Integrating Machine Learning in Swift: Enhancing iOS App Intelligence
In recent years, machine learning has emerged as a powerful tool for enhancing app intelligence and delivering personalized user experiences. iOS developers can leverage the capabilities of machine learning to create intelligent apps that adapt to user behavior and provide valuable insights. In this blog post, we will explore how to integrate machine learning in Swift using Core ML, Apple’s machine learning framework. We will delve into the benefits of incorporating machine learning into iOS apps, learn about Core ML, and provide practical examples and code snippets to get you started on your journey to building intelligent apps.
The Power of Machine Learning in iOS Apps
1. Personalization and User Experience:
Machine learning enables iOS apps to learn from user behavior and preferences, allowing for personalized experiences. By analyzing user interactions and patterns, apps can adapt their content, recommendations, and user interfaces to cater to individual needs. For example, a news app can prioritize articles based on the user’s reading habits or recommend products based on their browsing history.
2. Predictive Analysis and Recommendations:
With machine learning, iOS apps can predict user preferences and make intelligent recommendations. By leveraging historical data, machine learning algorithms can identify patterns and make accurate predictions. For instance, an e-commerce app can recommend products based on the user’s previous purchases or suggest movies to watch based on their viewing history.
3. Natural Language Processing:
Machine learning techniques such as natural language processing (NLP) enable iOS apps to understand and interpret human language. This opens up possibilities for voice assistants, chatbots, and language translation apps. NLP allows for sentiment analysis, entity recognition, and language generation, enabling apps to respond intelligently to user input.
Introducing Core ML
1. What is Core ML?
Core ML is Apple’s machine learning framework that allows developers to integrate machine learning models into iOS, macOS, watchOS, and tvOS apps. It provides a seamless way to use pre-trained machine learning models and perform on-device inference, making it fast, secure, and privacy-friendly.
2. Supported Models and Frameworks:
Core ML supports a wide range of machine learning models and frameworks, including neural networks, tree ensembles, support vector machines, and more. You can leverage popular machine learning libraries such as TensorFlow and Keras to train and convert models into the Core ML format.
3. Core ML Tools and Integration:
To work with Core ML, you need Xcode, Apple’s integrated development environment for iOS app development. Xcode provides tools for importing, visualizing, and testing Core ML models. Additionally, Core ML seamlessly integrates with other iOS frameworks like Vision for computer vision tasks and Natural Language for NLP.
4. Core ML Model Format:
Core ML models are typically packaged in the .mlmodel format. These models are optimized for on-device performance and can be easily integrated into your Xcode project. You can either train your own models using popular machine learning frameworks or leverage pre-trained models available in Apple’s model gallery.
Getting Started with Core ML
1. Installing Core ML Tools:
To start integrating machine learning in Swift, ensure that you have the latest version of Xcode installed on your Mac. Xcode provides all the necessary tools and libraries to work with Core ML.
2. Preparing the Data and Model:
Before integrating a machine learning model into your app, you need to prepare the data and train the model. This involves collecting and preprocessing the relevant data, choosing an appropriate machine learning algorithm, and training the model using a suitable framework like TensorFlow or PyTorch.
3. Importing the Model into Xcode:
Once you have a trained model, you can import it into your Xcode project. Xcode provides a visual interface for importing Core ML models, which generates Swift code to access the model’s input and output. This allows you to seamlessly integrate the model into your app’s logic.
4. Making Predictions with Core ML:
With the model imported, you can start making predictions in your app. Core ML provides a straightforward API to feed input data to the model and receive predictions as output. This can be done synchronously or asynchronously, depending on the requirements of your app.
Enhancing iOS App Intelligence with Core ML
1. Image Recognition and Classification:
With Core ML, you can implement image recognition and classification in your iOS app. By leveraging pre-trained models like MobileNet or Inceptionv3, you can identify objects, scenes, or even perform facial recognition. This opens up possibilities for augmented reality, object tracking, and photo analysis apps.
2. Sentiment Analysis:
Sentiment analysis allows you to determine the sentiment or emotion behind a piece of text. By utilizing natural language processing and pre-trained models, you can analyze social media posts, customer reviews, or user feedback in real-time. This enables you to gain insights into user opinions and tailor your app accordingly.
3. Object Detection and Tracking:
Using the Vision framework in conjunction with Core ML, you can build iOS apps that perform real-time object detection and tracking. This can be useful for creating applications like smart cameras, augmented reality games, or security systems that can recognize and track objects or people.
