Vue.js and Progressive Web Apps: Building Offline-First Experiences
Progressive Web Apps (PWAs) have revolutionized web development by offering fast, reliable, and engaging user experiences. One key aspect of PWAs is their ability to function offline or under poor network conditions, ensuring users can access content anytime, anywhere. Vue.js, a popular JavaScript framework, provides a robust foundation for building interactive and reactive user interfaces. By combining Vue.js and the principles of Progressive Web Apps, developers can create Offline-First Experiences that deliver seamless user interactions even without an internet connection.
Table of Contents
In this blog, we’ll explore how Vue.js and Progressive Web Apps complement each other and how you can leverage their unique features to build responsive, offline-first web applications. We’ll cover various techniques and best practices, along with code samples, to help you get started on your journey towards creating exceptional offline-first experiences.
1. Understanding Progressive Web Apps
1.1. What are Progressive Web Apps?
Progressive Web Apps are web applications that leverage modern web technologies to provide an app-like experience to users. They are designed to work on any device or browser and can be installed directly from the web browser, just like native mobile applications. PWAs offer features such as offline support, push notifications, and background synchronization, enhancing user engagement and retention.
1.2. Why Choose Progressive Web Apps?
Offline Support: PWAs can function without an internet connection, ensuring uninterrupted access to content, and providing users with a seamless experience, even in areas with limited or no connectivity.
- Fast Loading: Progressive Web Apps are optimized for speed, offering quick load times and smooth interactions, which significantly impacts user satisfaction and retention.
- Engaging User Experience: PWAs provide an immersive, app-like experience with smooth animations and intuitive user interfaces, enhancing user engagement and making them more likely to return.
- Cross-platform Compatibility: Unlike native apps that require separate development for each platform, PWAs are platform-agnostic, allowing developers to reach a wider audience with a single codebase.
2. Building with Vue.js
2.1. Why Choose Vue.js?
Vue.js has gained immense popularity due to its simplicity, reactivity, and seamless integration with other tools and libraries. Some of the reasons developers prefer Vue.js are:
- Reactivity: Vue.js utilizes a reactive data binding system, making it easy to manage and update the state of the application without directly manipulating the DOM.
- Component-Based Architecture: Vue.js follows a component-based architecture, enabling developers to create reusable, self-contained UI components, leading to cleaner and more maintainable code.
- Performance: Vue.js is lightweight and offers excellent performance, making it ideal for building high-performance web applications, even on low-end devices.
- Large Ecosystem: Vue.js has a vast and active community, providing a rich ecosystem of plugins, extensions, and supporting libraries that speed up development.
2.2. Getting Started with Vue.js
Before we dive into building Progressive Web Apps with Vue.js, let’s set up a basic Vue.js application to get a better understanding of its structure:
HTML: index.html
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>My Vue.js App</title> </head> <body> <div id="app"> <!-- Your Vue.js components will be mounted here --> </div> <script src="https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js"></script> <script src="app.js"></script> </body> </html>
JavaScript: app.js
javascript // Create a new Vue instance new Vue({ el: '#app', data: { message: 'Hello, Vue.js!', }, });
In this example, we’ve created a simple Vue instance that displays the message “Hello, Vue.js!” on the page. This demonstrates the basic setup required to get started with Vue.js. Now let’s move on to building an offline-first experience with Progressive Web Apps.
3. Building Offline-First Experiences
3.1. Setting Up a Service Worker
One of the key components of a Progressive Web App is the service worker, a script that runs in the background and handles various tasks, such as caching assets, intercepting network requests, and providing offline support.
JavaScript: service-worker.js
javascript // Cache names const staticCacheName = 'app-static-v1'; const dynamicCacheName = 'app-dynamic-v1'; // Files to cache const assets = [ '/', '/index.html', '/app.js', '/styles.css', // Add other static assets like images, fonts, etc. ]; // Install event self.addEventListener('install', (event) => { event.waitUntil( caches.open(staticCacheName) .then((cache) => cache.addAll(assets)) ); });
In this code snippet, we create a service worker that caches the static assets of our application during the installation phase. The service worker intercepts network requests and serves cached assets when the user is offline, ensuring the app remains functional even without an internet connection.
