TypeScript vs. JavaScript: Choosing the Right Language
When it comes to web development, choosing the right programming language is crucial. In recent years, TypeScript has gained significant popularity as a superset of JavaScript. While both TypeScript and JavaScript share similarities, they also have distinct differences. This blog post aims to provide a comprehensive comparison of TypeScript and JavaScript, highlighting their key features, advantages, and use cases. Whether you’re a seasoned developer or a beginner, understanding the differences between these two languages will help you make an informed decision for your next project.
1. TypeScript: An Introduction
1.1 What is TypeScript?
TypeScript is a statically typed superset of JavaScript that compiles to plain JavaScript. It was developed by Microsoft and first released in 2012. TypeScript introduces optional static typing, which enables developers to write more robust and maintainable code. By leveraging a powerful type system, TypeScript provides better tooling and improved developer experience.
1.2 Advantages of TypeScript
- Static Typing: TypeScript introduces static typing, allowing developers to catch type-related errors at compile-time rather than runtime. This leads to increased code reliability and improved refactoring capabilities.
- Enhanced Tooling: TypeScript offers excellent tooling support, including autocompletion, refactoring tools, and better code navigation. Editors and IDEs can provide real-time feedback and suggestions due to the availability of type information.
- Improved Developer Experience: With static typing and advanced tooling, TypeScript provides a smoother development experience. Developers can catch potential bugs early in the development process, leading to faster development and fewer runtime errors.
1.3 Use Cases for TypeScript
- Large-Scale Applications: TypeScript excels in large-scale applications where code maintainability and reliability are critical. Its static typing and tooling support make it easier to manage complex codebases.
- Collaborative Development: TypeScript’s type system aids collaboration within development teams. It provides a clear contract between different parts of the codebase, making it easier for developers to understand and work together on projects.
2. JavaScript: An Introduction
2.1 What is JavaScript?
JavaScript is a dynamic, interpreted programming language that powers the web. It was originally designed to add interactivity to web pages but has evolved into a versatile language used for both front-end and back-end development. JavaScript is known for its flexibility, simplicity, and extensive ecosystem of libraries and frameworks.
2.2 Advantages of JavaScript
- Flexibility and Compatibility: JavaScript is supported by all major browsers, making it a reliable choice for building web applications. Its versatility allows developers to use the same language across different platforms and environments.
- Vibrant Ecosystem: JavaScript has a vast ecosystem of libraries and frameworks, such as React, Angular, and Node.js. This ecosystem provides developers with a wide range of tools and resources to build robust and feature-rich applications.
- Easy to Learn: JavaScript has a gentle learning curve, making it accessible to beginners. Its syntax is similar to other C-style languages, and there are numerous online resources and communities available for learning and getting help.
2.3 Use Cases for JavaScript
- Web Development: JavaScript is the de facto language for client-side web development. It enables developers to create interactive user interfaces and dynamic web applications.
- Server-Side Development: With the rise of Node.js, JavaScript is now widely used for server-side development. It allows developers to build scalable and efficient server applications.
3. Key Differences between TypeScript and JavaScript
3.1 Static Typing vs. Dynamic Typing
TypeScript introduces static typing, whereas JavaScript is dynamically typed. In TypeScript, variables can have explicit types, and type checking is performed at compile-time. JavaScript, on the other hand, determines types dynamically at runtime.
typescript // TypeScript let message: string = "Hello, TypeScript!"; console.log(message); // JavaScript let message = "Hello, JavaScript!"; console.log(message);
The static typing in TypeScript helps catch potential type-related errors early, while JavaScript’s dynamic typing allows for more flexibility and quicker prototyping.
3.2 Tooling and Development Experience
TypeScript provides superior tooling support compared to JavaScript. Integrated Development Environments (IDEs) and text editors can leverage TypeScript’s type information to offer autocompletion, refactoring tools, and error checking. JavaScript, although improving, doesn’t offer the same level of tooling out-of-the-box.
typescript // TypeScript function greet(name: string) { return `Hello, ${name}!`; } let message = greet("TypeScript"); // JavaScript function greet(name) { return `Hello, ${name}!`; } let message = greet("JavaScript");
In TypeScript, the IDE can provide autocompletion and type hints for the greet function parameter, resulting in a more productive development experience.
3.3 Language Features and Expressiveness
TypeScript extends JavaScript by adding additional features such as classes, interfaces, and modules. These language features enable developers to write more structured and modular code.
typescript // TypeScript interface Shape { draw(): void; } class Circle implements Shape { draw() { console.log("Drawing a circle"); } } let shape: Shape = new Circle(); shape.draw(); // JavaScript (without interfaces and classes) let shape = { draw: function() { console.log("Drawing a shape"); } }; shape.draw();
The ability to use interfaces and classes in TypeScript enhances code readability and maintainability, especially in larger codebases.
3.4 Performance and Execution
Since TypeScript compiles down to JavaScript, the performance of both languages is essentially the same. TypeScript’s static typing is only enforced at compile-time and does not introduce any runtime overhead.
TypeScript’s type annotations are erased during the compilation process, so the resulting JavaScript code is similar to what a developer would write manually.
4. Choosing the Right Language for Your Project
When deciding between TypeScript and JavaScript, consider the following factors:
4.1 Consider Your Project Requirements
- Complexity: If you’re working on a large-scale project with complex logic and a team of developers, TypeScript’s static typing and tooling support can greatly improve code maintainability and collaboration.
- Prototyping: For smaller projects or quick prototyping, JavaScript’s dynamic typing and simplicity may provide a faster development cycle.
4.2 Evaluate Your Team’s Skills and Experience
Consider the skills and experience of your development team. If they are familiar with JavaScript but haven’t worked extensively with TypeScript, the learning curve may impact the project’s timeline. However, if your team already has TypeScript expertise, using TypeScript can boost productivity and code quality.
4.3 Community Support and Ecosystem
Both TypeScript and JavaScript have vibrant communities and extensive ecosystems. Consider the availability of libraries, frameworks, and resources that align with your project’s requirements. JavaScript has a broader range of options, while TypeScript has gained significant traction and support.
4.4 Future Compatibility and Scalability
Take into account the long-term viability and scalability of your project. TypeScript’s static typing can prevent subtle bugs and make refactoring easier, resulting in more maintainable code as your project grows. JavaScript, on the other hand, remains a fundamental language of the web and enjoys widespread compatibility.
Conclusion
Choosing between TypeScript and JavaScript depends on various factors, including project requirements, team skills, and development goals. TypeScript offers static typing, enhanced tooling, and improved code maintainability, making it ideal for large-scale applications and collaborative development. JavaScript, with its simplicity, flexibility, and vast ecosystem, remains an excellent choice for web development, especially for smaller projects and rapid prototyping. By understanding the key differences and considering your specific needs, you can confidently select the language that best suits your project.
Table of Contents
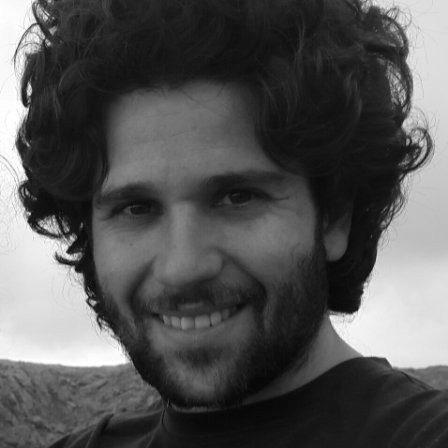
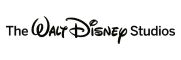