Writing Clean and Maintainable Code with TypeScript
In the ever-evolving landscape of software development, writing code isn’t just about making things work; it’s about making them work efficiently, effectively, and with longevity in mind. In this era of complex applications and collaborative development, the significance of clean and maintainable code cannot be overstated. Enter TypeScript – a superset of JavaScript that brings static typing to the language, aiding developers in catching errors early and ensuring robust code. In this blog, we will delve into the art of writing clean and maintainable code using TypeScript, exploring essential techniques, best practices, and code samples that can significantly elevate your programming skills.
Table of Contents
1. Understanding the Importance of Clean Code
1.1. What is Clean Code?
Clean code is more than just code that functions correctly. It is code that is easy to understand, read, and modify. Clean code follows established conventions and adheres to coding standards, making it comprehensible for not only the original developer but also for anyone who encounters it. It emphasizes clarity and simplicity, which in turn promotes collaboration and reduces the chances of introducing bugs during maintenance or feature additions.
1.2. Benefits of Writing Clean Code
Writing clean code yields numerous benefits:
- Enhanced Readability: Clean code is like a well-written story – it’s easy to follow and understand. This makes debugging, modification, and collaboration smoother.
- Reduced Bugs: Clean code minimizes the risk of introducing new bugs when changes are made. It’s easier to spot issues and fix them when the code is well-structured and organized.
- Efficient Collaboration: Team collaboration becomes seamless when everyone can comprehend the codebase quickly. Clean code improves team efficiency and communication.
- Time and Cost Savings: As code maintenance becomes more efficient, less time and effort are wasted on deciphering and fixing messy code. This translates to cost savings.
- Scalability: Clean code is easier to extend and adapt. When new features need to be added, a well-organized codebase facilitates the process.
2. TypeScript: A Tool for Clean Code
2.1. Advantages of TypeScript for Maintainability
TypeScript, being a statically typed superset of JavaScript, provides an array of advantages for writing clean and maintainable code:
- Static Typing: TypeScript enforces data types, catching type-related errors during development rather than at runtime. This leads to more predictable behavior and reduces runtime errors.
- Code Documentation: The use of static types inherently documents the code. Developers can understand the shape of data and function parameters without delving into the implementation details.
- IDE Support: TypeScript offers robust tooling and IDE support. With features like auto-completion, type checking, and inline documentation, developers can be more productive.
- Refactoring: With a well-typed codebase, refactoring becomes safer and easier. The compiler helps identify areas that need adjustment after changes.
2.2. Integrating TypeScript into Your Project
Integrating TypeScript into your project is relatively straightforward:
- Installation: Begin by installing TypeScript using npm or yarn: npm install typescript –save-dev
- Configuration: Create a tsconfig.json file in the root directory. This file holds compiler options and settings for your TypeScript project. You can configure target environments, output directories, and more.
- Type Definitions: If you’re using external libraries, install their type definitions to leverage TypeScript’s benefits. For example: npm install @types/react –save-dev
- Writing TypeScript: Start writing TypeScript files (.ts or .tsx). Leverage TypeScript’s static typing by explicitly declaring variable types, function parameter types, and return types.
3. Best Practices for Writing Clean and Maintainable TypeScript Code
3.1. Descriptive Naming Conventions
Choosing meaningful and consistent names for variables, functions, classes, and modules is a fundamental aspect of clean code. Descriptive names communicate intent and purpose, making the code self-explanatory. Avoid cryptic abbreviations or overly short names that require additional mental effort to decipher.
Example:
typescript // Poor Naming const a = getUserInfo(); // Improved Naming const userInfo = fetchUserInfo();
3.2. Proper Use of Data Types
TypeScript’s strength lies in its static typing. Leverage it to its full potential by using appropriate data types. This not only prevents type-related errors but also makes the code more understandable.
