Vue.js Animation and Transitions: Adding Flair to Your User Interface
In today’s competitive digital landscape, delivering a captivating user experience is crucial for the success of any web application. Users expect a seamless and visually appealing interface that engages and delights them. Vue.js, a progressive JavaScript framework, provides powerful tools to implement animations and transitions, enabling developers to add flair and interactivity to their user interfaces.
Table of Contents
Animations and transitions breathe life into a web application, making it more user-friendly and immersive. They can be used to convey information, guide users through the interface, and enhance overall usability. In this blog, we’ll explore how to leverage Vue.js to create eye-catching animations and transitions that will elevate your app’s user experience.
1. What are Animations and Transitions?
Animations and transitions are visual effects that enhance the way elements on a web page change and move. Animations refer to dynamic, smooth, and continuous movements, while transitions are used to manage element state changes, such as when an element appears, disappears, or moves within the DOM.
By incorporating animations and transitions into your Vue.js app, you can create a more intuitive and engaging user experience. Users will find your app more enjoyable to interact with, leading to increased user retention and overall satisfaction.
2. Getting Started with Vue.js
Before diving into animations and transitions, make sure you have a basic understanding of Vue.js. If you’re new to Vue.js, here’s a quick setup to get you started:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>My Vue.js App</title> </head> <body> <div id="app"> {{ message }} </div> <script src="https://unpkg.com/vue@3.2.0/dist/vue.global.js"></script> <script> const app = Vue.createApp({ data() { return { message: 'Hello, Vue.js!' } } }); app.mount('#app'); </script> </body> </html>
3. Basic Vue.js Transitions
Vue.js provides a straightforward way to apply animations and transitions to elements using the built-in transition component. Let’s explore some basic transition examples.
3.1. Entering and Leaving Transitions
You can use the v-if directive to conditionally show or hide elements and apply transitions to them:
html <template> <div> <button @click="showBox = !showBox">Toggle Box</button> <transition name="fade"> <div v-if="showBox" class="box"></div> </transition> </div> </template> <script> export default { data() { return { showBox: false, }; }, }; </script> <style> .box { width: 100px; height: 100px; background-color: #3498db; } .fade-enter-active, .fade-leave-active { transition: opacity 0.5s; } .fade-enter-from, .fade-leave-to { opacity: 0; } </style>
In this example, we have a button that toggles the visibility of a blue box. The fade class is applied as a transition name, and CSS styles control the animation. The box will fade in and out when toggled.
3.2. Transition Classes
Vue.js applies specific CSS classes during various transition phases. The classes used in the example above are:
- fade-enter-active: Active during the entire entering phase.
- fade-leave-active: Active during the entire leaving phase.
- fade-enter-from: Applied immediately when an element begins entering (before entering phase).
- fade-leave-to: Applied immediately when an element begins leaving (before leaving phase).
You can customize these classes to create your own animations tailored to your app’s design and branding.
4. Vue’s Transition Modes
Vue.js offers three transition modes that define how elements are inserted and removed from the DOM.
4.1. In-Mode
The default mode, in-mode, allows the element to stay in the DOM during the entire transition process. It means that even if the element is not shown (via v-if), it is still present in the DOM, occupying space. Use the following example to understand the in-mode better:
html <template> <div> <button @click="toggle">Toggle Box</button> <transition name="fade" mode="in-out"> <div v-if="showBox" class="box"></div> </transition> </div> </template> <script> export default { data() { return { showBox: true, }; }, methods: { toggle() { this.showBox = !this.showBox; }, }, }; </script>
In the above example, the mode=”in-out” attribute is used to specify the in-mode. The box remains in the DOM during the entire transition process, causing a space constraint when hidden.
4.2. Out-Mode
In the out-mode, elements are removed from the DOM immediately when they leave. This means that when an element is not shown (via v-if), it is removed from the DOM, and space is freed up. Use the following example to understand the out-mode better:
html <template> <div> <button @click="toggle">Toggle Box</button> <transition name="fade" mode="out-in"> <div v-if="showBox" class="box"></div> </transition> </div> </template> <script> export default { data() { return { showBox: true, }; }, methods: { toggle() { this.showBox = !this.showBox; }, }, }; </script>
In this example, the mode=”out-in” attribute specifies the out-mode. The box is removed from the DOM when hidden, freeing up space.
4.3. In-Out Mode
Lastly, the in-out mode lets the leaving element stay in the DOM during the entering transition of its replacement. This mode is particularly useful when elements are replaced by others of similar size. Use the following example to understand the in-out mode better:
html <template> <div> <button @click="toggle">Toggle Box</button> <transition name="fade" mode="in-out"> <div :key="showBox" class="box" v-if="showBox"></div> </transition> </div> </template> <script> export default { data() { return { showBox: true, }; }, methods: { toggle() { this.showBox = !this.showBox; }, }, }; </script>
The :key attribute is used to bind the key of the element to showBox, ensuring that the element stays in the DOM during the transition process.
