Secure Coding in TypeScript: Common Pitfalls and Solutions
In today’s rapidly evolving digital landscape, security is a paramount concern for developers. As more and more applications are built using TypeScript, a statically typed superset of JavaScript, understanding secure coding practices becomes essential to protect sensitive data and ensure the integrity of your software. In this blog post, we will delve into the world of secure coding in TypeScript, highlighting common pitfalls that developers might encounter and providing effective solutions to mitigate these issues.
Table of Contents
1. Introduction to Secure Coding in TypeScript
1.1. The Importance of Secure Coding
Secure coding is the practice of writing software in a way that prevents security vulnerabilities and protects against potential threats. In the context of TypeScript, secure coding involves understanding the nuances of the language, identifying potential vulnerabilities, and implementing preventive measures. The repercussions of neglecting secure coding can be severe, leading to data breaches, unauthorized access, and compromised user privacy.
1.2. Benefits of Using TypeScript for Security
TypeScript offers several features that can enhance the security of your codebase:
- Static Typing: TypeScript’s static typing helps catch type-related errors at compile-time, reducing the chances of runtime vulnerabilities caused by type mismatches or incorrect data handling.
- Code Analysis: TypeScript’s tooling and static analysis capabilities can identify potential security issues before runtime, helping developers catch vulnerabilities early in the development process.
- Readability: TypeScript’s explicit and self-documenting syntax can make your codebase more understandable, enabling other developers to review and spot security issues more easily.
2. Common Security Pitfalls in TypeScript Applications
2.1. Cross-Site Scripting (XSS)
Cross-Site Scripting is a widespread vulnerability where malicious code is injected into a web application, often through user-generated content. This code can execute within the user’s browser, potentially stealing sensitive information or performing actions on behalf of the user without their consent.
Solution:
Sanitize user input and utilize security libraries like DOMPurify to sanitize dynamic content before rendering it in the browser. Additionally, adopt a Content Security Policy (CSP) to restrict the sources of executable scripts, mitigating the risk of XSS attacks.
typescript import DOMPurify from 'dompurify'; const userContent = '<script>maliciousCode()</script>'; const sanitizedContent = DOMPurify.sanitize(userContent); document.getElementById('content').innerHTML = sanitizedContent;
2.2. SQL Injection
SQL Injection occurs when user input is improperly sanitized and then executed as part of a database query. Attackers can manipulate the input to execute unauthorized database operations, potentially accessing, modifying, or deleting sensitive data.
Solution:
Use parameterized queries or prepared statements provided by database libraries to separate user input from the SQL query itself. This prevents user input from being treated as executable code.
typescript import { Database } from 'database-library'; const userInput = "'; DROP TABLE users; --"; const query = `SELECT * FROM users WHERE username = ?`; const results = await Database.query(query, [userInput]);
2.3. Cross-Site Request Forgery (CSRF)
CSRF attacks involve tricking a user into unknowingly making a malicious request to a different site on which they are authenticated. This can lead to unauthorized actions being performed on the user’s behalf without their consent.
Solution:
Implement Anti-CSRF tokens, also known as CSRF tokens, which are unique tokens generated for each user session. These tokens are included in forms and requests, and they are validated on the server to ensure that requests are coming from the same origin that initiated the session.
typescript // Server-side code to generate and validate CSRF tokens const csrfToken = generateCSRFToken(); app.use((req, res, next) => { if (req.method === 'POST' || req.method === 'PUT' || req.method === 'DELETE') { if (req.headers['csrf-token'] !== csrfToken) { return res.status(403).send('Invalid CSRF token'); } } next(); });
2.4. Insecure Dependencies
Insecure or outdated dependencies can introduce vulnerabilities into your application. Attackers often target known security issues in libraries that are widely used and have not been updated to fix these vulnerabilities.
Solution:
Regularly audit your project’s dependencies for security vulnerabilities using tools like npm audit or yarn audit. Keep your dependencies up to date and follow best practices for package management. Consider using tools like Snyk or Dependabot to automate dependency vulnerability monitoring.
3. Strategies and Solutions for Secure Coding
3.1. Input Validation and Sanitization
Proper input validation and sanitization are crucial to prevent various types of attacks, including XSS and SQL injection. Always validate and sanitize user input before processing it, and use libraries that offer robust validation mechanisms.
3.2. Prepared Statements and Query Builders
When interacting with databases, utilize prepared statements or query builders to parameterize your queries. This ensures that user input is treated as data and not as executable code, mitigating the risk of SQL injection.
3.3. Anti-CSRF Tokens
Implement Anti-CSRF tokens to protect against CSRF attacks. Generate unique tokens for each user session and validate them on the server before processing sensitive requests. This prevents attackers from forging requests on behalf of authenticated users.
3.4. Dependency Management and Monitoring
Regularly audit your project’s dependencies for security vulnerabilities. Keep your dependencies up to date and respond promptly to any security advisories. Consider using automated tools to monitor your dependencies and receive alerts when vulnerabilities are detected.
Conclusion
Secure coding in TypeScript is an essential skill for modern developers. By understanding common security pitfalls and adopting best practices, you can safeguard your applications from potential vulnerabilities. Whether it’s preventing XSS, defending against SQL injection, or ensuring CSRF protection, taking a proactive approach to security can save you from significant headaches down the road. Remember, security is an ongoing process, so stay vigilant, stay informed, and keep your TypeScript applications secure.
Table of Contents
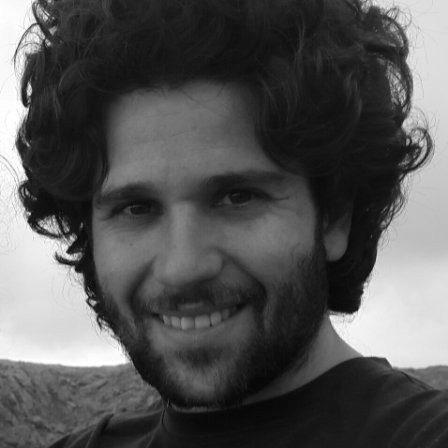
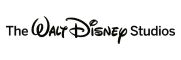