TypeScript and Data Analysis: Crunching Numbers with ease
In today’s data-driven world, the ability to analyze and extract meaningful insights from data is more valuable than ever. Whether you are working on a scientific research project, building a financial model, or simply exploring data for decision-making, having the right tools and languages at your disposal can make a world of difference. TypeScript, with its strong typing and modern features, is one such language that can empower data analysts and scientists to crunch numbers with ease.
Table of Contents
Why TypeScript for Data Analysis?
1. Type Safety
One of the key advantages of TypeScript for data analysis is its strong type system. Unlike JavaScript, where types are often inferred at runtime, TypeScript enforces type checking at compile time. This means that you can catch type-related errors before they make it into your analysis code.
Let’s look at a simple example to illustrate the point. Consider a function that calculates the average of an array of numbers:
typescript function calculateAverage(numbers: number[]): number { const sum = numbers.reduce((acc, num) => acc + num, 0); return sum / numbers.length; }
In this TypeScript code, we explicitly specify that the numbers parameter must be an array of numbers. If you attempt to pass an array of strings or any other type, TypeScript will raise a compilation error. This type safety reduces the likelihood of runtime errors and enhances the reliability of your data analysis code.
2. Code Readability
Data analysis code can quickly become complex, especially when dealing with large datasets or intricate calculations. TypeScript’s strong typing can make your code more self-documenting and easier to understand. When you or your team revisit the code weeks or months later, having type annotations can provide valuable context.
Consider the following TypeScript code that calculates the correlation coefficient between two arrays of numbers:
typescript function calculateCorrelation(x: number[], y: number[]): number { // Implementation here... }
In this snippet, the type annotations x: number[] and y: number[] clearly indicate the expected input types, making it easier for anyone reading the code to grasp its purpose without diving into the implementation details.
Data Analysis Libraries in TypeScript
To leverage TypeScript for data analysis, you can take advantage of various libraries and tools that are well-suited for this purpose. Here are some of the popular ones:
1. num.ts
num.ts is a TypeScript library that provides a wide range of mathematical and statistical functions for data analysis. It includes functions for basic operations like mean, median, and standard deviation, as well as more advanced statistical tests like t-tests and chi-squared tests.
Here’s an example of how you can use num.ts to calculate the mean and standard deviation of an array of numbers:
typescript import { mean, std } from 'num.ts'; const data = [1, 2, 3, 4, 5]; const average = mean(data); const deviation = std(data); console.log(`Mean: ${average}`); console.log(`Standard Deviation: ${deviation}`);
2. pandas-js
For those familiar with Python’s Pandas library, pandas-js is a TypeScript equivalent that provides powerful data manipulation and analysis capabilities. It allows you to perform operations like filtering, grouping, and aggregation on tabular data with ease.
Here’s a snippet demonstrating how to load data into a pandas-js DataFrame and perform basic operations:
typescript import { DataFrame } from 'pandas-js'; const data = [ { name: 'Alice', age: 28 }, { name: 'Bob', age: 35 }, { name: 'Charlie', age: 22 }, // More data... ]; const df = new DataFrame(data); // Filter the DataFrame const filteredDf = df.where((row) => row.age > 25); // Calculate the mean age const meanAge = filteredDf.get('age').mean();
3. d3.js with TypeScript
If you’re into data visualization as part of your analysis, d3.js is a powerful library for creating interactive and engaging data visualizations. With TypeScript, you can benefit from type definitions that make working with d3.js more straightforward.
Here’s a simple example of creating a bar chart using d3.js with TypeScript:
typescript import * as d3 from 'd3'; const data = [10, 20, 30, 40, 50]; const width = 400; const height = 200; const svg = d3.select('body').append('svg').attr('width', width).attr('height', height); const xScale = d3.scaleLinear().domain([0, d3.max(data)]).range([0, width]); const yScale = d3.scaleLinear().domain([0, d3.max(data)]).range([height, 0]); svg.selectAll('rect') .data(data) .enter() .append('rect') .attr('x', (d, i) => i * (width / data.length)) .attr('y', (d) => yScale(d)) .attr('width', width / data.length - 1) .attr('height', (d) => height - yScale(d)) .attr('fill', 'blue');
TypeScript for Machine Learning and AI
In addition to traditional data analysis, TypeScript can also be applied to machine learning and artificial intelligence (AI) tasks. Several libraries and frameworks enable you to build machine learning models using TypeScript. Let’s take a look at a couple of them:
1. TensorFlow.js
TensorFlow.js is a JavaScript library for training and deploying machine learning models in the browser and on Node.js. With TypeScript support, you can write type-safe code for tasks like image recognition, natural language processing, and more.
