Empowering iOS Development: A Deep Dive into the Swift Standard Library
Every modern programming language boasts a standard library, and Swift is no exception. The Swift Standard Library is a collection of built-in functions, types, and capabilities that provide developers, especially those looking to hire Swift iOS developers, with foundational features to enhance productivity and code efficiency. This library plays a key role in making Swift robust, expressive, and a favorite among not only iOS developers but also those who hire Swift iOS developers.
This blog post will explore the Swift Standard Library, focusing on several of its built-in functions that can significantly streamline iOS development and make the job of a Swift iOS developer more efficient.
Map, Filter, and Reduce
Swift’s `map`, `filter`, and `reduce` functions are a trio of powerful methods that provide a high-level approach to manipulating and iterating over collections.
Map
The `map` function applies a given transformation to each element in a collection. Here’s how you might use it to square every element in an array:
```swift let numbers = [1, 2, 3, 4, 5] let squared = numbers.map { $0 * $0 } print(squared) // Outputs: [1, 4, 9, 16, 25]
Filter
The `filter` function is used to select elements in a collection that satisfy a certain condition. For example, to extract only the even numbers from an array:
```swift let numbers = [1, 2, 3, 4, 5] let evens = numbers.filter { $0 % 2 == 0 } print(evens) // Outputs: [2, 4]
Reduce
The `reduce` function takes a collection of elements and combines them into a single value. Suppose we want to find the sum of all numbers in an array:
```swift let numbers = [1, 2, 3, 4, 5] let sum = numbers.reduce(0, +) print(sum) // Outputs: 15
Enumerate
The `enumerated` function adds indices to the elements of a collection, which can be extremely handy when you need to track the position of an item in a sequence. Here’s an example:
```swift let names = ["Anna", "Eli", "Sophie"] for (index, name) in names.enumerated() { print("Person \(index + 1): \(name)") } // Outputs: // Person 1: Anna // Person 2: Eli // Person 3: Sophie
Sort and Sorted
Swift Standard Library provides two sorting functions, `sort` and `sorted`. The `sorted` function returns a new array that is sorted, whereas `sort` sorts the original array in place. Here’s how you can use them:
```swift var numbers = [5, 3, 2, 1, 4] let sortedNumbers = numbers.sorted() // numbers is still [5, 3, 2, 1, 4] print(sortedNumbers) // Outputs: [1, 2, 3, 4, 5] numbers.sort() // numbers is now [1, 2, 3, 4, 5] print(numbers) // Outputs: [1, 2, 3, 4, 5]
Zip
`Zip` is a fantastic utility that can combine two sequences into a sequence of tuples. For example, if you want to combine two arrays, one containing names and another containing ages:
```swift let names = ["Anna", "Eli", "Sophie"] let ages = [23, 30, 27] let combined = Array(zip(names, ages)) print(combined) // Outputs: [("Anna", 23), ("Eli", 30), ("Sophie", 27)]
Conclusion
Swift’s Standard Library is a treasure trove of functionality. It’s essential for developers, including those looking to hire Swift iOS developers, to familiarize themselves with these built-in functions to make the most of Swift’s expressive, concise, and powerful syntax. These functions not only simplify complex operations but also make your code cleaner, more efficient, and easier to understand, a trait highly appreciated by those who hire Swift iOS developers.
Remember, the key to harnessing the power of the Swift Standard Library is practice. Try to incorporate these built-in functions into your daily coding tasks, and you’ll soon realize just how much they can streamline your iOS development process, making you a valuable asset for anyone looking to hire Swift iOS developers.
So, next time you find yourself writing extra lines of code or creating complex loops, take a step back and revisit the Swift Standard Library. The solution might be simpler than you think. As the Swift language evolves, so too does its library. So stay updated, stay curious, and keep exploring. With the right tools at your disposal, your journey into iOS development can become a swift sail, elevating your profile for those who seek to hire Swift iOS developers.
Table of Contents
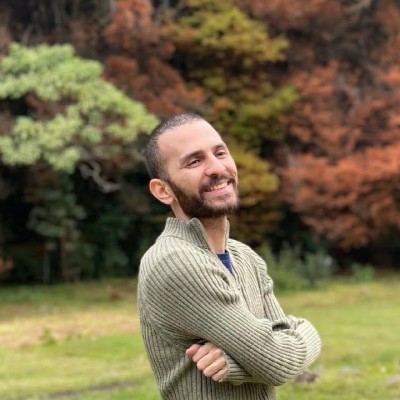
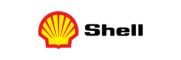