Example:
typescript // Without Type function calculateTax(income) { /* ... */ } // With Type function calculateTax(income: number) { /* ... */ }
3.3. Modularization and Code Organization
Breaking down your code into smaller, modular components enhances readability and maintainability. Each module should have a clear responsibility and a well-defined interface. This promotes code reuse and makes testing and debugging easier.
Example:
typescript // Poor Modularization function processUserData() { /* ... */ } function manipulateUserSettings() { /* ... */ } // Improved Modularization import { processUserData } from './user'; import { manipulateUserSettings } from './settings';
3.4. Error Handling and Logging
Effective error handling and logging contribute to code robustness. Properly handle exceptions, providing clear error messages and taking appropriate actions. Use logging to track important events and aid in debugging.
Example:
typescript try { // Code that might throw an error } catch (error) { console.error('An error occurred:', error.message); // Take necessary actions }
3.5. Regular Code Reviews
Encourage a culture of code reviews within your team. Peer reviews help catch potential issues early and ensure that code adheres to established standards. Fresh perspectives often lead to improvements and insights.
4. Ensuring Code Quality with Automated Testing
4.1. Writing Unit Tests with Jest
Automated testing is a cornerstone of maintaining code quality. Jest, a popular testing framework, pairs seamlessly with TypeScript. Write unit tests to verify individual pieces of code, ensuring they function as intended.
Example:
typescript // Math.ts export function add(a: number, b: number): number { return a + b; } // Math.test.ts import { add } from './Math'; test('addition works correctly', () => { expect(add(2, 3)).toBe(5); });
4.2. Integration of Testing into the Development Workflow
Integrate testing into your development workflow to ensure that tests are run consistently. Continuous Integration (CI) tools like Jenkins, Travis CI, or GitHub Actions can automate the testing process, providing immediate feedback on code changes.
5. Advanced Techniques for Maintainability
5.1. Dependency Injection and Inversion of Control
Implementing dependency injection and inversion of control principles promotes loose coupling between components. This enhances code modularity and allows for easier testing and replacement of components.
5.2. Design Patterns for Clean Architecture
Explore design patterns like Dependency Inversion, SOLID principles, and MVC/MVVM to structure your code in a clean and maintainable manner. These patterns guide you in separating concerns, making code more modular and adaptable.
6. Keeping Documentation Up to Date
6.1. Inline Comments and Documentation Comments
Document your code using inline comments to explain complex logic or highlight important details. Additionally, use documentation comments (TypeDoc, JSDoc) to generate comprehensive documentation that describes the purpose, usage, and inputs/outputs of functions and modules.
6.2. Generating Documentation with Tools
Utilize tools like TypeDoc or JSDoc to automatically generate documentation from your codebase. This ensures that your documentation remains up to date and accurate as the code evolves.
Conclusion
Writing clean and maintainable code with TypeScript is not just a luxury; it’s a necessity. The benefits of code that is readable, understandable, and scalable are immense. By following best practices, leveraging TypeScript’s features, incorporating testing, and exploring advanced techniques, you can create codebases that stand the test of time. Remember, the true mark of a skilled developer isn’t just in making things work, but in making them work well. So, start your journey towards producing cleaner, more maintainable code with TypeScript today. Your future self and your team will thank you for it.
Table of Contents
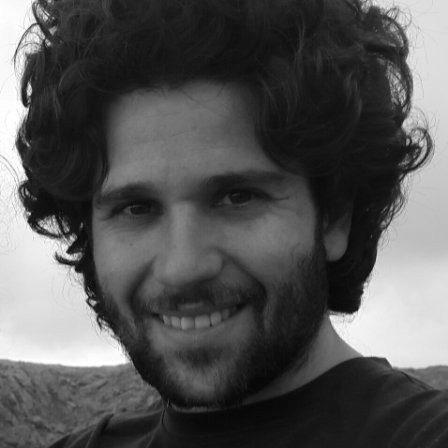
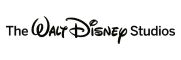