Managing State in TypeScript Applications
In the ever-evolving landscape of web development, creating complex applications demands a robust and efficient approach to managing state. Whether you’re building a small-scale project or a large enterprise-level application, effectively managing state is crucial to maintain code clarity, scalability, and maintainability. In this article, we will delve into various strategies and techniques for managing state in TypeScript applications, including local state, global state, and the use of state management libraries.
Table of Contents
State, in the context of a web application, refers to the data that represents the current state of the application. Managing this data effectively is crucial to ensure that your application behaves as expected and is maintainable as it grows in complexity. In TypeScript applications, which bring the benefits of static typing, managing state becomes even more critical to prevent errors and promote code reliability.
1. Local State Management
Local state pertains to data that is confined to a particular component. It’s used when the data only affects a specific part of the user interface and doesn’t need to be shared with other components.
1.1 Understanding Local State
Local state is typically used for managing component-specific data. For example, if you’re building a form, you might use local state to store the user’s input as they fill out the form fields. Local state is managed within the component itself and doesn’t impact other components.
1.2 Using the useState Hook
In React applications, the useState hook is a powerful tool for managing local state. Let’s see how you can use it in a TypeScript component:
tsx import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState<number>(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }
In this example, the useState hook is used to manage the count state variable, which starts at 0. The setCount function is used to update the state whenever the “Increment” button is clicked.
1.3 Managing Local State with Classes
If you’re using class components, you can manage local state using the setState method. Here’s an equivalent example using a class component:
tsx import React, { Component } from 'react'; class CounterClass extends Component<{}, { count: number }> { state = { count: 0, }; render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={() => this.setState({ count: this.state.count + 1 })}>Increment</button> </div> ); } }
Both examples achieve the same result: managing local state within a component. Choose the approach that aligns with your preferred coding style and the project’s requirements.
2. Global State Management
Global state comes into play when data needs to be shared across multiple components that are not directly connected in the component tree. Instead of passing data through props from parent to child components, global state allows components to access and update shared data directly.
2.1 When to Use Global State
Global state management is suitable for scenarios where data needs to be accessible to multiple parts of your application without the complexity of prop drilling. For instance, user authentication status, shopping cart items, or theme preferences could be managed using global state.
2.2 Introducing Redux for Global State
Redux is a popular state management library that offers a predictable and centralized way of managing global state in a React application. It enforces unidirectional data flow and helps prevent spaghetti code by maintaining a single source of truth for your application’s data.
2.3 Implementing Global State with Redux in TypeScript
To use Redux with TypeScript, you need to install the necessary packages:
bash npm install redux react-redux @types/react-redux
Next, let’s create a simple example using Redux to manage a user’s authentication state:
tsx // actions.ts export const setUserAuthenticated = (authenticated: boolean) => ({ type: 'SET_USER_AUTHENTICATED', payload: authenticated, }); // reducers.ts interface AppState { isAuthenticated: boolean; } const initialState: AppState = { isAuthenticated: false, }; const appReducer = (state = initialState, action: any) => { switch (action.type) { case 'SET_USER_AUTHENTICATED': return { ...state, isAuthenticated: action.payload }; default: return state; } }; export default appReducer; // store.ts import { createStore } from 'redux'; import appReducer from './reducers'; const store = createStore(appReducer); export default store; // App.tsx import React from 'react'; import { Provider, useSelector, useDispatch } from 'react-redux'; import store from './store'; import { setUserAuthenticated } from './actions'; function App() { const isAuthenticated = useSelector((state: AppState) => state.isAuthenticated); const dispatch = useDispatch(); return ( <Provider store={store}> <div> <p>User Authenticated: {isAuthenticated ? 'Yes' : 'No'}</p> <button onClick={() => dispatch(setUserAuthenticated(true))}>Log In</button> </div> </Provider> ); } export default App;
In this example, actions are defined to modify the state, a reducer combines these actions into the application’s state, and a Redux store holds the state. The useSelector hook allows components to access the global state, and the useDispatch hook provides a way to dispatch actions to modify the state.
3. State Management Libraries
While Redux is a powerful solution for global state management, there are other libraries available that offer different trade-offs in terms of simplicity and ease of use.
3.1 MobX: Simple and Observable State Management
MobX is a state management library that uses observables to automatically track and update changes to the state. It’s known for its simplicity and ease of integration with React applications.
Here’s a brief example of using MobX in TypeScript:
tsx import { observable, action } from 'mobx'; class TodoStore { @observable todos: string[] = []; @action addTodo(todo: string) { this.todos.push(todo); } } const todoStore = new TodoStore(); export default todoStore;
In this example, the @observable decorator marks the todos array as observable. The @action decorator marks the addTodo function as an action that modifies the state. This allows components to react automatically when the state changes.
3.2 Zustand: Minimalistic State Management
Zustand is a lightweight state management library that embraces hooks and functional programming principles. It provides a simple and intuitive API for managing global state.
Here’s a minimal example of using Zustand in TypeScript:
tsx import create from 'zustand'; type TodoState = { todos: string[]; addTodo: (todo: string) => void; }; const useTodoStore = create<TodoState>((set) => ({ todos: [], addTodo: (todo) => set((state) => ({ todos: [...state.todos, todo] })), })); export default useTodoStore;
In this example, the create function from Zustand is used to create a custom hook, useTodoStore. The hook returns the state and a function to update the state. The updates are made immutably, similar to how the useState hook works.
4. Best Practices for State Management
While different applications have unique requirements, there are some best practices that can help maintain a clean and maintainable state management solution.
4.1 Keep State Changes Predictable
In global state management, always update the state using predefined actions and reducers. This makes it easier to track changes and understand the state transitions in your application.
4.2 Separate Concerns with Modularization
Break down your state management into manageable modules or slices. This reduces the complexity of your global state and helps with organization and collaboration in larger projects.
4.3 Embrace Immutability
Whether you’re using local state or global state, follow the principle of immutability. Instead of modifying the state directly, create new instances with the necessary changes. This helps prevent unintended side effects and enhances predictability.
Conclusion
Effective state management is a cornerstone of building maintainable and scalable TypeScript applications. By carefully choosing between local and global state, leveraging state management libraries like Redux, MobX, or Zustand, and adhering to best practices, you can create applications that are more reliable, easier to understand, and ready for future growth. As your project evolves, keep experimenting and refining your state management strategy to strike the right balance between complexity and simplicity.
Table of Contents
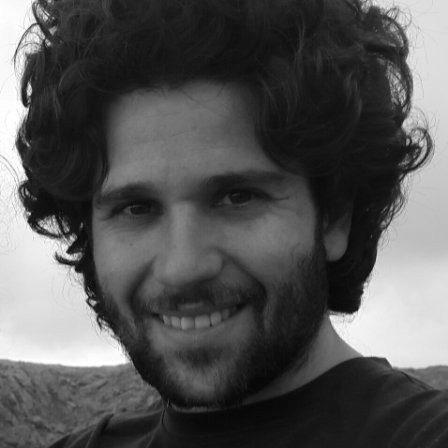
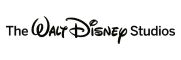