TypeScript and Cloud-Native Development: Going Serverless
Serverless computing has emerged as a game-changer in the world of cloud-native development. With its promise of scalability, cost-efficiency, and reduced operational overhead, serverless architectures are becoming increasingly popular among developers and organizations. When combined with TypeScript, a statically-typed superset of JavaScript, serverless development reaches new heights of productivity and maintainability. In this blog, we’ll explore how TypeScript and serverless go hand-in-hand, providing a powerful combination for modern cloud-native applications.
Table of Contents
1. Why Serverless?
Before delving into the synergy between TypeScript and serverless, let’s briefly understand why serverless computing has gained such traction.
1.1. Scalability
Serverless platforms automatically scale your application based on incoming traffic. There’s no need to provision servers or manage the underlying infrastructure. As demand increases, your application seamlessly scales to handle it, ensuring optimal performance.
1.2. Cost-Efficiency
You only pay for the computing resources your code consumes during execution. In traditional server-based architectures, you’re billed for idle resources, whereas serverless ensures cost savings by charging only for actual usage.
1.3. Reduced Operational Overhead
Serverless providers handle operational tasks like server maintenance, patching, and scaling. Developers can focus on writing code, deploying functions, and delivering value to users, rather than managing infrastructure.
1.4. Speed of Development
Serverless enables rapid development cycles. You can quickly build, deploy, and iterate on functions, reducing time-to-market for new features and applications.
2. TypeScript: The Power of Type Safety
TypeScript, developed by Microsoft, is an open-source, statically-typed superset of JavaScript. It brings the benefits of strong type checking to JavaScript, making code more reliable and maintainable. When combined with serverless development, TypeScript offers several advantages:
2.1. Type Safety
With TypeScript, you catch type-related errors at compile time rather than runtime. This reduces the chances of bugs making it into production, leading to more robust serverless applications.
typescript // TypeScript code sample function addNumbers(a: number, b: number): number { return a + b; } const result = addNumbers(10, "5"); // Type error: Argument of type '"5"' is not assignable to parameter of type 'number'.
2.2. Enhanced IDE Support
TypeScript’s static typing allows for better code completion, auto-refactoring, and error highlighting in modern integrated development environments (IDEs) like Visual Studio Code. This streamlines development and debugging processes.
2.3. Improved Code Maintainability
As serverless applications grow, maintaining code becomes crucial. TypeScript’s type annotations serve as documentation, making it easier for developers to understand and modify the codebase, even as it evolves.
2.4. Easier Collaboration
TypeScript facilitates collaboration among developers by providing clear interfaces and reducing misunderstandings about function signatures and data structures.
3. Getting Started with TypeScript and Serverless
Now that we’ve established the advantages of both serverless and TypeScript let’s dive into creating a serverless application using TypeScript. We’ll use AWS Lambda as our serverless platform of choice, but the principles can be applied to other serverless providers like Azure Functions and Google Cloud Functions.
3.1. Prerequisites
Before you begin, make sure you have the following prerequisites in place:
- An AWS account with proper IAM permissions.
- Node.js and npm installed on your development machine.
- The AWS CLI configured with your AWS credentials.
3.2. Setting Up a TypeScript Project
Let’s start by setting up a new TypeScript project for our serverless application. Open your terminal and run the following commands:
bash # Create a new directory for your project mkdir my-serverless-app # Navigate to the project directory cd my-serverless-app # Initialize a new Node.js project npm init -y # Install TypeScript and AWS SDK npm install typescript aws-sdk --save-dev # Initialize TypeScript configuration npx tsc --init
This sets up a basic TypeScript project with the necessary dependencies and a tsconfig.json file for configuring TypeScript compilation.
3.3. Creating an AWS Lambda Function
In this example, we’ll create a simple AWS Lambda function that calculates the sum of two numbers. Create a new TypeScript file, sum.ts, in your project directory:
typescript // sum.ts import { APIGatewayEvent, APIGatewayProxyResult } from 'aws-lambda'; export const handler = async ( event: APIGatewayEvent ): Promise<APIGatewayProxyResult> => { try { const { num1, num2 } = JSON.parse(event.body || '{}'); const result = num1 + num2; return { statusCode: 200, body: JSON.stringify({ result }), }; } catch (error) { return { statusCode: 500, body: JSON.stringify({ error: 'Internal Server Error' }), }; } };
In this code, we define an AWS Lambda function handler that takes an APIGatewayEvent as input and returns an APIGatewayProxyResult. The function parses the input JSON, calculates the sum, and returns the result as a JSON response.
