Swift for Beginners: A Comprehensive Guide to Mastering iOS Programming Language
Swift, developed by Apple Inc. in 2014, has become an essential language for any iOS, macOS, watchOS, or tvOS developer. If you’re looking to hire Swift iOS developers, a solid understanding of this language is crucial. Its efficient syntax, coupled with its safety features, makes Swift a modern, powerful programming language that is easy to learn and fun to use.
This blog will guide you, whether you’re a developer or a recruiter, on your journey to mastering Swift. Let’s dive in!
Understanding Swift Basics
Swift, like most programming languages, has variables, constants, and data types. Here’s a quick introduction:
```swift var myVariable = "Hello, Swift" // Variable declaration let myConstant = 10 // Constant declaration
In the above example, `myVariable` is a variable, and `myConstant` is a constant. The `var` keyword is used for declaring variables, and `let` is used for constants. Swift also provides type inference, which means you don’t have to specify the type of every declared variable or constant explicitly. Understanding these fundamental principles can aid those aiming to hire Swift iOS developers, as they represent the basic skill set such a developer should possess.
Data types in Swift are quite similar to other languages:
```swift let integer: Int = 10 let double: Double = 10.0 let float: Float = 10.0 let bool: Bool = true let string: String = "Hello, Swift"
These are examples of Swift’s basic types: Int for integers, Double and Float for floating-point numbers, Bool for boolean values, and String for textual data.
Functions and Control Flow
Functions in Swift are declared using the `func` keyword, followed by the function name and parameters inside parentheses. The function’s return type is denoted after the ‘->’ symbol. For example:
```swift func greet(person: String) -> String { let greeting = "Hello, \(person)!" return greeting } print(greet(person: "Anna")) // Prints "Hello, Anna!"
Swift has various control flow statements, such as `if`, `switch`, `for-in`, `while`, and `repeat-while`. Here’s an example using `if` and `for-in`:
```swift let numbers = [1, 2, 3, 4, 5] for number in numbers { if number % 2 == 0 { print("\(number) is even") } else { print("\(number) is odd") } }
Swift Collections
Swift provides several collection types, like arrays and dictionaries. Arrays are ordered collections of values, and dictionaries are unordered collections of key-value pairs.
```swift // Array var fruits = ["Apple", "Banana", "Cherry"] fruits.append("Dragonfruit") // Adding a new element // Dictionary var bookAuthors: [String: String] = [ "1984": "George Orwell", "To Kill a Mockingbird": "Harper Lee" ] bookAuthors["The Great Gatsby"] = "F. Scott Fitzgerald" // Adding a new key-value pair
Object-Oriented Programming in Swift
Swift supports object-oriented programming. Here’s a simple class definition:
```swift class Animal { var name: String init(name: String) { self.name = name } func describe() { print("Animal's name is \(name)") } } let dog = Animal(name: "Rex") dog.describe() // Prints "Animal's name is Rex"
In this example, `Animal` is a class with a property `name` and a function `describe()`. The `init(name: String)` is a special function called an initializer, used to create instances of the class.
Error Handling
Swift provides robust support for throwing, catching, propagating, and manipulating recoverable errors at runtime.
```swift enum PrinterError: Error { case outOfPaper case noToner case onFire } func send(job: Int, toPrinter printerName: String) throws -> String { if printerName == "Never Has Toner" { throw PrinterError.noToner } return "Job sent" } do { let printerResponse = try send(job: 1040, toPrinter: "Never Has Toner") print(printerResponse) } catch { print(error) }
In this example, `PrinterError` is an enumeration that conforms to the `Error` protocol and represents the type of error that the `send(job:toPrinter:)` function can throw. If an error is thrown, the code execution transfers to the `catch` block.
Swift’s Protocol-Oriented Programming
Swift is known for its protocol-oriented programming model. Protocols define a blueprint of methods, properties, and other requirements suitable for a particular task.
```swift protocol Flyable { var canFly: Bool { get set } func fly() } class Bird: Flyable { var canFly = true func fly() { if canFly { print("The bird is flying.") } else { print("This bird can't fly.") } } }
In this example, `Flyable` is a protocol that requires a `canFly` property and a `fly()` function. The `Bird` class conforms to this protocol by implementing these requirements.
Conclusion
Swift is a powerful and intuitive programming language that provides safety, performance, and a playful syntax. It integrates well with existing Objective-C code and also with Apple’s robust frameworks. This makes it a highly desirable skill for those who wish to hire Swift iOS developers. The concepts outlined above should provide a solid foundation for developers honing their Swift skills, or for recruiters aiming to understand the competencies of potential hires.
Practice is key – start building iOS apps today! If you’re a company looking to hire Swift iOS developers, an understanding of these principles will assist you in making informed recruitment decisions.
With Swift’s continuous evolution, it is crucial for developers and those hiring them to keep up with the new features and enhancements introduced with each release. Happy Swifting!
Table of Contents
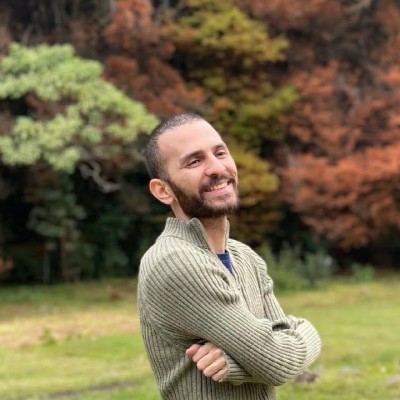
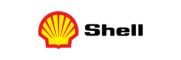