Building Mobile Apps with TypeScript and React Native
In the fast-paced world of mobile app development, choosing the right tools and technologies can make a significant difference in the success of your project. When it comes to building performant and maintainable mobile apps, React Native has emerged as a popular choice. Pairing it with TypeScript, a powerful superset of JavaScript, can further enhance your development experience by providing strong typing and improved code organization. In this guide, we’ll dive into the world of mobile app development using TypeScript and React Native, exploring essential concepts, best practices, and code samples to help you create high-quality applications.
Table of Contents
1. Introduction to TypeScript and React Native
1.1. What is React Native?
React Native is an open-source framework developed by Facebook that allows you to build mobile applications using JavaScript and React. It enables you to create native-like user interfaces for both iOS and Android platforms using a single codebase. This eliminates the need for writing separate code for each platform, saving time and effort in the development process. React Native provides a rich set of pre-built components and allows you to integrate native modules when necessary.
1.2. The Benefits of TypeScript in Mobile App Development
TypeScript is a statically typed superset of JavaScript that adds optional static typing to the language. This brings several advantages to mobile app development with React Native:
- Type Safety: TypeScript allows you to catch type-related errors during development, reducing the chances of runtime errors and enhancing code quality.
- Code Maintainability: With TypeScript, you can better organize your codebase and improve readability through type annotations and interfaces.
- Intelligent Tooling: TypeScript offers advanced autocompletion and code navigation, making the development process more efficient.
2. Setting Up Your Development Environment
2.1. Installing Node.js and npm
Before diving into mobile app development, ensure you have Node.js and npm (Node Package Manager) installed on your system. You can download them from the official Node.js website.
2.2. Creating a New React Native Project
To create a new React Native project, you can use the following command:
bash npx react-native init MyApp
Replace MyApp with the desired name for your project. This command sets up a new project with the basic structure and necessary files.
2.3. Configuring TypeScript for Your Project
To incorporate TypeScript into your React Native project, follow these steps:
Install the required dependencies:
bash npm install --save-dev typescript @types/react @types/react-native @types/react-navigation
Create a tsconfig.json file in the root of your project:
json { "compilerOptions": { "allowJs": true, "allowSyntheticDefaultImports": true, "esModuleInterop": true, "jsx": "react-native", "lib": ["es6"], "moduleResolution": "node", "noEmit": true, "skipLibCheck": true, "resolveJsonModule": true, "strict": true }, "exclude": ["node_modules", "babel.config.js", "metro.config.js", "jest.config.js"] }
This configuration file specifies TypeScript settings and excludes unnecessary files from the compilation process.
3. Building Components with TypeScript and React Native
3.1. Functional Components vs. Class Components
React Native supports two main types of components: functional and class components. Functional components are simpler and easier to test, making them a preferred choice. TypeScript adds type inference and annotations to these components, making the codebase more robust.
Here’s an example of a functional component in TypeScript:
tsx import React from 'react'; import { View, Text, StyleSheet } from 'react-native'; interface Props { title: string; } const MyComponent: React.FC<Props> = ({ title }) => { return ( <View style={styles.container}> <Text>{title}</Text> </View> ); }; const styles = StyleSheet.create({ container: { padding: 16, backgroundColor: 'lightgray', }, }); export default MyComponent;
3.2. Creating Reusable UI Elements
With TypeScript, you can define props interfaces for your components, making it clear what data is expected. This enhances code readability and helps prevent potential bugs. Reusable UI elements become more manageable and self-documenting.
tsx interface ButtonProps { label: string; onPress: () => void; } const Button: React.FC<ButtonProps> = ({ label, onPress }) => { return ( <TouchableOpacity onPress={onPress} style={styles.button}> <Text style={styles.buttonLabel}>{label}</Text> </TouchableOpacity> ); };
3.3. Managing Component State and Props
In React Native development, props and state are core concepts. With TypeScript, you can define the shape of your props and state using interfaces, providing clarity and avoiding mistakes.
tsx interface CounterProps { initialValue: number; } interface CounterState { count: number; } class Counter extends React.Component<CounterProps, CounterState> { constructor(props: CounterProps) { super(props); this.state = { count: props.initialValue, }; } render() { return ( <View> <Text>Count: {this.state.count}</Text> </View> ); } }
4. Navigation and Routing
4.1. Navigating Between Screens
React Native apps often require navigation between different screens. React Navigation, a popular library, simplifies this process. Combining React Navigation with TypeScript enhances the navigation flow with strong type checking.
