Creating Desktop Applications with Electron and TypeScript
In the modern software development landscape, building desktop applications that run seamlessly on various operating systems is a challenging task. Fortunately, technologies like Electron and TypeScript have revolutionized the way developers approach this challenge. In this guide, we will delve into the world of creating desktop applications using Electron and TypeScript. By the end of this tutorial, you’ll have a solid understanding of the process and be ready to develop your own cross-platform desktop applications.
Table of Contents
1. Introduction to Electron and TypeScript
1.1. What is Electron?
Electron is an open-source framework that enables developers to build cross-platform desktop applications using web technologies such as HTML, CSS, and JavaScript. It achieves this by combining the Chromium rendering engine and the Node.js runtime, allowing developers to create desktop applications that look and feel like native applications on Windows, macOS, and Linux.
1.2. The Advantages of TypeScript
TypeScript, on the other hand, is a superset of JavaScript that introduces static typing and other advanced features to the language. By utilizing TypeScript in your Electron projects, you gain benefits such as improved code quality, better tooling support, and enhanced maintainability. TypeScript enables you to catch potential errors during development and enjoy code completion and better documentation, resulting in a smoother development experience.
2. Setting Up Your Development Environment
Before diving into creating an Electron application with TypeScript, you need to set up your development environment.
2.1. Installing Node.js and npm
To get started, ensure you have Node.js and npm (Node Package Manager) installed on your system. You can download and install them from the official Node.js website. After installation, you can verify if Node.js and npm are correctly set up by running the following commands in your terminal:
bash node -v npm -v
These commands should display the installed Node.js and npm versions, confirming that the installation was successful.
2.2. Initializing a New Project
With Node.js and npm in place, you can now initialize a new project. Create a new directory for your project and navigate to it using the terminal. Run the following command to initialize a new npm project:
bash npm init
Follow the prompts to set up your project’s details, and you’ll end up with a package.json file in your project directory.
3. Creating Your First Electron Application
With your project set up, it’s time to start building your first Electron application.
3.1. Project Structure
A typical Electron application has a main process and a renderer process. The main process manages the lifecycle of the application, interacts with the operating system, and creates the application window. The renderer process handles the UI and runs within the application window.
Create the necessary project structure with the following directories and files:
markdown - your-app/ - index.html (renderer process) - main.js (main process) - package.json
In your package.json file, add the following configuration:
json { "name": "your-electron-app", "version": "1.0.0", "main": "main.js", "scripts": { "start": "electron ." } }
3.2. Main Process and Renderer Process
In the main.js file, you define the main process. This process initializes the Electron application and creates a window. Here’s a basic example:
javascript const { app, BrowserWindow } = require('electron'); function createWindow() { const win = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true } }); win.loadFile('index.html'); } app.whenReady().then(() => { createWindow(); app.on('activate', function () { if (BrowserWindow.getAllWindows().length === 0) createWindow(); }); }); app.on('window-all-closed', function () { if (process.platform !== 'darwin') app.quit(); });
The index.html file represents the renderer process, containing the user interface of your application. You can start with a simple HTML structure and gradually build upon it.
4. Building a User Interface with HTML and CSS
The user interface of your Electron application is built using HTML and styled with CSS, just like building a website.
4.1. HTML5 and CSS3 Fundamentals
Write your HTML code within the index.html file. For instance, to create a basic interface with a title and a button:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Your Electron App</title> <link rel="stylesheet" href="styles.css"> </head> <body> <h1>Welcome to Your Electron App</h1> <button id="clickButton">Click Me</button> <script src="renderer.js"></script> </body> </html>
In the above example, a button is added along with an ID for targeting in JavaScript.
4.2. Integrating UI Components
To make your application visually appealing, create a styles.css file in the same directory as your index.html:
css body { font-family: Arial, sans-serif; text-align: center; } h1 { color: #333; } button { padding: 10px 20px; font-size: 16px; background-color: #007bff; color: #fff; border: none; cursor: pointer; border-radius: 5px; } button:hover { background-color: #0056b3; }
This simple CSS file styles the button and the title, making them visually appealing.
5. Making Your Application Interactive with TypeScript
Now, let’s make your Electron application interactive using TypeScript.