Here’s an example of using TensorFlow.js with TypeScript to create a simple neural network:
typescript import * as tf from '@tensorflow/tfjs-node'; const model = tf.sequential(); model.add(tf.layers.dense({ units: 10, activation: 'relu', inputShape: [5] })); model.add(tf.layers.dense({ units: 1, activation: 'linear' })); model.compile({ loss: 'meanSquaredError', optimizer: 'sgd' }); const xs = tf.tensor2d([[1, 2, 3, 4, 5]]); const ys = tf.tensor2d([[10]]); model.fit(xs, ys, { epochs: 1000 }).then(() => { const prediction = model.predict(tf.tensor2d([[6, 7, 8, 9, 10]])); prediction.print(); });
2. Synaptic
Synaptic is a neural network library for Node.js that offers TypeScript support. It allows you to build and train custom neural networks for various machine learning tasks.
Here’s a basic example of creating a neural network for XOR gate prediction using Synaptic and TypeScript:
typescript import { Architect, Trainer, Network } from 'synaptic'; const inputLayer = new Architect.Perceptron(2, 4, 1); const network = new Network({ input: inputLayer }); const trainingData = [ { input: [0, 0], output: [0] }, { input: [0, 1], output: [1] }, { input: [1, 0], output: [1] }, { input: [1, 1], output: [0] }, ]; const trainer = new Trainer(network); trainer.train(trainingData); const result = network.activate([0, 1]); console.log(`Predicted output: ${result[0]}`);
Challenges and Considerations
While TypeScript brings numerous benefits to data analysis and machine learning, it’s essential to acknowledge some challenges and considerations:
1. Learning Curve
If you’re new to TypeScript, there may be a learning curve involved in understanding its type system and features. However, the investment in learning TypeScript can pay off in terms of code quality and reliability.
2. Library Support
Although TypeScript has gained popularity in recent years, not all data analysis and machine learning libraries have robust TypeScript support. You may need to write custom type definitions or use TypeScript’s any type for certain libraries.
3. Performance
TypeScript compiles to JavaScript, which may not be as performant as languages like Python or C++ for certain data-intensive operations. However, TypeScript’s performance is often sufficient for many data analysis tasks.
Conclusion
In the world of data analysis and machine learning, TypeScript offers a powerful and flexible environment for crunching numbers with ease. Its strong type system enhances code reliability and readability, making it a valuable choice for both traditional data analysis and machine learning tasks. With the right libraries and tools, you can harness TypeScript’s capabilities to explore, analyze, and extract insights from data effectively.
So, whether you’re a data scientist, analyst, or machine learning enthusiast, consider adding TypeScript to your toolbox for a more robust and type-safe approach to data analysis. The world of data awaits your exploration, and TypeScript is here to help you make sense of it all. Happy coding!
Table of Contents
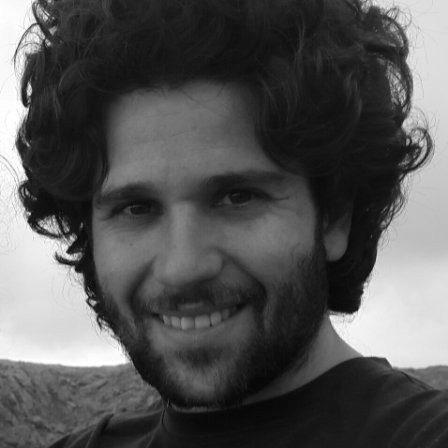
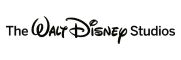