TypeScript and Natural Language Generation: AI-Powered Text
In the ever-evolving landscape of technology, two remarkable domains, TypeScript and Natural Language Generation (NLG), have converged to revolutionize text generation. TypeScript, a statically typed superset of JavaScript, provides a strong foundation for building robust and maintainable applications. On the other hand, NLG, powered by artificial intelligence (AI), enables computers to generate human-like text. When combined, TypeScript and NLG create a potent synergy that can be harnessed for various applications, from chatbots and content generation to data reporting and personalized messaging.
Table of Contents
This blog post delves into the exciting world of TypeScript and NLG, exploring how TypeScript can be used to enhance NLG capabilities and provide developers with powerful tools to create AI-powered text generation solutions. We’ll also provide code samples and practical insights to help you get started.
1. Understanding TypeScript
1.1. What is TypeScript?
TypeScript is a statically typed programming language developed by Microsoft. It is a strict syntactical superset of JavaScript that adds static typing capabilities. In TypeScript, developers can define types for variables, function parameters, and return values, which helps catch errors during development and enhances code readability.
1.2. Benefits of TypeScript
- Type Safety: TypeScript enforces strict typing, reducing the chances of runtime errors and making code more reliable.
- Tooling Support: TypeScript offers excellent tooling, including autocompletion, refactoring, and debugging features in popular code editors.
- Readability: Type annotations make code more self-documenting, making it easier for developers to understand and maintain.
- Large Ecosystem: TypeScript seamlessly integrates with JavaScript libraries and frameworks, allowing developers to leverage existing codebases.
2. Natural Language Generation (NLG)
2.1. What is NLG?
Natural Language Generation (NLG) is a branch of artificial intelligence (AI) that focuses on generating human-like text from structured data. NLG systems analyze data and use predefined templates or rules to create coherent and contextually relevant narratives. NLG has found applications in various domains, including content generation, automated reporting, chatbots, and more.
2.2. Applications of NLG
- Content Generation: NLG can automatically generate articles, product descriptions, and marketing content tailored to specific audiences.
- Data Reporting: NLG can convert data into human-readable reports, simplifying complex information for stakeholders.
- Chatbots: NLG-powered chatbots can engage users in natural conversations and provide personalized responses.
- Personalized Messaging: NLG can be used to create customized emails, notifications, and messages based on user preferences and behaviors.
3. The Synergy of TypeScript and NLG
3.1. TypeScript’s Role in NLG
TypeScript plays a crucial role in enhancing NLG capabilities in several ways:
- Type Safety for Data: NLG relies heavily on structured data. TypeScript’s static typing ensures that data used in NLG processes is well-defined and error-free.
- Development Productivity: TypeScript’s strong tooling support accelerates the development of NLG applications. Developers can catch type-related errors early, reducing debugging time.
- Intelligent Code Completion: TypeScript’s code editors offer intelligent autocompletion, making it easier to work with NLG libraries and APIs.
3.2. Advantages of Using TypeScript with NLG
- Enhanced Maintainability: TypeScript’s type system improves code maintainability, making it easier to understand and update NLG logic over time.
- Reduced Error Rate: TypeScript’s type checking helps identify and fix errors before runtime, ensuring that NLG-generated content is accurate and error-free.
- Seamless Integration: TypeScript can be seamlessly integrated with NLG libraries and APIs, allowing developers to harness the power of NLG while benefiting from TypeScript’s features.
4. Getting Started: NLG with TypeScript
4.1. Setting up Your Development Environment
To start building NLG-powered applications with TypeScript, you’ll need to set up your development environment. Follow these steps:
- Install Node.js and npm: Ensure you have Node.js and npm (Node Package Manager) installed on your machine.
- Create a TypeScript Project: Initialize a new TypeScript project using npm init and configure your project settings.
- Install NLG Libraries: Install NLG libraries such as GPT-3, OpenAI’s powerful language model, or other NLG APIs.
