TypeScript and UI/UX Design: Creating Beautiful Interfaces
User Interface (UI) and User Experience (UX) design are essential aspects of any successful software project. Creating beautiful and functional interfaces that provide an exceptional user experience is a complex process that requires attention to detail, creativity, and efficient development practices. TypeScript, a statically typed superset of JavaScript, can be a powerful tool in achieving these goals.
Table of Contents
In this blog post, we’ll explore how TypeScript can elevate your UI/UX design by helping you create stunning interfaces and streamline your development process. We’ll cover the following topics:
1. Why TypeScript for UI/UX Design?
1.1. TypeScript: A Brief Overview
Before diving into the specifics of TypeScript in UI/UX design, let’s briefly understand what TypeScript is and why it’s gaining popularity.
TypeScript is a statically typed superset of JavaScript. It extends JavaScript by adding type annotations, which enable developers to catch errors at compile-time rather than runtime. This feature alone can significantly improve the reliability and maintainability of your codebase. In addition to type safety, TypeScript offers several other advantages:
- Better Tooling: TypeScript provides rich tooling support with features like code completion, intelligent code navigation, and refactoring assistance. This makes development faster and more efficient.
- Enhanced Collaboration: With TypeScript, teams can more easily collaborate on large codebases, as type annotations provide clear documentation and reduce ambiguity.
- Compatibility with JavaScript: TypeScript is a superset of JavaScript, meaning you can gradually adopt TypeScript in your projects and take advantage of its benefits without rewriting your entire codebase.
- Community and Ecosystem: TypeScript has a thriving community and a vast ecosystem of libraries and tools. This makes it a strong choice for building modern web applications.
Now that we understand why TypeScript is a compelling choice for web development in general, let’s delve into its specific benefits for UI/UX design.
1.2. Type Safety in UI/UX Design
1.2.1. Preventing Bugs and Errors
One of the primary advantages of TypeScript in UI/UX design is the prevention of bugs and errors that can negatively impact the user experience. When you define types for your data and components, TypeScript checks for type compatibility at compile-time, catching issues before they reach your users. This leads to more reliable and robust interfaces.
Let’s take a simple example of a button component:
typescript interface ButtonProps { label: string; onClick: () => void; } function Button(props: ButtonProps) { return ( <button onClick={props.onClick}> {props.label} </button> ); }
In this example, TypeScript ensures that the label is of type string, and onClick is a function that takes no arguments and returns void. Any deviation from these types will result in a compile-time error, preventing runtime issues.
1.2.2. Improved Codebase Maintenance
As your UI/UX design project evolves, maintaining and updating your codebase becomes crucial. TypeScript simplifies this process by providing clear type annotations. This means that even if you revisit your code after some time, you can quickly understand the types of data and components, reducing the chances of introducing breaking changes.
2. TypeScript and Component-Based UI Design
2.1. Building Reusable Components
Component-based UI design is a fundamental approach to creating user interfaces. TypeScript enhances this approach by allowing you to define interfaces for your components, making them reusable and self-documenting.
Consider a scenario where you have a user profile card component:
typescript interface UserProfileProps { username: string; bio: string; avatarUrl: string; } function UserProfile(props: UserProfileProps) { // Render user profile card using props }
By defining the UserProfileProps interface, you provide a clear contract for how this component should be used. Other developers (or even your future self) can quickly understand the expected input and output of this component.
2.2. Type-Safe Prop Passing
TypeScript ensures that you pass the correct props to your components. This not only prevents runtime errors but also improves the development experience. IDEs can provide autocompletion and type checking, making it easier to work with components in a large codebase.
typescript // Usage of UserProfile component <UserProfile username="john_doe" bio="Front-end developer" avatarUrl="john_avatar.jpg" />
Here, TypeScript ensures that you pass the required props, all of which are of the correct type.
