Exploring PHP’s strrev() Function: Reversing Strings
In the world of web development, string manipulation is a common task. Whether it’s processing user inputs, generating dynamic content, or performing data transformations, developers frequently need to work with strings in various ways. One such essential operation is reversing a string, and PHP offers a convenient solution for this task through the strrev() function. In this blog post, we’ll delve into the workings of strrev(), its syntax, use cases, and provide hands-on code examples to illustrate its functionality.
Table of Contents
1. Understanding the Basics
1.1. What is the strrev() Function?
The strrev() function in PHP is a powerful tool designed specifically for reversing strings. It takes a single argument, the input string that you want to reverse, and returns a new string with the characters in reverse order. This can be incredibly useful in scenarios such as displaying text in a mirror-like fashion, checking for palindromes, or simply manipulating strings for creative purposes.
1.2. Syntax of strrev()
The syntax of the strrev() function is straightforward:
php string strrev ( string $string )
Here, $string is the input string that you want to reverse. The function returns the reversed string.
2. Practical Examples
Let’s dive into some practical examples to better understand how the strrev() function works and when it can be particularly handy.
Example 1: Basic String Reversal
php $input = "Hello, World!"; $reversed = strrev($input); echo $reversed; // Output: "!dlroW ,olleH"
In this example, the function takes the input string “Hello, World!” and returns a reversed string “!dlroW ,olleH”. This demonstrates the fundamental usage of the strrev() function.
Example 2: Palindrome Checking
A palindrome is a word, phrase, or sequence of characters that reads the same forward and backward (ignoring spaces and punctuation). The strrev() function can be used to check if a string is a palindrome.
php function isPalindrome($string) { $cleanedString = preg_replace('/[^A-Za-z0-9]/', '', $string); $reversedString = strrev($cleanedString); return strtolower($cleanedString) === strtolower($reversedString); } $input1 = "racecar"; $input2 = "Hello"; $input3 = "A man, a plan, a canal, Panama!"; var_dump(isPalindrome($input1)); // Output: bool(true) var_dump(isPalindrome($input2)); // Output: bool(false) var_dump(isPalindrome($input3)); // Output: bool(true)
The isPalindrome() function first cleans the input string by removing non-alphanumeric characters and spaces. Then, it compares the cleaned string with its reversed version to determine if it’s a palindrome.
Example 3: Creative Text Effects
The ability to reverse strings opens up opportunities for creative text effects on websites or applications. Let’s look at a simple example where we reverse individual words in a sentence:
php function reverseWordsInSentence($sentence) { $words = explode(' ', $sentence); $reversedWords = array_map('strrev', $words); return implode(' ', $reversedWords); } $inputSentence = "PHP is an amazing scripting language"; $reversedSentence = reverseWordsInSentence($inputSentence); echo $reversedSentence; // Output: "PHP si na gnizama gnitpics egaugnal"
In this example, the function reverseWordsInSentence() splits the input sentence into words, reverses each word using strrev(), and then joins the reversed words back into a sentence.
3. Advantages and Considerations
3.1. Advantages of Using strrev()
- Simplicity: The strrev() function offers a straightforward way to reverse strings without the need for complex logic.
- Performance: While it’s possible to implement string reversal through loops or other methods, the built-in strrev() function is often optimized for performance, making it a reliable choice for handling large strings.
- Code Readability: Utilizing strrev() can enhance code readability and maintainability by encapsulating the reversal logic in a concise function call.
3.2. Considerations and Limitations
- Character Encoding: It’s important to note that strrev() operates on a byte level rather than a character level. This means that for multi-byte character encodings like UTF-8, the function might not work as expected, potentially leading to scrambled characters.
- Alternative Approaches: If you’re working with multi-byte character encodings or require more complex string manipulations, you might need to explore alternative approaches, such as using the mb_strrev() function from the mbstring extension.
Conclusion
The strrev() function in PHP is a versatile tool for reversing strings, offering simplicity and performance benefits for various string manipulation tasks. From creating mirror-like text effects to checking for palindromes, strrev() can be an indispensable part of your string manipulation toolkit. However, it’s essential to be mindful of its limitations, particularly when dealing with multi-byte character encodings. As you continue to explore PHP’s capabilities, keep the strrev() function in mind as a valuable asset in your coding journey.
Table of Contents
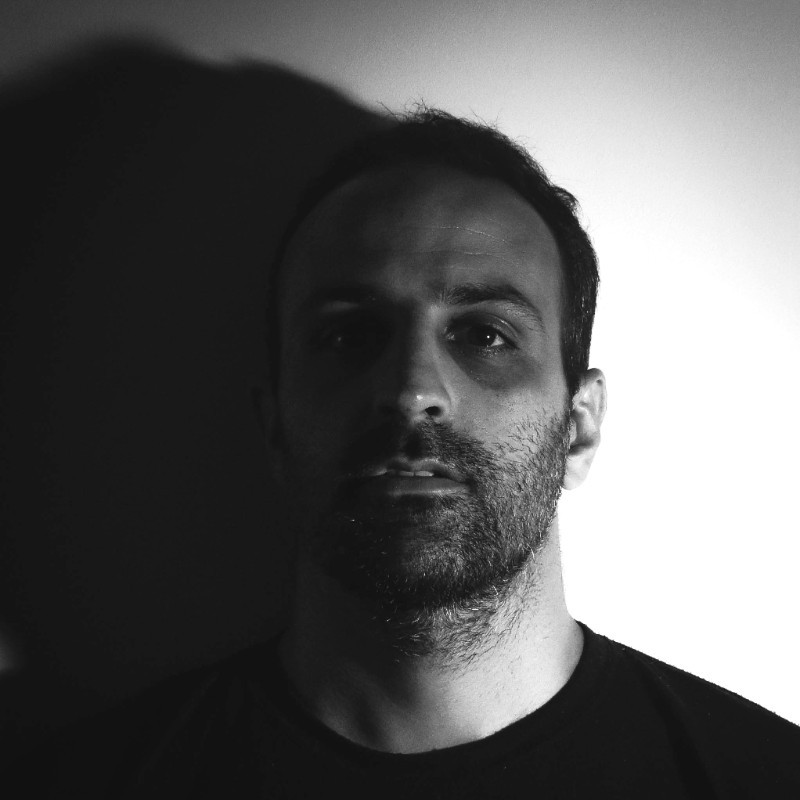
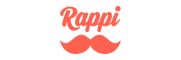