Deploying TypeScript Applications with Docker
In today’s fast-paced software development landscape, deploying applications quickly and reliably is crucial. Docker, a leading containerization platform, has revolutionized the way applications are deployed and managed. When combined with TypeScript, a powerful and statically typed superset of JavaScript, Docker can enhance the deployment process for TypeScript applications. In this guide, we will explore the process of deploying TypeScript applications using Docker, from setting up your development environment to creating Docker images and orchestrating containers.
Table of Contents
1. Introduction to Docker and TypeScript
Docker has transformed the way developers package, distribute, and run applications by encapsulating them in isolated environments known as containers. Containers ensure that applications run consistently across different environments, making deployment more predictable and efficient.
TypeScript, on the other hand, enhances the JavaScript development experience by providing static typing, better tooling, and improved code organization. Combining TypeScript with Docker brings a powerful synergy to the deployment process, as it ensures that the application code and its dependencies are encapsulated together in a container, eliminating potential conflicts and inconsistencies.
2. Setting Up Your Development Environment
Before diving into the deployment process, ensure you have the necessary tools in place.
2.1. Installing Node.js and TypeScript
To develop TypeScript applications, you need Node.js installed. Node.js comes with the Node Package Manager (npm), which is essential for managing dependencies.
bash # Install Node.js and npm sudo apt-get update sudo apt-get install nodejs npm # Check installations node -v npm -v # Install TypeScript globally npm install -g typescript
2.2. Installing Docker
To work with Docker, you need to install the Docker Engine, which provides the necessary tools for creating, managing, and running containers.
Visit the official Docker documentation to find installation instructions for your specific operating system.
3. Creating a TypeScript Application
For demonstration purposes, let’s create a simple TypeScript application. Create a new directory for your project and navigate into it:
bash mkdir my-ts-app cd my-ts-app
Initialize a Node.js project:
bash npm init -y
Next, create a TypeScript file (e.g., app.ts) and write a simple “Hello, Docker!” program:
typescript // app.ts const message: string = "Hello, Docker!"; console.log(message);
4. Writing a Dockerfile for Your Application
A Dockerfile is a script that defines the steps necessary to create a Docker image. Here’s how you can create a Dockerfile for your TypeScript application:
Dockerfile # Use an official Node.js runtime as the base image FROM node:14 # Set the working directory in the container WORKDIR /app # Copy package.json and package-lock.json to the container COPY package*.json ./ # Install application dependencies RUN npm install # Copy the rest of the application code to the container COPY . . # Specify the command to run your application CMD [ "node", "app.ts" ]
In this Dockerfile:
- We’re using the official Node.js runtime image as our base image.
- The WORKDIR instruction sets the working directory inside the container to /app.
- We copy the package.json and package-lock.json files first and run npm install to install dependencies.
- The remaining application code is then copied to the container.
- Finally, the CMD instruction specifies the command to run when the container starts.
5. Building a Docker Image
With the Dockerfile in place, you can now build a Docker image for your TypeScript application:
bash docker build -t my-ts-app .
The -t flag tags the image with the name my-ts-app.
6. Running Containers from Your Image
Once the image is built, you can run containers from it. This isolates your application and its dependencies, ensuring consistency across environments.
bash docker run -p 8080:8080 my-ts-app
The -p flag maps port 8080 from the host to port 8080 in the container. Open your web browser and navigate to http://localhost:8080 to see the “Hello, Docker!” message.
7. Docker Compose for Streamlined Development
Docker Compose is a tool that allows you to define and manage multi-container Docker applications. It simplifies the process of managing interconnected services and their dependencies.
Create a docker-compose.yml file in your project directory:
yaml version: '3' services: my-ts-app: build: . ports: - "8080:8080"
Run your application using Docker Compose:
bash docker-compose up
8. Deploying to a Production Environment
In a production environment, you’ll likely need to manage multiple containers and orchestrate them for high availability and scalability. Kubernetes is a powerful orchestration platform that can help you manage Docker containers at scale.
8.1. Container Orchestration (Kubernetes)
Kubernetes allows you to define and manage the deployment, scaling, and operation of containerized applications. It abstracts the underlying infrastructure, providing a consistent way to manage containers across different environments.
Setting up Kubernetes and deploying your application is beyond the scope of this guide, but learning Kubernetes can significantly enhance your ability to manage production deployments effectively.
9. Best Practices for Dockerizing TypeScript Apps
When Dockerizing TypeScript applications, consider these best practices to ensure efficient and maintainable deployments:
- Keeping Images Small: Use multi-stage builds to keep image sizes minimal by only including necessary files and dependencies.
- Using Multi-Stage Builds: Multi-stage builds allow you to build intermediate images for compiling TypeScript and then create a smaller image for running the application. This reduces the attack surface and keeps images lightweight.
- Properly Handling Dependencies: Ensure you only install production dependencies in the final image to reduce potential security risks.
Conclusion
Deploying TypeScript applications with Docker brings simplicity, consistency, and scalability to your development process. By encapsulating your application and its dependencies in containers, you can confidently deploy your application across different environments without worrying about environment-specific issues. Incorporate Docker and TypeScript into your workflow to streamline development and deliver robust applications to your users.
Table of Contents
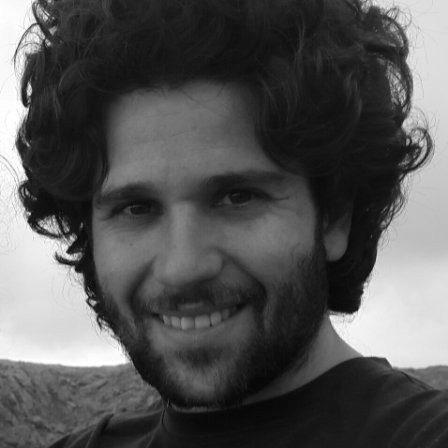
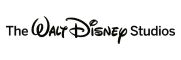