5. Animate.css Integration
Animate.css is a popular library for ready-to-use CSS animations. By integrating it with Vue.js, you can quickly apply pre-built animations to your components.
First, install Animate.css using npm or include it via CDN in your HTML:
html <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/animate.css/4.1.1/animate.min.css" />
Next, let’s apply an animation to a button in a Vue.js component:
html <template> <div> <button @click="animateButton" class="animate__animated">Click Me!</button> </div> </template> <script> export default { methods: { animateButton() { // Add the animate.css class to apply the animation const button = document.querySelector('button'); button.classList.add('animate__rubberBand'); // Remove the class after the animation is complete button.addEventListener('animationend', () => { button.classList.remove('animate__rubberBand'); }); }, }, }; </script>
In this example, we’re using the animate__rubberBand class from Animate.css to apply a rubber band animation to the button when it’s clicked. The animationend event listener ensures that we remove the animation class after the animation finishes, allowing the animation to be triggered again later.
Integrating Animate.css with Vue.js gives you access to a wide range of pre-made animations that can easily be added to your components, adding a touch of professionalism and flair to your user interface.
6. Custom Animations with Keyframes
While Animate.css provides a great set of pre-built animations, you may want to create your own custom animations tailored to your application’s unique style. CSS keyframes allow you to define complex animations with complete control over each animation step.
Let’s create a simple custom animation for a card component:
html <template> <div class="card" @click="animateCard"></div> </template> <script> export default { methods: { animateCard() { const card = document.querySelector('.card'); card.style.animation = 'spin 1s ease-in-out'; // Remove the animation property after the animation is complete card.addEventListener('animationend', () => { card.style.animation = ''; }); }, }, }; </script> <style> .card { width: 200px; height: 150px; background-color: #f39c12; cursor: pointer; } @keyframes spin { 0% { transform: rotate(0deg); } 100% { transform: rotate(360deg); } } </style>
In this example, clicking on the card triggers a custom spin animation defined with CSS keyframes. The animation property is applied to the card element, and we use the animationend event to remove the animation property after the animation finishes.
By using CSS keyframes, you have the flexibility to create unique, engaging animations that match your application’s aesthetics and personality.
7. The Transition Component
In previous examples, we used the <transition> component to apply animations and transitions to elements directly in the template. However, you can also create reusable transitions using the <transition> component.
7.1. Transition Events
The <transition> component emits various events during different phases of the transition. You can use these events to trigger additional actions when an animation starts or finishes.
html <template> <div> <button @click="toggleBox">Toggle Box</button> <transition name="fade" @enter="onEnter" @after-enter="onAfterEnter"> <div v-if="showBox" class="box"></div> </transition> </div> </template> <script> export default { data() { return { showBox: false, }; }, methods: { toggleBox() { this.showBox = !this.showBox; }, onEnter(el) { // Do something when the element starts entering }, onAfterEnter(el) { // Do something when the entering transition is complete }, }, }; </script>
In this example, we’re using the @enter and @after-enter event handlers to perform custom actions when the element starts entering and when the entering transition is complete, respectively. You can use these events to synchronize animations with other actions in your application.
7.2. Transitioning Multiple Elements
The <transition> component can also be used to transition multiple elements as a group. By wrapping the elements in a container and applying the transition to the container, all elements inside the container will be animated together.
html <template> <div> <button @click="toggleList">Toggle List</button> <transition-group name="fade"> <div v-if="showList" v-for="item in items" :key="item" class="item"> {{ item }} </div> </transition-group> </div> </template> <script> export default { data() { return { showList: false, items: ['Item 1', 'Item 2', 'Item 3'], }; }, methods: { toggleList() { this.showList = !this.showList; }, }, }; </script> <style> .item { margin: 10px; padding: 10px; background-color: #2ecc71; } </style>
In this example, we have a list of items that fade in and out together when the “Toggle List” button is clicked. The <transition-group> component is used to wrap the list items, and the v-for directive iterates through the items array.
8. Advanced Animation Techniques
While the examples so far have covered basic animation and transition scenarios, Vue.js offers advanced techniques to fine-tune and control animations for more sophisticated user experiences.
