Migrating JavaScript Projects to TypeScript
In the dynamic world of web development, staying updated with the latest trends and technologies is crucial. One such advancement that has gained significant popularity is TypeScript – a statically typed superset of JavaScript. Many JavaScript projects have embraced TypeScript due to its ability to catch type-related errors early in the development process, resulting in more reliable and maintainable codebases. In this blog post, we will delve into the reasons behind migrating JavaScript projects to TypeScript, the benefits it offers, the step-by-step migration process, and illustrate each step with code samples.
Table of Contents
1. Why Migrate JavaScript to TypeScript?
1.1. Type Safety and Early Error Detection
JavaScript, being a dynamically typed language, can sometimes lead to runtime errors that might go unnoticed until the code is executed. TypeScript, on the other hand, introduces static typing, allowing developers to define types for variables, function parameters, and return values. This enables the TypeScript compiler to catch type-related errors during development, reducing the chances of runtime errors and enhancing code quality.
typescript // JavaScript function add(a, b) { return a + b; } // TypeScript function add(a: number, b: number): number { return a + b; }
1.2. Improved Code Maintainability
As projects grow in complexity, maintaining JavaScript code can become challenging. TypeScript’s type annotations make the codebase more self-documenting, making it easier for developers to understand and modify code. This enhanced readability leads to improved collaboration and reduces the time required for onboarding new team members.
1.3. Better IDE Support and Auto-completion
TypeScript’s static typing enables powerful code analysis and auto-completion features in modern integrated development environments (IDEs) like Visual Studio Code. This aids developers by providing real-time feedback, suggesting method names, and even offering details about available properties and methods of objects.
1.4. Refactoring with Confidence
Refactoring is a crucial part of software development. With TypeScript, refactoring becomes less daunting as the compiler ensures that changes propagate consistently across the codebase. Renaming variables, extracting functions, and reorganizing code can be done with confidence, knowing that type-related errors will be caught by the compiler.
2. Benefits of Migrating to TypeScript
2.1. Enhanced Developer Productivity
While there might be an initial learning curve, the benefits of TypeScript quickly outweigh the investment. TypeScript’s features like type inference, interfaces, and generics enable developers to write more concise and expressive code. The improved developer experience leads to faster development and fewer bugs.
2.2. Robust Tooling and Ecosystem
TypeScript has a thriving ecosystem with a wide range of libraries, frameworks, and tools built specifically for it. This ecosystem empowers developers to leverage the best practices of TypeScript in their projects, leading to better code quality and maintainability.
2.3. Seamless Integration
TypeScript can be gradually integrated into existing JavaScript projects. This means you don’t need to rewrite your entire codebase overnight. You can start by converting individual files to TypeScript and gradually migrate the entire project, ensuring minimal disruption to ongoing development.
2.4. Continuous Improvement
TypeScript is an open-source project backed by Microsoft. This means it’s actively maintained and regularly updated with new features, improvements, and bug fixes. By migrating to TypeScript, you align your project with a technology that is constantly evolving to meet the needs of modern development.
3. Migrating from JavaScript to TypeScript: A Step-by-Step Guide
Step 1: Setting Up TypeScript
The first step is to install TypeScript as a dependency in your project. You can do this using npm or yarn:
bash npm install typescript --save-dev # or yarn add typescript --dev
Once TypeScript is installed, create a tsconfig.json file in the root of your project to configure TypeScript settings. You can start with a basic configuration:
json { "compilerOptions": { "target": "ESNext", "module": "CommonJS", "outDir": "./dist", "strict": true } }
Step 2: Renaming Files to .ts
Begin the migration process by renaming your .js files to .ts. TypeScript can understand JavaScript syntax, so your existing code will still work. However, to fully leverage TypeScript’s features, you’ll need to gradually add type annotations.