3.2. Offline Fallback Page
While caching assets improves offline support, it’s essential to provide a fallback page for scenarios where a requested page isn’t cached. We can achieve this by modifying the service worker to intercept fetch requests and return cached pages in case of network failures.
JavaScript: service-worker.js (continued)
javascript // Fetch event self.addEventListener('fetch', (event) => { event.respondWith( caches.match(event.request) .then((cacheResponse) => { return cacheResponse || fetch(event.request) .then((fetchResponse) => { return caches.open(dynamicCacheName) .then((cache) => { cache.put(event.request.url, fetchResponse.clone()); return fetchResponse; }); }); }).catch(() => { return caches.match('/offline.html'); }) ); });
In this snippet, we handle the fetch event by trying to find a cached response for the requested resource. If it exists, we serve the cached version; otherwise, we fetch it from the network and store it in the dynamic cache for future use. If both cache and network fail, the service worker will return the offline fallback page, ‘/offline.html’.
3.3. Adding Offline Support to Vue Components
To ensure that our Vue.js components work offline, we need to implement logic that handles offline scenarios gracefully. For instance, if a component relies on data fetched from a server, we should display cached data or show a placeholder when the user is offline.
Vue Component: Example.vue
html <template> <div> <h1>{{ title }}</h1> <p v-if="isOffline">You are currently offline. Some data may not be up-to-date.</p> <ul v-if="data.length > 0"> <li v-for="item in data" :key="item.id">{{ item.name }}</li> </ul> <p v-else>No data available. Please check your internet connection.</p> </div> </template> <script> export default { data() { return { title: 'My Offline-First Component', data: [], isOffline: false, }; }, created() { // Fetch data from the server this.fetchData(); // Check if the user is offline window.addEventListener('offline', this.handleOffline); window.addEventListener('online', this.handleOnline); }, destroyed() { window.removeEventListener('offline', this.handleOffline); window.removeEventListener('online', this.handleOnline); }, methods: { fetchData() { // Fetch data from the server and update the 'data' array // ... }, handleOffline() { this.isOffline = true; }, handleOnline() { this.isOffline = false; // Refresh data when the user comes back online this.fetchData(); }, }, }; </script>
In this Vue component example, we display a list of items fetched from the server. If the user is offline, we show a message indicating that some data may not be up-to-date. When the user comes back online, the component fetches fresh data from the server. By handling online and offline events, we keep our app in sync with the user’s network status.
4. Optimizing Progressive Web Apps with Vue.js
4.1. Using Vue Router with Service Worker
Vue Router is a powerful tool for managing application navigation in Vue.js. When combined with a service worker, we can ensure that all routes of our Vue.js app are cached and accessible offline.
JavaScript: service-worker.js (updated)
javascript // ... // Install event self.addEventListener('install', (event) => { event.waitUntil( caches.open(staticCacheName) .then((cache) => cache.addAll(assets)) .then(() => { self.skipWaiting(); // Activate the service worker immediately }) ); }); // Activate event self.addEventListener('activate', (event) => { event.waitUntil( caches.keys() .then((cacheNames) => { return Promise.all( cacheNames .filter((name) => name !== staticCacheName && name !== dynamicCacheName) .map((name) => caches.delete(name)) ); }) .then(() => { self.clients.claim(); // Take control of all open client pages }) ); }); // …
In the updated service worker code, we have added an activate event listener that allows the service worker to take control of all open client pages. This ensures that the updated service worker and cached assets are used immediately, even if the previous service worker is still running.
4.2. Lazy Loading Components
Lazy loading is a technique to optimize web apps by loading components or resources only when they are needed. Vue.js makes lazy loading easy with dynamic imports.
