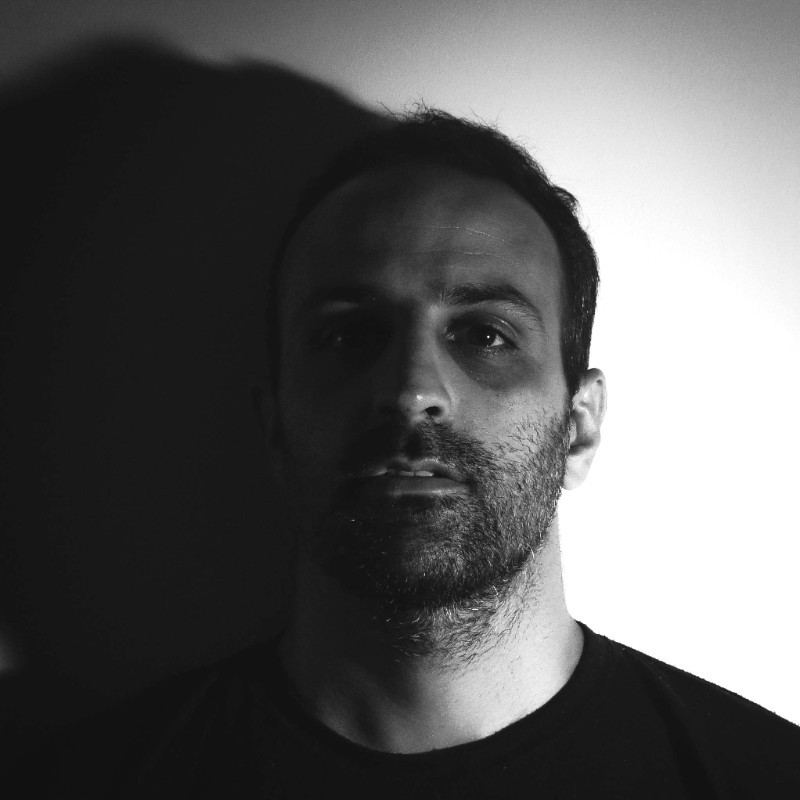
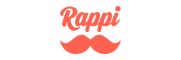