The Power of Type Safety in TypeScript
TypeScript has emerged as a popular choice among developers due to its ability to add static type checking to JavaScript. By enforcing type safety, TypeScript enables developers to catch errors early in the development process, leading to improved code quality, increased productivity, and enhanced robustness. In this blog post, we will explore the power of type safety in TypeScript and its numerous benefits, along with real-life examples and best practices for maximizing its potential.
1. Understanding Type Safety
Type safety refers to the ability of a programming language to prevent certain types of errors during compilation, thereby minimizing runtime issues. Unlike JavaScript, which is dynamically typed, TypeScript introduces static typing, where types are checked at compile time. This means that TypeScript ensures variables, parameters, and function return values adhere to the specified types, helping catch potential errors before the code is even executed.
TypeScript achieves type safety through the use of type annotations. These annotations explicitly define the expected types of variables, function arguments, and return values. For example, consider the following TypeScript function:
typescript function addNumbers(a: number, b: number): number { return a + b; }
In this example, the addNumbers function explicitly specifies that both a and b should be of type number and the function itself will return a value of type number. This type annotation ensures that only numerical values are passed to the function and that the returned result will always be a number.
2. Benefits of Type Safety in TypeScript
2.1 Enhanced Code Quality
Type safety significantly enhances code quality by catching errors at compile time. It helps eliminate common mistakes, such as passing incorrect types to functions or accessing properties that don’t exist on certain objects. The compiler acts as a safety net, preventing these errors from propagating to the runtime environment.
By catching errors early, developers can address them before they become costly and time-consuming issues. With fewer runtime errors, debugging becomes more efficient, leading to more reliable and stable code.
2.2 Increased Productivity
TypeScript’s type safety leads to increased productivity throughout the development process. With type annotations, developers get better tooling support, including autocompletion, type inference, and static analysis. These features allow for faster and more accurate development, as developers can rely on the IDE or editor to provide context-aware suggestions and catch potential issues.
Furthermore, type safety reduces the need for extensive manual testing, as many errors are caught during compilation. Developers can have greater confidence in their code changes and refactorings, allowing them to iterate and deliver new features more efficiently.
2.3 Robust Error Handling
TypeScript’s type safety helps improve error handling in applications. By catching errors at compile time, developers can prevent common mistakes that could lead to runtime exceptions or crashes. This enhances the robustness of the codebase and ensures a smoother user experience.
With TypeScript, developers can also leverage features such as union types, intersection types, and nullability checks to model more complex scenarios and handle potential errors explicitly. The compiler can guide developers in handling these cases correctly, preventing runtime issues that could otherwise occur in a dynamically typed language like JavaScript.
3. Real-Life Examples
3.1 Catching Bugs at Compile Time
Consider a scenario where a function expects a string parameter, but an integer is accidentally passed instead. In JavaScript, this would result in unexpected behavior or runtime errors. However, in TypeScript, the type checker detects this issue during compilation, preventing it from causing problems at runtime. This early detection allows developers to fix the bug before it impacts the functionality of the application.
typescript function greet(name: string) { console.log(`Hello, ${name}!`); } greet(123); // Type error: Argument of type 'number' is not assignable to parameter of type 'string'.
3.2 Refactoring with Confidence
Refactoring code can be a daunting task, especially in large codebases. However, with TypeScript’s type safety, developers can refactor their code with confidence, knowing that the compiler will flag any issues caused by the changes.
For example, let’s say we have a function that calculates the total price of items in a shopping cart:
typescript function calculateTotalPrice(items: number[]): number { return items.reduce((sum, item) => sum + item, 0); }
Later, we decide to update the function to use a different data structure, such as an array of objects, where each object represents an item with a price property. With type safety, we can make the necessary changes, and the compiler will guide us to fix any issues that arise:
typescript type Item = { price: number; }; function calculateTotalPrice(items: Item[]): number { return items.reduce((sum, item) => sum + item.price, 0); }
TypeScript helps ensure that all code paths are updated correctly, reducing the risk of introducing bugs during the refactoring process.
3.3 Collaborative Development
TypeScript shines in collaborative development scenarios, where multiple developers work on the same codebase. By providing a clear and explicit representation of types, TypeScript improves communication and reduces misunderstandings between team members. This is particularly beneficial when working on shared libraries or APIs, as the types serve as a contract that defines how different components should interact.
Type annotations and type inference make it easier for developers to understand the shape of data and function interfaces, even when working with unfamiliar code. Additionally, modern IDEs and editors provide excellent support for TypeScript, allowing developers to navigate through code, explore type definitions, and understand dependencies more efficiently.
4. Best Practices for Maximizing Type Safety
To maximize the benefits of type safety in TypeScript, consider the following best practices:
4.1 Use Explicit Types
While TypeScript provides powerful type inference capabilities, it’s generally a good practice to use explicit type annotations wherever possible. Explicitly specifying types improves code readability and helps communicate the developer’s intent effectively. It also prevents potential issues when type inference might not be able to deduce the desired type accurately.
4.2 Leverage TypeScript’s Advanced Features
TypeScript offers various advanced features that can further enhance type safety. Explore features like union types, intersection types, mapped types, conditional types, and generics to create expressive and flexible type systems. Leveraging these features can enable you to model complex scenarios and capture more precise type relationships in your code.
4.3 Strict Compiler Options
Take advantage of TypeScript’s strict compiler options to enforce stricter type checking rules. Enable options such as strictNullChecks, noImplicitAny, and strictFunctionTypes to catch more potential errors and enforce better code quality. While these options may lead to more initial work upfront, they significantly contribute to reducing bugs and improving the reliability of your codebase in the long run.
Conclusion
TypeScript’s type safety empowers developers to write more reliable, maintainable, and robust code. By catching errors at compile time, TypeScript enhances code quality, boosts productivity, and improves error handling. Real-life examples demonstrate how type safety catches bugs early, enables confident refactoring, and facilitates collaborative development.
To harness the full potential of type safety in TypeScript, follow best practices such as using explicit types, leveraging advanced features, and enabling strict compiler options. By embracing type safety, developers can unlock a new level of confidence in their code, resulting in more efficient development processes and better quality software.
Table of Contents
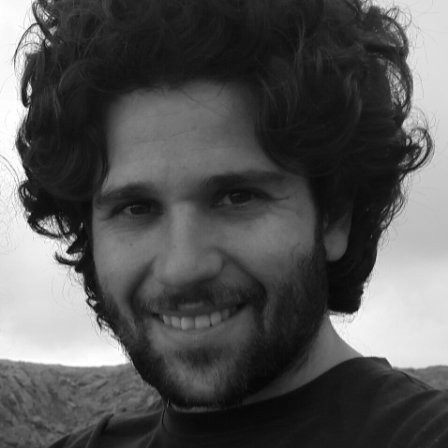
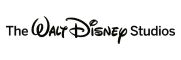