TypeScript in Production: Deployment and Performance Tips
In the world of modern web development, TypeScript has emerged as a powerful and popular programming language. Its strong typing capabilities, combined with the flexibility of JavaScript, make it an ideal choice for building complex and maintainable applications. However, as your TypeScript project moves from development to production, several challenges related to deployment and performance optimization arise. In this blog post, we will delve into some crucial tips and best practices to ensure that your TypeScript applications run smoothly in a production environment.
Table of Contents
1. Optimizing TypeScript Code
1.1. Leveraging Static Typing
One of TypeScript’s primary advantages is its static typing system, which catches type-related errors at compile time. By taking full advantage of TypeScript’s type annotations, you can reduce the chances of runtime errors, making your application more robust. Strong typing also enhances code readability and maintainability.
typescript interface User { id: number; name: string; } function getUserInfo(user: User): string { return `ID: ${user.id}, Name: ${user.name}`; }
1.2. Tree Shaking
When deploying TypeScript applications, minimizing the bundle size is crucial for performance. Tree shaking is a technique that eliminates unused code from the final bundle. Make sure to use ES6 module syntax and avoid importing entire modules when you only need specific functions or classes.
typescript import { getUserInfo } from './userModule'; // Only getUserInfo function is included in the bundle
1.3. Minification and Dead Code Elimination
Minification reduces the size of your code by removing unnecessary characters like whitespace and comments. Additionally, enabling dead code elimination ensures that parts of your code that are never executed are excluded from the bundle.
Tools like Terser can be integrated into your build process to achieve both minification and dead code elimination.
2. Effective Error Handling
2.1. Robust Type Checking
TypeScript’s type system helps catch type-related errors, but it’s essential to perform additional checks when interacting with external data sources or APIs. Utilize runtime type checking libraries like io-ts or class-validator to validate incoming data.
typescript import * as t from 'io-ts'; const User = t.type({ id: t.number, name: t.string, }); const userData = { id: '123', name: 'John' }; if (User.is(userData)) { // Proceed with processing the user data } else { // Handle validation errors }
2.2. Runtime Error Management
Even with strong typing, runtime errors can occur. Implement proper error handling mechanisms, such as try-catch blocks, to gracefully handle unexpected issues and prevent application crashes.
typescript try { // Risky operation } catch (error) { // Handle the error }
2.3. Monitoring and Logging
In a production environment, monitoring and logging play a critical role in identifying and diagnosing issues. Integrate logging libraries like Winston or Bunyan to capture relevant information about errors, user interactions, and application behavior. Consider using centralized logging solutions for easier management and analysis.
3. Bundling Strategies
3.1. Module Bundlers
Module bundlers like Webpack or Rollup are essential for packaging your TypeScript code and its dependencies into a format that browsers can understand. Configure your bundler to optimize for production by enabling features like minification, tree shaking, and code splitting.
3.2. Code Splitting
Code splitting is a technique that divides your application into smaller chunks, allowing users to only download the code they need initially. This results in faster initial load times and improved performance.
typescript // Dynamically load a module import('./lazyModule').then((module) => { // Use the module });
3.3. Lazy Loading
Lazy loading involves loading specific parts of your application only when they are needed. This is particularly useful for large applications with multiple routes or sections.
4. Fine-tuning Performance
4.1. AOT Compilation
Ahead-of-Time (AOT) compilation translates your TypeScript code into highly optimized JavaScript during the build process. This eliminates the need for runtime compilation, leading to faster load times.
bash ng build --aot
4.2. Memoization Techniques
Memoization is a caching technique that can significantly improve the performance of functions by storing the results of expensive computations and returning the cached result when the same inputs occur again.
typescript function calculateExpensiveValue(input: number): number { // Expensive computation } const memoizedCalculate = memoize(calculateExpensiveValue);
4.3. Debouncing and Throttling
In scenarios where frequent user interactions trigger actions (like search suggestions), debouncing and throttling can prevent excessive function calls and improve performance.
typescript // Debouncing const debounceSearch = debounce(searchFunction, 300); // Throttling const throttleScroll = throttle(scrollFunction, 200);
5. Deployment Automation
5.1. Continuous Integration and Deployment
Implementing CI/CD pipelines automates the process of building, testing, and deploying your TypeScript application. Services like Jenkins, CircleCI, or GitHub Actions can be used to ensure that your code is consistently delivered to production environments with minimal manual intervention.
5.2. Dockerization
Containerizing your TypeScript application using Docker allows for consistent deployment across different environments. Docker containers encapsulate your application, its dependencies, and runtime configuration, reducing the chances of deployment-related issues.
5.3. Cloud Services
Leverage cloud platforms like AWS, Azure, or Google Cloud to host and scale your TypeScript applications. These services provide tools for easy deployment, load balancing, auto-scaling, and monitoring.
Conclusion
Deploying and optimizing TypeScript applications for production requires careful consideration of various aspects, including code optimization, error handling, bundling strategies, performance fine-tuning, and deployment automation. By following these tips and best practices, you can ensure that your TypeScript projects not only run smoothly but also deliver exceptional performance and reliability in real-world production environments. Remember, the journey doesn’t end after development; it’s crucial to continuously monitor, analyze, and improve your application to meet the evolving needs of your users and the demands of the digital landscape.
Table of Contents
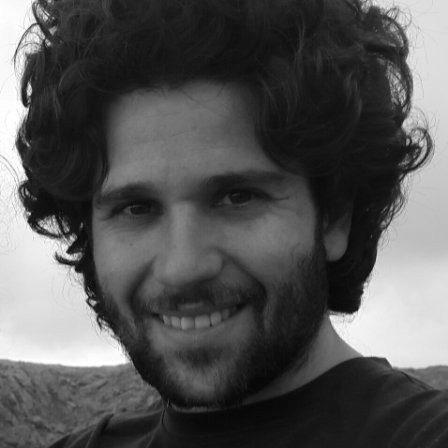
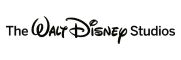