4. Speech Recognition and Translation:
Core ML, in combination with the Speech framework, allows you to integrate speech recognition and translation capabilities into your iOS app. This enables you to create voice-controlled interfaces, real-time transcription apps, or language translation tools, all directly on the user’s device.
Code Samples and Practical Examples
1. Image Classification with Core ML:
swift import CoreML import Vision func classifyImage(image: UIImage) { guard let model = try? VNCoreMLModel(for: ResNet50().model) else { fatalError("Failed to load Core ML model.") } let request = VNCoreMLRequest(model: model) { (request, error) in guard let results = request.results as? [VNClassificationObservation], let topResult = results.first else { fatalError("Failed to classify image.") } print("Image classification result: \(topResult.identifier) (\(topResult.confidence))") } let handler = VNImageRequestHandler(cgImage: image.cgImage!) do { try handler.perform([request]) } catch { print("Failed to perform image classification.") } }
2. Sentiment Analysis using Natural Language Processing:
swift import NaturalLanguage func analyzeSentiment(text: String) { let tagger = NLTagger(tagSchemes: [.sentimentScore]) tagger.string = text guard let sentiment = tagger.tag(at: text.startIndex, unit: .paragraph, scheme: .sentimentScore).0 else { print("Failed to analyze sentiment.") return } print("Sentiment analysis result: \(sentiment.rawValue)") }
3. Real-Time Object Detection with Vision Framework:
swift import Vision func detectObjects(in image: UIImage) { guard let model = try? VNCoreMLModel(for: YOLOv3().model) else { fatalError("Failed to load Core ML model.") } let request = VNCoreMLRequest(model: model) { (request, error) in guard let results = request.results as? [VNRecognizedObjectObservation] else { fatalError("Failed to detect objects.") } for result in results { print("Detected object: \(result.labels.first?.identifier ?? "") (\(result.confidence))") } } let handler = VNImageRequestHandler(cgImage: image.cgImage!, options: [:]) do { try handler.perform([request]) } catch { print("Failed to perform object detection.") } }
4. Speech Recognition and Translation with Speech Framework:
swift import Speech func recognizeSpeech() { let recognizer = SFSpeechRecognizer(locale: Locale(identifier: "en-US")) SFSpeechRecognizer.requestAuthorization { (status) in guard status == .authorized else { print("Speech recognition authorization denied.") return } let request = SFSpeechAudioBufferRecognitionRequest() let audioEngine = AVAudioEngine() guard let inputNode = audioEngine.inputNode else { fatalError("Audio engine has no input node.") } let recordingFormat = inputNode.outputFormat(forBus: 0) inputNode.installTap(onBus: 0, bufferSize: 1024, format: recordingFormat) { (buffer, _) in request.append(buffer) } audioEngine.prepare() do { try audioEngine.start() } catch { print("Failed to start audio engine.") return } recognizer?.recognitionTask(with: request) { (result, error) in if let transcription = result?.bestTranscription { print("Speech recognition result: \(transcription.formattedString)") translateText(transcription.formattedString) } else if let error = error { print("Speech recognition error: \(error.localizedDescription)") } } } } func translateText(_ text: String) { let translator = Translator() translator.translate(text: text, sourceLanguage: "en", targetLanguage: "fr") { (result, error) in if let translatedText = result { print("Translation result: \(translatedText)") } else if let error = error { print("Translation error: \(error.localizedDescription)") } } }
Conclusion
Integrating machine learning in Swift using Core ML opens up a world of possibilities for enhancing iOS app intelligence. By leveraging Core ML’s capabilities, you can create intelligent apps that adapt to user behavior, provide personalized recommendations, and perform complex tasks like image recognition, sentiment analysis, object detection, and speech recognition. With the code samples and practical examples provided in this blog post, you are well-equipped to embark on your journey to building intelligent iOS apps that deliver exceptional user experiences. So go ahead, harness the power of machine learning in Swift, and take your iOS app development to new heights.
Table of Contents
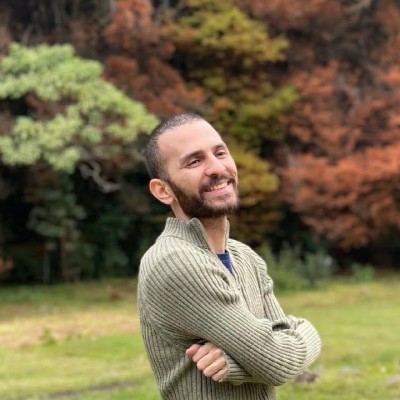
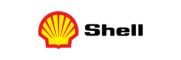