Unpacking PHP’s unpack() Function
PHP is a versatile language that can handle various data manipulation tasks, including binary data. If you’ve ever worked with binary data in PHP, you might have come across the unpack() function. In this comprehensive guide, we’ll delve into the intricacies of PHP’s unpack() function, exploring its syntax, use cases, and practical examples.
Table of Contents
1. Understanding Binary Data
Before we dive into the unpack() function, it’s essential to understand what binary data is. In computing, binary data consists of sequences of 0s and 1s, representing various types of information, such as numbers, text, images, and more. Unlike human-readable text, binary data is encoded in a way that’s efficient for computers to process.
Binary data is commonly encountered in various scenarios, including file formats (like images and videos), network communication protocols, and data storage. To work with binary data effectively in PHP, you need the right tools, and one such tool is the unpack() function.
2. What is unpack()?
The unpack() function in PHP is used to interpret binary data according to a specified format and convert it into a more usable form. It allows you to extract values from binary data, which may be packed using the pack() function or obtained from external sources like files or network sockets.
2.1. Syntax of unpack()
Here’s the basic syntax of the unpack() function:
php array unpack(string $format, string $data)
- $format: This parameter specifies the format of the binary data. It defines how the binary data is structured and how the values should be extracted. The format is a string that consists of format codes and optionally, repeat counts and name labels for extracted values.
- $data: This parameter contains the binary data that you want to unpack.
2.2. Format Codes
The format codes define how the binary data should be interpreted and extracted. Each format code corresponds to a specific data type. Here are some common format codes:
- a: NUL-padded string
- A: SPACE-padded string
- c: Signed char
- C: Unsigned char
- s: Signed short (always 16 bit, machine byte order)
- S: Unsigned short (always 16 bit, machine byte order)
- n: Unsigned short (always 16 bit, big endian byte order)
- v: Unsigned short (always 16 bit, little endian byte order)
- i: Signed integer (machine byte order)
- I: Unsigned integer (machine byte order)
- l: Signed long (always 32 bit, machine byte order)
- L: Unsigned long (always 32 bit, machine byte order)
- N: Unsigned long (always 32 bit, big endian byte order)
- V: Unsigned long (always 32 bit, little endian byte order)
- f: Float (machine byte order)
- d: Double (machine byte order)
- …and more.
The format code specifies the data type and the number of bytes used to represent it. For example, c represents a signed char, which is a 1-byte signed integer.
2.3. Repeat Counts and Name Labels
You can modify the format codes with repeat counts to extract multiple values of the same type in a single unpack() call. Additionally, you can assign names to extracted values using the @ symbol followed by a label.
Here’s an example that demonstrates the use of repeat counts and name labels:
php $data = "\x01\x02\x03\x04\x05"; $result = unpack("Cfirst/Csecond/Cthird", $data); print_r($result);
In this example, we extract three unsigned chars from the binary data, and we’ve assigned names to them: first, second, and third. The resulting array will contain these named values.
2.4. Return Value
The unpack() function returns an associative array containing the extracted values. The keys of the array correspond to the names assigned to the extracted values (if any), and the values are the extracted data.
3. Practical Use Cases
Now that we understand the basics of the unpack() function, let’s explore some practical use cases where it can be incredibly helpful.
3.1. Parsing Binary File Formats
Many file formats, such as image formats (e.g., BMP, GIF, PNG) and document formats (e.g., PDF), store their data in binary format. By using the unpack() function, you can parse these files and extract relevant information. For instance, you can extract image dimensions, color information, or metadata from image files.
php // Example: Parsing a BMP image header $bmpData = file_get_contents('image.bmp'); $header = unpack('vtype/Vsize/vreserved1/vreserved2/Voffset', $bmpData); print_r($header);
In this example, we unpack the header of a BMP image file and extract various properties, such as the file type, size, and offset.
3.2. Handling Network Protocols
When working with network communication, data is often sent and received in binary form. The unpack() function can be used to decode incoming data and extract meaningful information. For instance, you can use it to parse network packets and extract specific fields like IP addresses, port numbers, or protocol headers.
php // Example: Parsing an IPv4 header $packet = socket_read($socket, 20); $header = unpack('Cversion/Chlen/nid/ndf/Cttl/Cprotocol/nchecksum/Nsrc_ip/Ndest_ip', $packet); print_r($header);
Here, we’re reading an IPv4 packet from a network socket and extracting various fields from the header.