4.2. Integrating NLG Libraries
Integrating NLG libraries into your TypeScript project is straightforward. Here’s a simplified example using the OpenAI GPT-3 API:
typescript import * as openai from 'openai'; // Initialize the OpenAI API with your API key const apiKey = 'your-api-key'; const client = new openai.Client({ apiKey }); // Define a function to generate text using NLG async function generateText(prompt: string): Promise<string> { const response = await client.completions.create({ prompt, max_tokens: 100, // Adjust the maximum length of generated text }); return response.choices[0].text; } // Example usage const generatedText = await generateText('Once upon a time...'); console.log(generatedText);
This code demonstrates how to set up a basic NLG integration in TypeScript. Replace ‘your-api-key’ with your actual API key.
5. Code Samples and Examples
5.1. Building a Basic NLG-Powered Chatbot
Let’s create a simple chatbot using TypeScript and NLG. We’ll use the OpenAI GPT-3 API to generate responses based on user input.
typescript // Import necessary libraries and initialize the NLG API client async function chatbot(userInput: string): Promise<string> { const prompt = `User: ${userInput}\nChatbot:`; const response = await generateText(prompt); // Extract the chatbot's response const chatbotResponse = response.split('Chatbot:')[1].trim(); return chatbotResponse; } // Example usage const userMessage = 'Tell me a joke!'; const botResponse = await chatbot(userMessage); console.log(`User: ${userMessage}\nChatbot: ${botResponse}`);
This code snippet demonstrates how to create a basic NLG-powered chatbot using TypeScript and NLG libraries.
5.2. Generating Dynamic Reports with TypeScript and NLG
Imagine you need to generate weekly sales reports from a dataset. TypeScript and NLG can automate this process efficiently.
typescript // Import necessary libraries and initialize the NLG API client interface SalesData { week: number; revenue: number; unitsSold: number; } async function generateSalesReport(data: SalesData[]): Promise<string> { let report = 'Weekly Sales Report\n\n'; for (const entry of data) { report += `Week ${entry.week}:\n`; report += `Revenue: ${entry.revenue}\n`; report += `Units Sold: ${entry.unitsSold}\n\n`; } const response = await generateText(report); return response; } // Example usage const salesData = [ { week: 1, revenue: 5000, unitsSold: 100 }, { week: 2, revenue: 6000, unitsSold: 120 }, // Add more data entries as needed ]; const salesReport = await generateSalesReport(salesData); console.log(salesReport);
This code showcases how TypeScript can be used to generate dynamic reports with NLG, making it easy to automate data-driven document creation.
6. Challenges and Considerations
6.1. Handling NLG Errors and Exceptions
NLG systems may occasionally produce unexpected or incorrect output. It’s essential to implement error handling and validation mechanisms to address such issues gracefully.
6.2. Scalability and Performance
As your NLG-powered applications grow, scalability and performance become crucial considerations. Ensure your architecture can handle increased workloads efficiently.
Conclusion
The fusion of TypeScript and Natural Language Generation is a compelling synergy that opens doors to innovative text generation solutions. TypeScript’s type safety, development productivity, and strong tooling support, when combined with NLG’s ability to generate human-like text, provide developers with a potent toolkit for creating AI-powered applications.
By following the steps outlined in this blog post and exploring the provided code samples, you can embark on your journey to harness the power of TypeScript and NLG to automate content generation, enhance user interactions, and revolutionize the way text is created in your applications. As the fields of TypeScript and NLG continue to evolve, the possibilities for AI-powered text generation are limitless, and your creativity is the only boundary.
Table of Contents
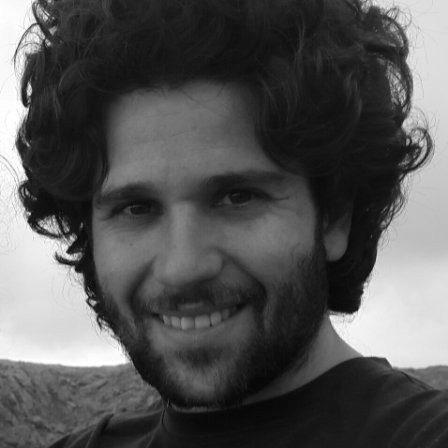
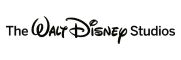