Hire TypeScript Developers Interview Questions Guide
TypeScript, a superset of JavaScript, brings static typing and enhanced tooling to JavaScript development. When hiring TypeScript developers, it’s crucial to assess their understanding of TypeScript, JavaScript, and web development. This guide will assist you in navigating the hiring process effectively, enabling you to identify candidates with the right skills for your projects.
Table of Contents
1. Reasons to Hire TypeScript Developers
Embarking on the journey to hire TypeScript developers? Here are key reasons to consider:
1.1 Type Safety
TypeScript introduces static typing, catching errors at compile-time and providing enhanced code quality and maintainability.
1.2 Enhanced Tooling
Benefit from better IDE support, code navigation, and refactoring capabilities, improving developer productivity.
1.3 JavaScript Compatibility
Seamlessly integrate TypeScript into existing JavaScript projects, allowing for gradual adoption and easy collaboration.
2. How to Hire TypeScript Developers
Follow these steps for a successful TypeScript developer hiring process:
2.1 Job Requirements
Define specific job prerequisites, emphasizing skills such as TypeScript, JavaScript, front-end frameworks (e.g., React, Angular, or Vue), and knowledge of modern web development.
2.2 Search Channels
Utilize CloudDevs’ expertise to connect with potential TypeScript developers. Leverage job postings, online platforms, and tech communities to discover talented individuals.
2.3 Screening
Scrutinize candidates’ TypeScript proficiency, web development experience, and understanding of front-end technologies.
2.4 Technical Assessment
Develop a comprehensive technical assessment, including coding challenges and real-world scenarios related to TypeScript development.
3. Core Skills of TypeScript Developers to Look For
When evaluating TypeScript developers, focus on these core skills:
- TypeScript Proficiency: A deep understanding of TypeScript syntax, features, and best practices.
- JavaScript Expertise: Strong knowledge of JavaScript, especially ES6+ features.
- Front-End Frameworks: Experience with popular front-end frameworks like React, Angular, or Vue.
- Web Development Skills: Proficiency in HTML, CSS, and understanding of responsive web design.
- Testing: Familiarity with testing frameworks (e.g., Jest, Jasmine) and writing unit tests.
4. Overview of the TypeScript Developer Hiring Process
Here’s an overview of the TypeScript developer hiring process:
4.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking in TypeScript developers.
4.2 Crafting Compelling Job Descriptions
Create engaging job descriptions that accurately convey the role, emphasizing TypeScript-specific skills required.
4.3 Crafting TypeScript Developer Interview Questions
Develop a comprehensive set of interview questions covering TypeScript intricacies, web development, and relevant front-end technologies.
5. Sample TypeScript Developer Interview Questions and Answers
Explore these sample questions with detailed answers to assess candidates’ TypeScript skills:
Q1. Explain the key differences between JavaScript and TypeScript.
A:
TypeScript is a superset of JavaScript, adding static typing and other features. Key differences include TypeScript’s static typing, interfaces, and the ability to use the latest ECMAScript features.
Q2. How does TypeScript help in catching errors during development?
A:
TypeScript introduces static typing, enabling the compiler to catch type-related errors during development, providing early feedback and improving code robustness.
Q3. Implement a TypeScript class that represents a basic TODO item with properties for title and completion status.
A:
// Sample Answer: TypeScript TODO class class Todo { constructor(public title: string, public completed: boolean) {} } // Example usage const myTodo = new Todo('Complete TypeScript guide', false);
Q4. Describe the benefits of using TypeScript with React.
A:
TypeScript enhances React development by providing static typing, improved code navigation, and autocompletion. It helps catch common errors early in the development process.
Q5. How can you handle asynchronous operations in TypeScript?
A:
In TypeScript, you can handle asynchronous operations using Promises, async/await syntax, or libraries like RxJS for reactive programming.
Q6. Write a TypeScript function that takes an array of numbers and returns the sum.
A:
// Sample Answer: TypeScript sum function function sum(numbers: number[]): number { return numbers.reduce((acc, num) => acc + num, 0); } // Example usage const result = sum([1, 2, 3, 4]); // Result: 10
Q7. Explain the concept of TypeScript interfaces and provide an example.
A:
TypeScript interfaces define the structure of objects. Example:
// Sample Answer: TypeScript interface interface Person { firstName: string; lastName: string; } // Example usage const person: Person = { firstName: 'John', lastName: 'Doe' };
Q8. How does TypeScript support the concept of “union types”?
A:
TypeScript allows the creation of union types, which can hold values of multiple types. Example:
// Sample Answer: TypeScript union types type Status = 'success' | 'error'; // Example usage let response: Status = 'success';
Q9. Implement a TypeScript class that represents a geometric shape with methods to calculate area and perimeter.
A:
// Sample Answer: TypeScript geometric shape class abstract class Shape { abstract calculateArea(): number; abstract calculatePerimeter(): number; } class Circle extends Shape { constructor(private radius: number) { super(); } calculateArea(): number { return Math.PI * this.radius ** 2; } calculatePerimeter(): number { return 2 * Math.PI * this.radius; } } // Example usage const circle = new Circle(5); console.log(circle.calculateArea()); // Result: ~78.54 console.log(circle.calculatePerimeter()); // Result: ~31.42
Q10. How can you share code between the front end and back end using TypeScript?
A:
You can achieve code sharing using TypeScript’s ability to compile to different module systems (CommonJS, ES modules). Shared code can be placed in a separate package and used by both front-end and back-end projects.
6. Hiring TypeScript Developers through CloudDevs
Step 1: Connect with CloudDevs
Initiate a conversation with a CloudDevs consultant to discuss your project requirements, preferred skills, and expected experience.
Step 2: Discover Your Ideal Match
Within a short timeframe, CloudDevs presents you with carefully selected TypeScript developers from their pool of pre-vetted professionals. Review their profiles and select the candidate who aligns with your project’s vision.
Step 3: Embark on a Risk-Free Trial
Engage in discussions with your chosen developer to ensure a smooth onboarding process. Once satisfied, formalize the collaboration and commence a week-long free trial.
By leveraging the expertise of CloudDevs, you can effortlessly identify and hire exceptional TypeScript developers, ensuring your team possesses the skills required to build remarkable web applications.
7. Conclusion
With these additional technical questions and insights at your disposal, you’re now well-prepared to assess TypeScript developers comprehensively. Whether you’re developing front-end applications, Node.js services, or both, securing the right TypeScript developers for your team is pivotal to the success of your projects.
Table of Contents
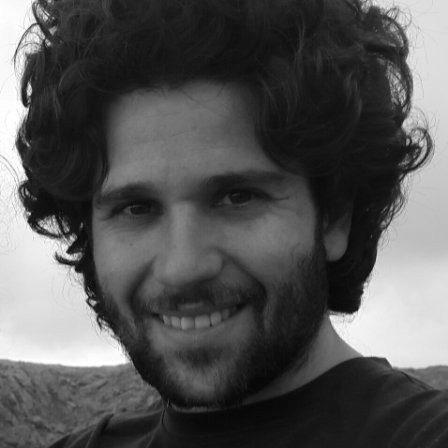
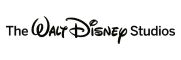