3.4. Configuring TypeScript
To configure TypeScript to compile your Lambda function, modify your tsconfig.json as follows:
json { "compilerOptions": { // ... "outDir": "./build", "rootDir": "./src" }, // ... }
This configuration tells TypeScript to compile the code from the src directory into the build directory.
4. Building and Packaging
To build your TypeScript code and package it for deployment, create a build script in your package.json:
json "scripts": { "build": "tsc", // ... }
Run the build script using npm run build. This compiles your TypeScript code into JavaScript and places it in the build directory.
5. Deploying to AWS Lambda
To deploy your Lambda function to AWS, you can use the AWS CLI or a tool like the Serverless Framework. Here, we’ll use the AWS CLI for simplicity.
First, create a deployment package by zipping the contents of the build directory:
bash cd build zip -r ../my-serverless-app.zip . cd ..
Next, use the AWS CLI to create a new Lambda function:
bash aws lambda create-function \ --function-name my-serverless-function \ --runtime nodejs14.x \ --handler sum.handler \ --role arn:aws:iam::123456789012:role/your-execution-role \ --zip-file fileb://my-serverless-app.zip
Replace my-serverless-function with your desired function name and provide the appropriate IAM role ARN for execution. This command creates a new Lambda function using the code in your deployment package.
6. Invoking the Lambda Function
You can invoke your Lambda function using the AWS CLI or an HTTP request via API Gateway. Here’s an example using the AWS CLI:
bash aws lambda invoke \ --function-name my-serverless-function \ --payload '{"num1": 5, "num2": 7}' \ response.json
This command invokes your Lambda function with the specified payload and saves the response to response.json. You should see the result of the sum in the output.
7. TypeScript and Serverless Best Practices
As you continue to develop serverless applications with TypeScript, consider the following best practices:
7.1. Keep Functions Small and Focused
Break down your serverless functions into smaller, focused units of work. This promotes reusability and simplifies testing and maintenance.
7.2. Use Environment Variables
Store sensitive information and configuration in environment variables rather than hardcoding them in your code. This enhances security and makes it easier to manage different deployment environments.
7.3. Implement Error Handling
Handle errors gracefully in your serverless functions. Use TypeScript’s type system to catch and handle exceptions effectively.
typescript try { // ... } catch (error) { console.error(`Error: ${error.message}`); return { statusCode: 500, body: JSON.stringify({ error: 'Internal Server Error' }), }; }
7.4. Monitor and Debug
Leverage serverless monitoring and debugging tools to gain insights into your application’s performance and troubleshoot issues.
7.5. Optimize Cold Starts
Cold starts can impact the latency of your serverless functions. Implement strategies like provisioned concurrency to reduce cold start times.
Conclusion
TypeScript and serverless computing are a dynamic duo in cloud-native development. TypeScript’s type safety, enhanced development experience, and maintainability complement the scalability, cost-efficiency, and reduced operational overhead of serverless architectures. By harnessing the power of TypeScript and serverless, you can build robust and efficient cloud-native applications that are well-suited for modern, agile development practices.
As you explore TypeScript and serverless further, remember to follow best practices, stay up-to-date with the latest tools and technologies, and continue learning to make the most of this powerful combination in your cloud-native development journey. Happy coding!
Table of Contents
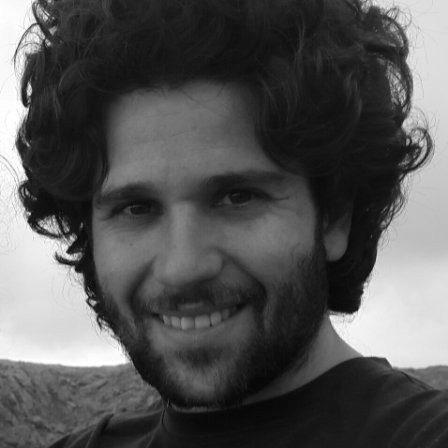
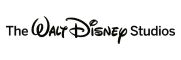