8.1. Staggering Animations
Staggering animations can add a polished touch to your UI by animating elements sequentially. Vue.js makes it easy to create staggered animations using the v-if directive and a computed property.
html <template> <div> <button @click="animateItems">Animate Items</button> <div v-for="(item, index) in animatedItems" :key="index" class="item"> {{ item }} </div> </div> </template> <script> export default { data() { return { items: ['Item 1', 'Item 2', 'Item 3', 'Item 4', 'Item 5'], animatedItems: [], }; }, methods: { animateItems() { this.animatedItems = []; this.items.forEach((item, index) => { setTimeout(() => { this.animatedItems.push(item); }, 200 * index); }); }, }, }; </script> <style> .item { margin: 10px; padding: 10px; background-color: #9b59b6; color: #fff; } </style>
In this example, when the “Animate Items” button is clicked, the animateItems method is called. It clears the animatedItems array and then pushes each item into it with a slight delay between each addition, creating a staggered animation effect.
8.2. Conditional Transitions
In some cases, you may want to apply different transitions based on certain conditions, such as when an element is added, removed, or replaced. Vue.js allows you to achieve this using the v-bind directive to dynamically set the name attribute of the <transition> component.
html <template> <div> <button @click="toggle">Toggle Box</button> <transition :name="transitionName"> <div v-if="showBox" class="box"></div> </transition> </div> </template> <script> export default { data() { return { showBox: false, transitionName: 'fade', }; }, methods: { toggle() { this.showBox = !this.showBox; this.transitionName = this.showBox ? 'fade' : 'slide'; }, }, }; </script> <style> .box { width: 100px; height: 100px; background-color: #3498db; } .fade-enter-active, .fade-leave-active { transition: opacity 0.5s; } .fade-enter-from, .fade-leave-to { opacity: 0; } .slide-enter-active, .slide-leave-active { transition: transform 0.5s; } .slide-enter-from, .slide-leave-to { transform: translateX(-100%); } </style>
In this example, we have two CSS classes, fade and slide, each defining a different transition. The transitionName data property is used to dynamically switch between the two transition classes based on the showBox state.
8.3. Grouping Animations
Sometimes, you may want to animate multiple elements together as a group. Vue.js allows you to wrap elements in a <transition-group> component to achieve this effect.
html <template> <div> <button @click="shuffle">Shuffle Items</button> <transition-group name="fade"> <div v-for="(item, index) in shuffledItems" :key="index" class="item"> {{ item }} </div> </transition-group> </div> </template> <script> export default { data() { return { items: ['A', 'B', 'C', 'D', 'E'], shuffledItems: ['A', 'B', 'C', 'D', 'E'], }; }, methods: { shuffle() { this.shuffledItems = this.items.sort(() => Math.random() - 0.5); }, }, }; </script> <style> .item { margin: 10px; padding: 10px; background-color: #e74c3c; color: #fff; } </style>
In this example, we have a list of items that are shuffled when the “Shuffle Items” button is clicked. The <transition-group> component ensures that all elements inside it are animated together when the order changes.
9. Performance Optimization
As animations and transitions can be resource-intensive, especially on mobile devices, it’s essential to optimize your animations for a smooth user experience.
9.1. Transition Modes
As mentioned earlier, choosing the appropriate transition mode (in-mode, out-mode, or in-out-mode) is crucial for managing DOM elements efficiently. Consider the context and behavior of your components to decide which mode best fits your needs.
9.2. Hardware Acceleration
Hardware acceleration is a technique that leverages the GPU (Graphics Processing Unit) to perform animations, making them smoother and more performant. To enable hardware acceleration for your animations, ensure that you use CSS properties that are GPU-accelerated, such as transform and opacity, instead of properties like left, top, or width, which can cause layout recalculations and affect performance.
9.3. Debouncing
Debouncing is a technique that prevents animations from triggering multiple times in quick succession, reducing unnecessary computations and rendering. For example, when animating elements based on user input (e.g., scrolling or typing), you can use a debounce function to ensure the animation only fires once within a specified interval.
Conclusion
Vue.js provides an impressive set of tools to add flair and interactivity to your user interface through animations and transitions. From simple fade-ins and fade-outs to custom keyframe animations, you have the flexibility to create engaging and delightful experiences for your users. By optimizing performance and leveraging advanced animation techniques, you can ensure your app feels smooth and professional.
Remember, the key to successful animations is moderation. Overusing animations can be distracting and frustrating for users. Instead, focus on subtle yet effective animations that enhance the user experience without overwhelming them.
As you experiment with Vue.js animations and transitions, keep testing and iterating to find the perfect balance that aligns with your app’s branding and user preferences. With a bit of creativity and thoughtful implementation, you’ll have an eye-catching user interface that captivates your users and keeps them coming back for more. Happy animating!
Table of Contents
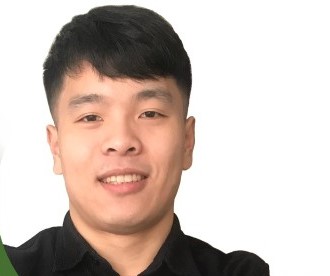
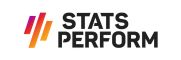