TypeScript and Browser APIs: Interacting with the DOM
In the realm of web development, creating dynamic and interactive user interfaces is a paramount goal. The Document Object Model (DOM) is at the heart of this endeavor, acting as the bridge between the structure of a web page and the programming languages that manipulate it. JavaScript has traditionally been the go-to language for this task, but with the advent of TypeScript, developers now have a powerful tool at their disposal to enhance their interaction with the DOM.
Table of Contents
1. Understanding TypeScript and Its Benefits
Before delving into the specifics of how TypeScript enhances interaction with the DOM, let’s briefly understand what TypeScript is and its benefits. TypeScript is a superset of JavaScript that introduces static typing to the language. This means that you can define the types of variables, function parameters, and return values, which helps catch potential errors during development, ultimately leading to more robust and reliable code.
2. The Power of Strong Typing
One of the standout features of TypeScript is its strong typing system. When it comes to interacting with the DOM, this feature becomes particularly valuable. Traditional JavaScript leaves room for errors due to its loose and dynamic typing. With TypeScript, you can explicitly define the types of elements you’re working with, whether they’re HTML elements, event listeners, or any other aspect of the DOM. This results in fewer runtime errors and better code quality.
Let’s look at an example of how TypeScript’s strong typing makes a difference:
typescript // JavaScript const button = document.querySelector('#myButton'); button.addEventListener('click', event => { // Handle the click event }); // TypeScript const button = document.querySelector('#myButton') as HTMLButtonElement; button.addEventListener('click', (event: Event) => { // Handle the click event });
In this example, TypeScript allows us to explicitly cast the button element to an HTMLButtonElement. This not only provides better autocompletion and documentation but also prevents accidental usage of properties/methods that aren’t available on regular HTML elements.
3. Leveraging Interfaces for Enhanced DOM Interaction
Interfaces are a powerful feature in TypeScript that allow you to define the structure of an object, specifying the names of properties, their types, and whether they’re required or optional. When working with the DOM, interfaces can greatly enhance the clarity and safety of your code.
Let’s say you’re creating a function that initializes a carousel component. Without TypeScript, your function might look like this:
javascript function initializeCarousel(container, options) { // Initialize the carousel }
While this function might work, it lacks clarity regarding the expected types of container and options. Enter TypeScript interfaces:
typescript interface CarouselOptions { autoplay: boolean; interval: number; transition: string; // ... other options } function initializeCarousel(container: HTMLElement, options: CarouselOptions) { // Initialize the carousel }
Now, anyone reading the code knows exactly what types of arguments are expected. Additionally, developers using this function will receive type hints and errors if they don’t adhere to the defined interface.
4. DOM Manipulation Made Safer
DOM manipulation can be error-prone, especially when dealing with complex structures or frequent updates. TypeScript helps mitigate this risk by providing intelligent autocompletion and type checking. When you’re traversing the DOM, modifying elements, or manipulating attributes, TypeScript guides you with relevant suggestions and warns you about potential issues.
Consider the scenario where you want to toggle the visibility of an element. In JavaScript, you might do something like this:
javascript const element = document.getElementById('myElement'); element.style.display = 'none'; // Hide the element
While this works, TypeScript can offer more assistance:
typescript const element = document.getElementById('myElement'); if (element) { element.style.display = 'none'; // Hide the element }
By using TypeScript, you signal to the compiler that you’ve considered the possibility of the element being null or undefined, thus preventing runtime errors.
5. Enhancing Event Handling
Event handling lies at the core of creating interactive web applications. TypeScript brings a layer of clarity and safety to this aspect of web development by allowing you to define the types of event objects and ensuring you’re only handling events relevant to the element in question.
Let’s say you’re handling a form submission event:
typescript const form = document.getElementById('myForm') as HTMLFormElement; form.addEventListener('submit', (event: Event) => { event.preventDefault(); // Handle the form submission });
In this example, TypeScript ensures you’re dealing with the correct event type and provides proper autocompletion for event-related properties and methods.
6. Utilizing Browser APIs with TypeScript
Browser APIs provide various functionalities that extend the capabilities of web applications. With TypeScript, working with these APIs becomes even more efficient and less error-prone.
For instance, if you’re using the Geolocation API to fetch a user’s location, TypeScript can help you handle the asynchronous nature of the API in a structured manner:
typescript navigator.geolocation.getCurrentPosition( (position: GeolocationPosition) => { const latitude = position.coords.latitude; const longitude = position.coords.longitude; // Do something with the coordinates }, (error: GeolocationPositionError) => { // Handle the error } );
TypeScript ensures you handle both success and error cases appropriately, as per the types defined by the Geolocation API.
Conclusion
TypeScript has undoubtedly elevated the way developers interact with the DOM and utilize browser APIs. Its strong typing, coupled with interfaces and intelligent autocompletion, empowers developers to create more efficient, reliable, and maintainable web applications. By minimizing runtime errors and providing clearer documentation, TypeScript streamlines the development process and enhances the overall quality of web development projects. As web applications continue to evolve, embracing TypeScript’s capabilities becomes an essential step toward building a better web. So, whether you’re a seasoned developer or just starting, diving into TypeScript for DOM interaction can undoubtedly transform your web development experience.
Table of Contents
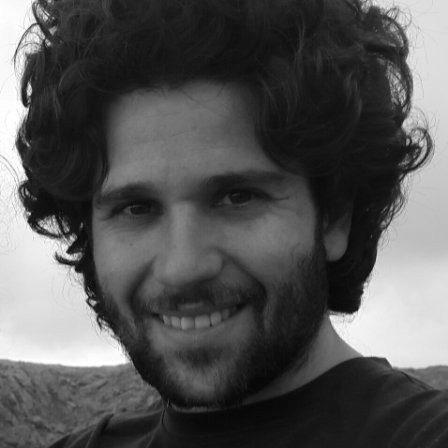
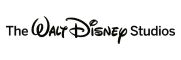