Step 3: Adding Type Annotations
Start by adding type annotations to variables and function parameters. TypeScript allows you to specify types using the : syntax. For example:
typescript // Before function calculateTotal(price, quantity) { return price * quantity; } // After function calculateTotal(price: number, quantity: number): number { return price * quantity; }
Step 4: Type Inference and Any Type
TypeScript’s type inference can save you time by automatically determining the type of a variable based on its value. However, you might encounter situations where TypeScript assigns the any type. Gradually replace any with more specific types to harness TypeScript’s benefits fully.
Step 5: Interfaces and Custom Types
Interfaces and custom types provide a way to define complex data structures with specific shapes. Instead of relying solely on inline type annotations, consider creating interfaces or types to improve code readability and reusability.
typescript // Before function printProduct(product) { console.log(product.name, product.price); } // After interface Product { name: string; price: number; } function printProduct(product: Product) { console.log(product.name, product.price); }
Step 6: Handling Null and Undefined
TypeScript has a feature called “strict null checks” enabled by default. This enforces a stricter approach to handling null and undefined values. Update your code to handle these cases explicitly, reducing the likelihood of runtime errors.
typescript // Before function getUser(id) { // ... } // After function getUser(id: number): User | null { // ... }
Step 7: Embracing Generics
Generics enable you to write flexible and reusable functions and classes. As you migrate your code, look for opportunities to introduce generics, enhancing the flexibility of your codebase.
typescript // Before function getLastElement(arr) { return arr[arr.length - 1]; } // After function getLastElement<T>(arr: T[]): T { return arr[arr.length - 1]; }
Step 8: Gradual Migration and Testing
Migrating a large project can be overwhelming. Start by migrating smaller, less critical parts of your codebase. This allows you to test TypeScript’s integration with your existing code and catch any issues early on.
Step 9: Enabling Strict Mode
As you become more comfortable with TypeScript, consider enabling stricter compiler options in your tsconfig.json file. This can help catch additional errors and enforce best practices.
Conclusion
Migrating a JavaScript project to TypeScript is a valuable investment in the long-term maintainability and reliability of your codebase. TypeScript’s type safety, enhanced developer productivity, and robust tooling make it an excellent choice for modern web development. By following the step-by-step migration process outlined in this guide, you can seamlessly transition your project to TypeScript while minimizing disruptions and maximizing the benefits it offers. So, take the leap and embark on the journey of enhancing your JavaScript project with the power of TypeScript.
In this blog post, we explored the reasons behind migrating JavaScript projects to TypeScript and highlighted the benefits this migration can bring. We covered the advantages of type safety, improved code maintainability, better IDE support, and confident refactoring. Additionally, we discussed the benefits of enhanced developer productivity, a robust tooling ecosystem, seamless integration, and continuous improvement within the TypeScript community.
The step-by-step migration guide provided insights into the practical aspects of migrating from JavaScript to TypeScript. We covered setting up TypeScript, renaming files, adding type annotations, utilizing interfaces and custom types, handling null and undefined values, embracing generics, and enabling strict mode. This comprehensive approach ensures a smooth and gradual migration process, allowing developers to transition their projects without major disruptions.
As the world of web development continues to evolve, staying up-to-date with the latest technologies is essential. Migrating JavaScript projects to TypeScript is a strategic move that not only aligns your codebase with modern development practices but also equips your team with the tools to create more maintainable and robust applications. By following the guidelines and best practices outlined in this blog post, you can confidently embark on the journey of migrating your JavaScript project to TypeScript, reaping the benefits for years to come.
Table of Contents
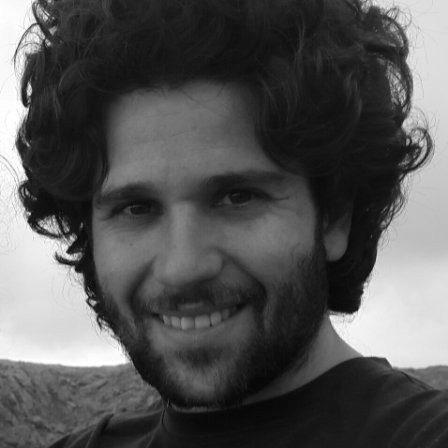
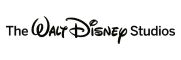