The Fundamentals of PHP’s trim() Function
In the realm of web development, PHP stands as one of the most widely used scripting languages. Its versatility and ease of use make it a preferred choice for developers building dynamic websites and web applications. One indispensable feature in PHP’s arsenal of string manipulation functions is trim(). This unassuming yet powerful function plays a crucial role in sanitizing and preparing user inputs, enhancing data reliability, and simplifying data handling tasks. In this article, we delve into the fundamentals of PHP’s trim() function, exploring its applications, variations, and best practices.
1. Understanding the Basics
1.1. What is trim()?
At its core, the trim() function is designed to remove excess whitespace, as well as control characters, from the beginning and end of a string. This seemingly simple task holds immense value when it comes to data validation and presentation. The ability to eliminate unintended spaces or invisible characters can prevent unexpected issues and maintain the integrity of your data.
1.2. Syntax and Usage
The syntax of the trim() function is straightforward:
php trim(string $string, string $character_mask = " \t\n\rphp trim(string $string, string $character_mask = " \t\n\r\0\x0B"): string\x0B"): string
Here, $string represents the input string you want to manipulate, and $character_mask is an optional parameter that allows you to specify a custom list of characters you wish to remove. By default, the function removes standard whitespace characters including space, tab, newline, carriage return, null byte, and vertical tab.
Let’s take a look at a simple example:
php $input = " Hello, trim me! "; $trimmed = trim($input); echo "Original: '$input' <br>"; echo "Trimmed: '$trimmed'";
In this case, the output would be:
vbnet Original: ' Hello, trim me! ' Trimmed: 'Hello, trim me!'
2. Practical Applications
The trim() function finds application in various scenarios, such as:
2.1. Form Input Processing
When users submit data through forms on your website, it’s common for extra spaces to inadvertently find their way into the input fields. By utilizing trim(), you can eliminate these spaces before further processing, ensuring accurate and consistent data storage.
php $username = trim($_POST['username']); $password = trim($_POST['password']);
2.2. Data Validation
Before validating user input or comparing strings, using trim() helps you remove extraneous characters that could otherwise lead to false negatives or positives. This is particularly crucial for fields like email addresses or usernames, where spaces can easily go unnoticed.
php $submittedEmail = trim($_POST['email']); $storedEmail = "user@example.com"; if ($submittedEmail === $storedEmail) { // Perform further actions }
2.3. Cleaning User-Generated Content
If your website incorporates user-generated content, such as comments or forum posts, the trim() function can be employed to clean up and normalize the text. This ensures a consistent look and feel throughout your platform.
php $userComment = trim($_POST['comment']); // Store the cleaned comment in the database
3. Exploring Advanced Functionality
3.1. Custom Character Masking
While the default character mask in trim() is useful in most cases, you might encounter situations where you need to remove specific characters beyond the defaults. This is where the optional $character_mask parameter comes into play.
For example, if you want to remove all occurrences of the dot (.) character from the beginning and end of a string, you can do so like this:
php $input = "...Hello, trim me!..."; $trimmed = trim($input, '.');
In this instance, the resulting value of $trimmed would be “Hello, trim me!”.
3.2. Handling Unicode Whitespace
It’s important to note that the default behavior of trim() only handles ASCII whitespace characters. If your application involves languages or scripts that use Unicode whitespace characters, such as non-breaking spaces, you should extend the function to handle them appropriately.
php function unicodeTrim($string) { return preg_replace('/^\p{Z}+|\p{Z}+$/u', '', $string); } $input = " Hello, trim me! "; $trimmed = unicodeTrim($input);
In this example, the unicodeTrim() function employs a regular expression with Unicode property escapes to remove Unicode whitespace characters from the beginning and end of the input string.
4. Best Practices for Using trim()
To maximize the benefits of the trim() function, keep these best practices in mind:
4.1. Contextual Usage
Consider the context in which you’re using trim(). Different scenarios might require different character masks or variations of the function to suit your specific needs.
4.2. Input Sanitization
Always sanitize user input before processing it. While trim() helps remove leading and trailing spaces, combining it with other filtering and validation techniques enhances security and data integrity.
4.3. Performance Considerations
While trim() is highly efficient, excessive usage on large datasets could impact performance. Use it judiciously and explore alternatives if required.
4.4. Consistent Data Storage
Use trim() before storing data in databases. This ensures consistency and prevents data duplication caused by variations in leading or trailing spaces.
Conclusion
In the world of PHP programming, mastering the trim() function is a fundamental skill that can significantly improve the reliability and maintainability of your code. By skillfully implementing this function, you can effortlessly manage whitespace and control characters, leading to better data validation, enhanced user experiences, and streamlined data manipulation.
Whether you’re processing form submissions, validating user inputs, or cleaning up user-generated content, the trim() function stands as a powerful ally in your quest for cleaner, more efficient code. With its intuitive syntax and versatile capabilities, trim() continues to be an indispensable tool for PHP developers worldwide.
Table of Contents
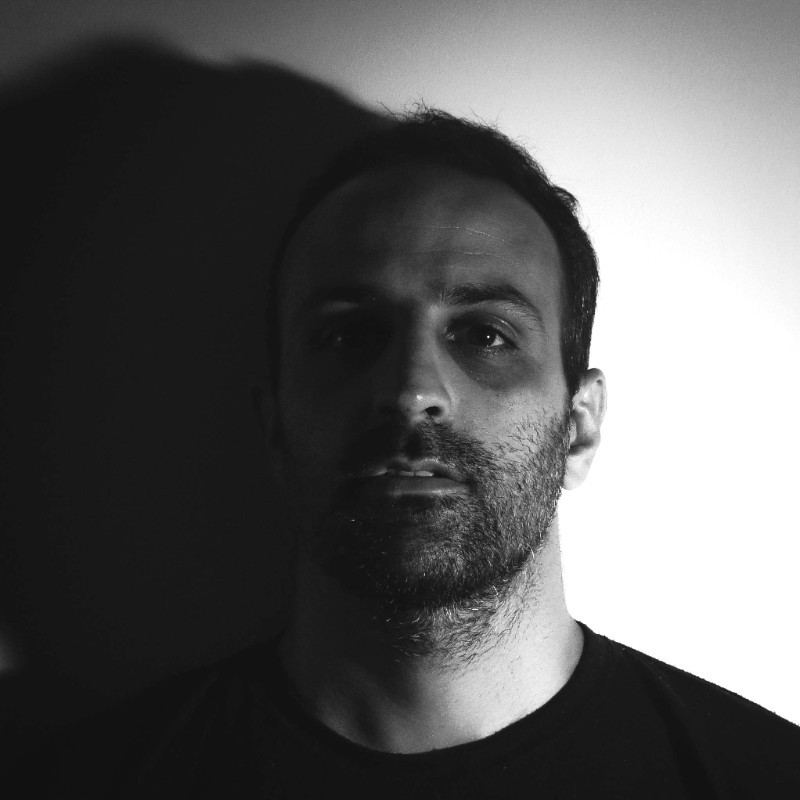
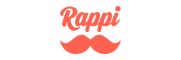