Vue Component: LazyLoadComponent.vue
html <template> <div> <!-- Content of the lazy-loaded component --> </div> </template> <script> export default { // Lazy loading the component // This component will be loaded only when it is needed component: () => import('./LazyLoadComponent.vue'), }; </script>
By lazy loading components, we reduce the initial load time of our Vue.js app, making it more performant. This approach is especially valuable in Progressive Web Apps, as it reduces the time it takes for the app to become interactive, even on slow networks.
5. Push Notifications in Vue.js PWAs
Push notifications are a powerful tool to engage users and bring them back to your app. They allow you to deliver real-time updates, news, or personalized content to users’ devices.
5.1. Adding Push Notifications to a Vue.js PWA
To enable push notifications in our PWA, we need to:
- Request permission from the user to send push notifications.
- Register a service worker to handle push events.
- Implement a server to send push notifications to the subscribed clients.
JavaScript: main.js (Vue.js application entry point)
javascript // ... if ('Notification' in window && 'serviceWorker' in navigator) { Notification.requestPermission().then((permission) => { if (permission === 'granted') { // Service worker registration navigator.serviceWorker.register('service-worker.js') .then((registration) => { // Subscription to push manager return registration.pushManager.subscribe({ userVisibleOnly: true, applicationServerKey: 'your_public_key', // Get this key from your server }); }) .then((subscription) => { // Send the subscription details to the server // For example, using Axios or Fetch API // axios.post('/api/save-subscription', subscription); }) .catch((error) => { console.error('Service worker registration failed:', error); }); } }); } // …
In this example, we request notification permission from the user and register the service worker. Upon successful registration, we subscribe to the push manager and obtain a subscription object containing endpoint information. This subscription data can then be sent to the server, allowing it to send push notifications to the subscribed clients.
6. Sending Push Notifications from the Server
To send push notifications from the server, you need a server capable of sending Web Push notifications. You can use libraries like web-push in Node.js to accomplish this.
6.1. Node.js Server: send-push-notification.js
javascript const webPush = require('web-push'); // Replace with your own VAPID keys (see web-push documentation) const vapidKeys = { publicKey: 'your_public_key', privateKey: 'your_private_key', }; webPush.setVapidDetails('mailto:your@email.com', vapidKeys.publicKey, vapidKeys.privateKey); const subscription = { endpoint: 'https://example.com/your-push-endpoint', keys: { auth: 'your_push_auth_key', p256dh: 'your_push_p256dh_key', }, }; const payload = JSON.stringify({ title: 'Hello from Vue.js PWA!', body: 'You just received a push notification!', }); webPush.sendNotification(subscription, payload) .then(() => console.log('Push notification sent successfully!')) .catch((error) => console.error('Error sending push notification:', error));
In this server-side code, we use the web-push library to send a push notification to a specific subscription. The server must store the subscription information and use it to deliver push notifications to users’ devices whenever necessary.
Conclusion
Building Offline-First Experiences with Vue.js and Progressive Web Apps provides a powerful combination of reactivity, performance, and offline support. Vue.js simplifies the development of reactive user interfaces, while Progressive Web Apps ensure seamless experiences, even in offline scenarios. By combining these technologies and employing best practices, developers can create modern, fast, and reliable web applications that engage users and keep them coming back.
Remember to optimize your Vue.js Progressive Web App by using lazy loading, caching static assets, and implementing push notifications thoughtfully. Always prioritize the user experience, considering various network conditions and delivering content that remains accessible and engaging, even without an internet connection.
Happy coding and enjoy building amazing Offline-First Experiences with Vue.js and Progressive Web Apps!
Table of Contents
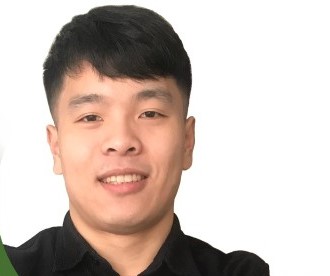
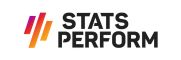