PHP’s strtotime(): A Comprehensive Guide
When it comes to working with dates and times in PHP, the strtotime() function is a powerful tool in your arsenal. Whether you need to parse dates from user input, calculate future or past dates, or perform complex date arithmetic, strtotime() has got you covered. In this comprehensive guide, we will dive deep into the world of strtotime() and explore its various capabilities and use cases.
Table of Contents
1. Understanding strtotime()
Before we delve into the intricacies of strtotime(), let’s start with the basics. At its core, strtotime() is a PHP function that parses a date string and returns a Unix timestamp representing that date and time. A Unix timestamp is a numeric value representing the number of seconds since January 1, 1970, 00:00:00 UTC (Coordinated Universal Time).
1.1. Syntax
The basic syntax of strtotime() is as follows:
php strtotime(string $time, int $now = time())
- $time: A string containing the date and time you want to parse.
- $now (optional): A timestamp used as a reference point for relative calculations. If not provided, the current timestamp is used.
1.2. Examples
Let’s start with some simple examples to illustrate how strtotime() works:
Example 1: Parsing a Date
php $dateString = "2023-09-30"; $timestamp = strtotime($dateString);
echo $timestamp; // Output: 1693488000
In this example, we provide a date string in the “YYYY-MM-DD” format, and strtotime() returns the Unix timestamp for September 30, 2023, at 00:00:00 UTC.
Example 2: Parsing a Date and Time
php $dateTimeString = "2023-09-30 15:30:00"; $timestamp = strtotime($dateTimeString); echo $timestamp; // Output: 1693563000
Here, we provide a date and time string in the “YYYY-MM-DD HH:MM:SS” format, and strtotime() returns the Unix timestamp for September 30, 2023, at 15:30:00 UTC.
Example 3: Relative Time
php $timestamp = strtotime("+1 week"); echo $timestamp; // Output: A timestamp representing one week from the current time
In this example, we use a relative time string (“+1 week”) to calculate a timestamp one week from the current time.
2. Working with Relative Dates
One of the most powerful features of strtotime() is its ability to work with relative dates and times. You can specify date intervals, such as “next week,” “last Monday,” or “2 days ago,” and strtotime() will calculate the corresponding timestamp for you.
2.1. Relative Date Formats
Here are some common relative date formats you can use with strtotime():
- “now”: Represents the current timestamp.
- “tomorrow” or “next day”: Represents the timestamp for the next day.
- “yesterday” or “last day”: Represents the timestamp for the previous day.
- “next week” or “last week”: Represents the timestamp for the next or previous week.
- “next month” or “last month”: Represents the timestamp for the next or previous month.
- “next year” or “last year”: Represents the timestamp for the next or previous year.
- “first day of next month”: Represents the timestamp for the first day of the next month.
- “last day of last month”: Represents the timestamp for the last day of the previous month.
2.2. Examples
Let’s explore some examples to see how relative dates work with strtotime():
Example 4: Relative Dates
php $now = time(); // Current timestamp $nextWeek = strtotime("next week", $now); echo date("Y-m-d", $nextWeek); // Output: Next week's date $lastMonday = strtotime("last Monday", $now); echo date("Y-m-d", $lastMonday); // Output: Last Monday's date $twoDaysAgo = strtotime("2 days ago", $now); echo date("Y-m-d", $twoDaysAgo); // Output: Two days ago's date
In these examples, we use various relative date strings with strtotime() to calculate timestamps for different dates. You can easily adapt this to create dynamic date ranges for your applications.
3. Advanced Usage
While strtotime() is great for basic date and time parsing, it becomes even more powerful when combined with more advanced techniques. Let’s explore some of these techniques:
3.1. Combining strtotime() with date()
The date() function in PHP allows you to format a Unix timestamp into a human-readable date string. When used in conjunction with strtotime(), you can parse a date string, manipulate it, and then format it as needed.
Example 5: Formatting Dates
php $dateString = "2023-09-30"; $timestamp = strtotime($dateString); $formattedDate = date("F j, Y", $timestamp); echo $formattedDate; // Output: September 30, 2023
In this example, we parse a date string using strtotime() and then format it into a more user-friendly format using date().
3.2. Calculating Time Differences
You can use strtotime() to calculate the time difference between two timestamps, making it useful for implementing features like countdown timers or age calculators.