5.1. Introduction to TypeScript
TypeScript allows you to add type annotations to your JavaScript code, which helps catch errors during development and improves code readability. To integrate TypeScript into your project, follow these steps:
- Install TypeScript globally (or locally):
bash npm install -g typescript
2. Create a tsconfig.json file in your project’s root directory to configure TypeScript:
json { "compilerOptions": { "target": "ES6", "outDir": "./dist", "rootDir": "./src", "module": "commonjs", "strict": true } }
3. Create a src directory in your project’s root directory to store your TypeScript files.
5.2. Handling Events
In your TypeScript file, handle the button click event and show an alert:
typescript const clickButton = document.getElementById('clickButton'); clickButton.addEventListener('click', () => { alert('Button Clicked!'); });
Compile the TypeScript code to JavaScript using the following command:
bash tsc
This generates the corresponding JavaScript files in the dist directory based on the TypeScript code in the src directory.
5.3. Communicating Between Processes
Electron allows communication between the main and renderer processes. For instance, you can send messages from the renderer process to the main process and vice versa.
In your main.js:
javascript ipcMain.on('button-click', () => { console.log('Button in Renderer Process Clicked!'); // Add logic or send a message back to the renderer process });
In your TypeScript file:
typescript const { ipcRenderer } = require('electron'); clickButton.addEventListener('click', () => { ipcRenderer.send('button-click'); });
This demonstrates how you can send messages between processes using Electron’s ipcRenderer and ipcMain.
6. Packaging and Distributing Your Application
After developing your Electron application with TypeScript, it’s time to package and distribute it.
6.1. Packaging for Different Platforms
Electron provides tools to package your application for different platforms, creating installable files for Windows, macOS, and Linux. Use the electron-builder package to simplify this process:
- Install electron-builder as a development dependency:
bash npm install electron-builder --save-dev
2. Configure your package.json to include build information:
json { "build": { "appId": "your.app.id", "productName": "Your App Name", "directories": { "output": "dist" }, "files": [ "dist/**/*", "main.js", "index.html", "styles.css", "renderer.js" ], "linux": { "target": "AppImage" }, "mac": { "target": "dmg" }, "win": { "target": "nsis" } } }
3. Build your application using electron-builder:
bash npx electron-builder
6.2. Code Signing and Security Considerations
When distributing your Electron application, consider code signing to ensure authenticity and prevent tampering. Code signing certificates can be obtained from various certificate authorities.
Additionally, adhere to security best practices such as validating user inputs, implementing secure communication, and staying up to date with security updates for the libraries and packages you use.
7. Advanced Topics and Best Practices
As you become more comfortable with Electron and TypeScript, you can explore advanced topics and best practices to enhance your application’s quality and performance.
7.1. Managing State with Redux
For more complex applications, managing state can become challenging. Integrating Redux, a state management library, can help you manage and synchronize application state across various components.
7.2. Testing Your Electron Application
Adopting testing practices is crucial to ensure the reliability of your application. Explore tools like Jest and Spectron for unit and end-to-end testing of your Electron application.
7.3. Performance Optimization Tips
Electron applications can sometimes suffer from performance issues. To optimize performance, consider techniques such as lazy loading, minimizing memory usage, and optimizing rendering.
Conclusion
Creating cross-platform desktop applications has never been easier, thanks to Electron and TypeScript. By combining the power of web technologies and the capabilities of native desktop applications, developers can create versatile and powerful software that runs seamlessly across different operating systems. Throughout this guide, you’ve learned how to set up your development environment, build user interfaces, make applications interactive with TypeScript, package and distribute applications, and explore advanced topics. Armed with this knowledge, you’re ready to embark on a journey of creating your own feature-rich, cross-platform desktop applications. Happy coding!
In this guide, we’ve explored the exciting world of creating cross-platform desktop applications using Electron and TypeScript. We started by introducing the powerful combination of Electron’s cross-platform capabilities and TypeScript’s robust type system. We walked through setting up your development environment, creating a basic Electron application structure, building user interfaces with HTML and CSS, and making your application interactive with TypeScript. Additionally, we delved into packaging and distributing your application and covered advanced topics like state management, testing, and performance optimization.
Table of Contents
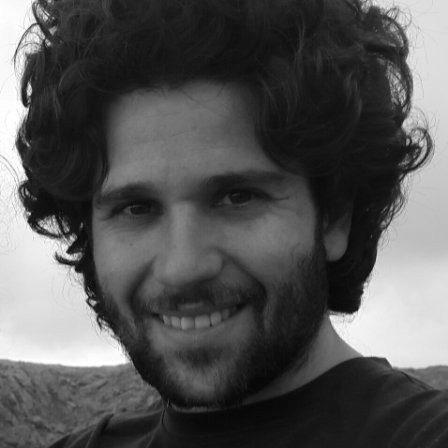
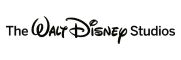