3.3. Working with Binary Sensors and Devices
In embedded systems and IoT applications, sensors and devices often communicate using binary data. By using unpack(), you can interpret data from sensors and make decisions based on the extracted information. For instance, you can read data from a temperature sensor and take action based on the temperature value.
php // Example: Reading data from a temperature sensor $data = readSensorData(); $result = unpack('ftemperature', $data); if ($result['temperature'] > 30.0) { // Take action based on high temperature // ... }
In this scenario, we’re reading data from a temperature sensor and checking if the temperature exceeds a certain threshold.
3.4. Binary Data Manipulation
Sometimes, you might need to manipulate binary data directly, such as bitwise operations, changing specific bits, or converting between different data representations. The unpack() function can help you access and modify individual components of binary data easily.
php // Example: Modifying individual bits of a binary value $data = "\x05"; // Binary: 00000101 $values = unpack('Cb1/Cb2', $data); $values['b1'] |= 0x04; // Set the third bit (binary: 00000101 | 00000100 = 00000101) $newData = pack('C*', $values['b1'], $values['b2']); echo bin2hex($newData); // Output: 05
In this example, we unpack a single byte into two bits and then modify one of the bits before packing it back into binary form.
4. Common Mistakes and Pitfalls
While the unpack() function is a powerful tool for working with binary data, it’s essential to be aware of common mistakes and pitfalls to avoid unexpected behavior in your code:
4.1. Incorrect Format Codes
Using incorrect format codes can lead to misinterpretation of binary data. Ensure that the format codes you use match the actual data type and structure of the binary data.
4.2. Endianness Issues
Endianness refers to the byte order used to represent multi-byte data types. Make sure you use the correct endianness when unpacking data, especially when dealing with network protocols or file formats that have specific byte order requirements.
4.3. Incomplete Data
If the binary data is truncated or incomplete, attempting to unpack it can result in errors or unexpected values. Always verify the integrity of the binary data before unpacking.
4.4. Overflow
Be cautious when unpacking large integers. PHP uses signed integers, so unpacking a large unsigned integer may result in overflow and unexpected values.
Conclusion
PHP’s unpack() function is a valuable tool for working with binary data. It allows you to interpret binary data according to a specified format, making it easier to extract and manipulate meaningful information. Whether you’re parsing binary file formats, handling network protocols, working with sensors, or performing low-level binary data operations, the unpack() function can streamline your development process.
By understanding the syntax, format codes, and practical use cases of unpack(), you can leverage its power to tackle a wide range of binary data challenges in your PHP projects. So, the next time you encounter binary data, remember to unpack it with confidence using PHP’s unpack() function.
Table of Contents
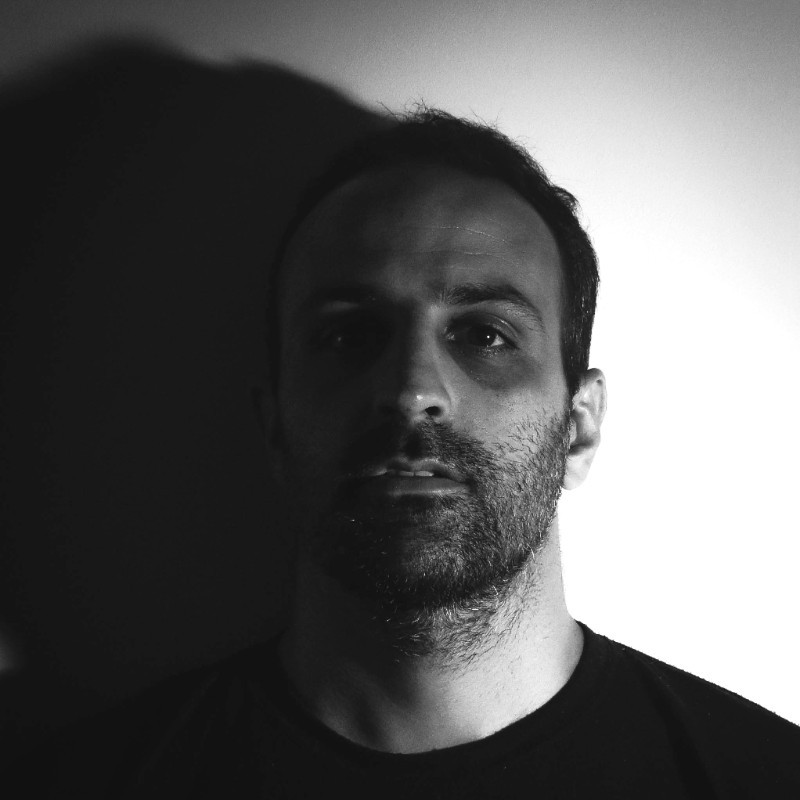
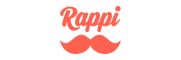