3. Enhancing UI State Management
3.1. Typed State Management
Effective UI/UX design often involves managing the state of your application. TypeScript can help in this area by providing type-safe state management solutions. Libraries like Redux and Mobx have TypeScript support, allowing you to define strongly typed states, actions, and reducers.
typescript // Redux with TypeScript example interface AppState { user: UserProfileProps; notifications: Notification[]; } // Define actions with type safety type AppAction = { type: 'UPDATE_USER'; payload: UserProfileProps }; function rootReducer(state: AppState, action: AppAction): AppState { switch (action.type) { case 'UPDATE_USER': return { ...state, user: action.payload }; // Handle other actions here default: return state; } }
By leveraging TypeScript, you ensure that your state management code is consistent with your UI components, reducing the chances of runtime errors.
4. Streamlining Collaboration in UI/UX Design
4.1. Clearer Documentation
UI/UX design often involves collaboration among designers, developers, and product managers. TypeScript’s type annotations serve as a form of documentation that everyone can understand. This clear communication ensures that the design vision is accurately implemented in code.
typescript interface ProductCardProps { product: ProductData; onClick: () => void; }
With such clear interfaces, designers can easily grasp how components are used and make design decisions accordingly.
4.2. Collaborative Debugging
When bugs or issues arise during development, TypeScript’s type system can help identify the root cause quickly. Developers can rely on the type information to trace problems back to their source, resulting in faster debugging and issue resolution.
5. TypeScript in UI/UX: Real-World Examples
Let’s explore some real-world examples of TypeScript in UI/UX design:
5.1. TypeScript in React Native
React Native is a popular framework for building mobile apps. By using TypeScript with React Native, you can create consistent and reliable user interfaces across different platforms. TypeScript’s type checking helps catch platform-specific issues early in the development process.
5.2. TypeScript in Design Systems
Design systems are essential for maintaining visual consistency in UI/UX design. TypeScript can be used to define and enforce design system components and guidelines, ensuring that designers and developers are on the same page.
5.3. TypeScript in Data Visualization
Data visualization is a critical aspect of many applications. TypeScript can help create complex visualizations with strong type safety. Libraries like D3.js have TypeScript typings available, making it easier to work with data-driven interfaces.
6. TypeScript Tips for UI/UX Designers
As a UI/UX designer, you can benefit from TypeScript even if you’re not writing code. Here are some tips:
6.1. Understand TypeScript Basics
Familiarize yourself with TypeScript basics, such as type annotations, interfaces, and type inference. This knowledge will help you communicate effectively with developers and understand the codebase better.
6.2. Review Component Interfaces
When working with developers, review component interfaces to ensure they align with your design vision. This will help catch any discrepancies early in the development process.
6.3. Provide Feedback
If you notice inconsistencies between the design and the implemented UI, provide constructive feedback to the development team. TypeScript’s type system can help identify areas where the design and code don’t match.
Conclusion
Incorporating TypeScript into your UI/UX design workflow can significantly enhance the quality and reliability of your interfaces. Its type safety features, support for component-based design, and collaboration benefits make it a valuable tool for both designers and developers.
By leveraging TypeScript, you can create beautiful interfaces that not only meet your design vision but also provide a seamless and error-free user experience. Whether you’re designing for web, mobile, or other platforms, TypeScript is a powerful ally in your journey to creating stunning interfaces.
So, why wait? Start exploring TypeScript for UI/UX design today and elevate your projects to new heights of beauty and functionality. Your users will thank you for it!
Table of Contents
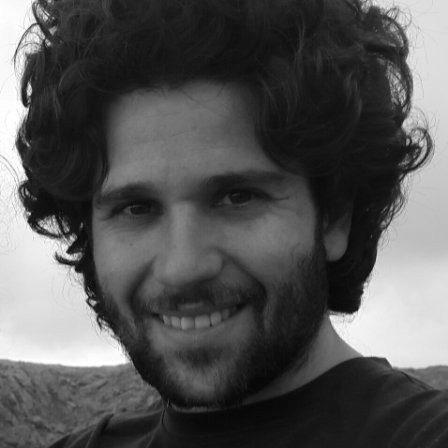
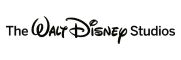