Example 6: Calculating Time Difference
php $birthday = strtotime("1990-05-20"); $currentDate = time(); $ageInSeconds = $currentDate - $birthday; $years = floor($ageInSeconds / (365 * 24 * 60 * 60)); $months = floor(($ageInSeconds - ($years * 365 * 24 * 60 * 60)) / (30 * 24 * 60 * 60)); $days = floor(($ageInSeconds - ($years * 365 * 24 * 60 * 60) - ($months * 30 * 24 * 60 * 60)) / (24 * 60 * 60)); echo "You are $years years, $months months, and $days days old.";
In this example, we calculate a person’s age based on their birthdate and the current date.
3.3. Handling Time Zones
When working with dates and times in a global application, it’s essential to consider time zones. strtotime() operates in the default time zone set in your PHP configuration. However, you can change the time zone using date_default_timezone_set() to ensure accurate date and time calculations.
Example 7: Changing Time Zones
php date_default_timezone_set("America/New_York"); $timestamp = strtotime("2023-09-30 12:00:00"); $formattedDate = date("F j, Y h:i A T", $timestamp); echo $formattedDate; // Output: September 30, 2023 12:00 PM EDT
In this example, we change the default time zone to “America/New_York” before parsing and formatting a timestamp. This ensures that the date and time are displayed correctly in the Eastern Time Zone.
4. Handling Invalid Date Strings
While strtotime() is powerful, it may not always handle all date strings gracefully. It returns false when it cannot parse a date string. To handle invalid date strings, you can use conditional statements to check for errors.
Example 8: Handling Invalid Dates
php $dateString = "invalid_date_string"; $timestamp = strtotime($dateString); if ($timestamp === false) { echo "Invalid date string."; } else { echo date("F j, Y", $timestamp); }
In this example, we check if strtotime() returns false and handle invalid date strings accordingly.
Conclusion
PHP’s strtotime() function is a versatile tool for working with dates and times in your web applications. From parsing date strings to performing complex calculations, it offers a wide range of capabilities. By understanding its syntax and advanced techniques, you can efficiently manipulate dates and times to meet your application’s needs.
In this comprehensive guide, we’ve covered the basics of strtotime(), explored relative date formats, demonstrated advanced usage scenarios, and discussed handling time zones and invalid date strings. Armed with this knowledge, you can confidently leverage strtotime() in your PHP projects to handle date and time-related tasks effectively. So go ahead, explore its possibilities, and make your date and time handling code more efficient and powerful. Happy coding!
Table of Contents
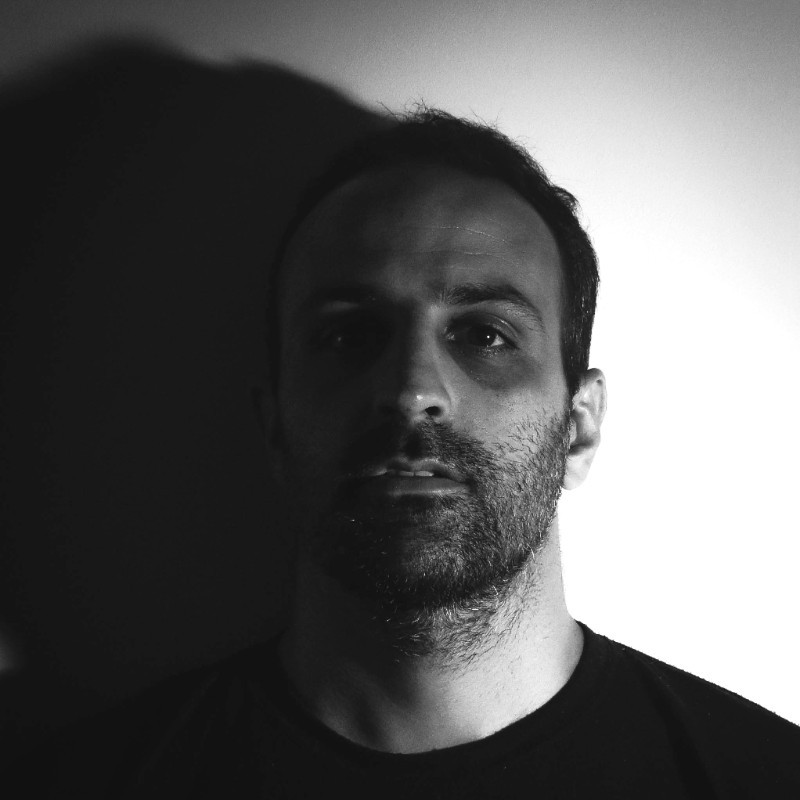
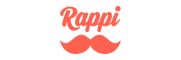