tsx // Define your screens type RootStackParamList = { Home: undefined; Profile: { userId: string }; }; const App: React.FC = () => { return ( <NavigationContainer> <Stack.Navigator> <Stack.Screen name="Home" component={HomeScreen} /> <Stack.Screen name="Profile" component={ProfileScreen} /> </Stack.Navigator> </NavigationContainer> ); };
5. Working with APIs and Data
5.1. Making API Requests with Axios
When working with APIs in a React Native app, TypeScript enhances your code by providing clear data types for API responses and error handling.
tsx import axios from 'axios'; interface Post { userId: number; id: number; title: string; body: string; } const fetchPosts = async (): Promise<Post[]> => { try { const response = await axios.get<Post[]>('https://jsonplaceholder.typicode.com/posts'); return response.data; } catch (error) { throw new Error('Error fetching posts'); } };
5.2. State Management with Redux and TypeScript
Redux is a popular state management library that works well with React Native and TypeScript. By defining type-safe actions and reducers, you can ensure better type checking and avoid common mistakes.
tsx // Define actions interface IncrementAction { type: 'INCREMENT'; } interface DecrementAction { type: 'DECREMENT'; } type CounterAction = IncrementAction | DecrementAction; // Create reducer const counterReducer = (state: number = 0, action: CounterAction) => { switch (action.type) { case 'INCREMENT': return state + 1; case 'DECREMENT': return state - 1; default: return state; } };
6. Styling Your App
6.1. Styling Approaches in React Native
React Native offers various styling methods, including inline styles, stylesheets, and third-party libraries. TypeScript complements these approaches by enabling type checking for style props.
tsx interface BoxProps { width: number; height: number; backgroundColor: string; } const Box: React.FC<BoxProps> = ({ width, height, backgroundColor }) => { return ( <View style={{ width, height, backgroundColor }}> {/* Content */} </View> ); };
6.2. Using Styled Components with TypeScript
Styled Components is a popular library that allows you to write CSS-in-JS styles. TypeScript can help you maintain type safety while using Styled Components.
tsx import styled from 'styled-components/native'; interface ButtonProps { primary: boolean; } const StyledButton = styled.TouchableOpacity<ButtonProps>` background-color: ${({ primary }) => (primary ? 'blue' : 'gray')}; padding: 10px 20px; `; const MyComponent: React.FC = () => { return ( <StyledButton primary onPress={() => console.log('Button clicked')}> <ButtonText>Click Me</ButtonText> </StyledButton> ); };
7. Debugging and Testing
7.1. Debugging Your App
React Native offers debugging tools like React DevTools and React Native Debugger. TypeScript aids in spotting type-related issues during development.
7.2. Writing Unit Tests with Jest and React Native Testing Library
TypeScript improves the quality of your unit tests by providing clear type annotations and enhancing code readability.
tsx // Example component const add = (a: number, b: number): number => { return a + b; }; // Test test('adds two numbers correctly', () => { const result = add(2, 3); expect(result).toBe(5); });
8. Deployment and Performance Optimization
8.1. Generating Build Variants
TypeScript and React Native streamline the process of generating separate build variants for different platforms. TypeScript’s static typing contributes to more reliable builds.
8.2. Performance Tips and Tricks
TypeScript encourages well-structured code, which can lead to better performance. Additionally, using libraries like React Native Fast Refresh and Hermes can further optimize your app’s performance.
Conclusion
In conclusion, building mobile apps with TypeScript and React Native offers a powerful combination that enhances the development process, improves code quality, and leads to more maintainable applications. By leveraging the benefits of TypeScript’s type checking and React Native’s cross-platform capabilities, developers can create feature-rich and efficient mobile applications that meet the demands of modern app development. Whether you’re a seasoned developer or just starting, embracing TypeScript and React Native can open up a world of possibilities for your mobile app projects.
Table of Contents
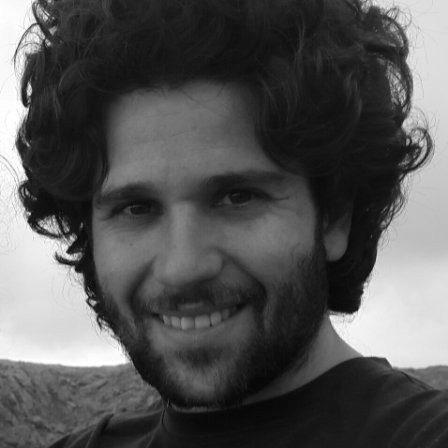
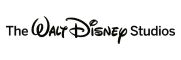