Optimizing SEO with Vue.js: Best Practices for Search Engine Visibility
In today’s digital age, Search Engine Optimization (SEO) plays a crucial role in driving organic traffic to websites. As web developers, it’s essential to understand how to optimize our Vue.js applications for better search engine visibility. While Vue.js is a powerful front-end framework that enables dynamic and interactive web applications, it presents some challenges for search engines to index and rank effectively.
Table of Contents
In this blog, we’ll delve into the best practices to enhance the SEO of your Vue.js applications, improving their chances of ranking higher on search engine results pages (SERPs). We’ll explore various techniques, including server-side rendering, meta tags optimization, lazy loading, and more, to ensure your Vue.js app gets the attention it deserves from search engines.
1. Implement Server-Side Rendering (SSR)
By default, Vue.js applications are client-side rendered, meaning the initial HTML content is minimal and often blank, making it less friendly for search engine crawlers. To improve SEO, consider implementing Server-Side Rendering (SSR) using frameworks like Nuxt.js.
SSR Code Sample:
javascript // nuxt.config.js module.exports = { mode: 'universal', /* ** Add serverMiddleware to handle SSR */ serverMiddleware: ['~/server-middleware/render'], }; javascript // server-middleware/render.js const { createRenderer } = require('vue-server-renderer'); const template = require('fs').readFileSync('index.html', 'utf-8'); const renderer = createRenderer({ template }); module.exports = (req, res) => { const context = { url: req.url }; renderer.renderToString(context, (err, html) => { if (err) { console.error(err); res.status(500).end('Internal Server Error'); return; } res.end(html); }); };
SSR generates the initial HTML content on the server-side and sends it to the client, ensuring search engines can index the content accurately. This significantly improves the SEO of your Vue.js app and positively impacts rankings.
2. Optimize Meta Tags
Meta tags provide essential information about your web page to search engines and social media platforms when your page is shared. Properly optimizing meta tags is crucial for SEO.
Meta Tags Code Sample:
html <!-- Inside the head tag of your Vue.js components --> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>My Awesome Vue.js App</title> <meta name="description" content="A concise and compelling description of your page's content"> <meta name="keywords" content="your, keywords, here"> <meta property="og:title" content="My Awesome Vue.js App"> <meta property="og:description" content="A concise and compelling description of your page's content"> <meta property="og:image" content="url-to-your-image"> <meta property="og:url" content="https://www.example.com"> <meta property="og:type" content="website"> <meta name="twitter:card" content="summary_large_image"> </head>
Replace the values in the content attribute with appropriate information relevant to your Vue.js app. The meta tags help search engines understand your page’s content and increase the likelihood of it being displayed correctly in search results.
3. Manage Dynamic Routes
Vue.js excels in creating single-page applications (SPAs) with dynamic routes. However, SPA’s dynamic nature can lead to challenges in SEO, as search engines might not effectively crawl and index these dynamic pages.
To handle dynamic routes effectively, use the “Dynamic Import” feature of Vue.js to lazily load components for each route. This approach ensures that only the necessary content is loaded, which improves the overall performance and crawlability of your app.
Dynamic Route Code Sample:
javascript // Inside your router configuration const router = new VueRouter({ routes: [ { path: '/blog/:postId', component: () => import('./components/BlogPost.vue'), }, // Other routes... ], });
By implementing dynamic imports, you improve the efficiency of your Vue.js app and provide search engines with content that is easier to crawl and index.
4. Generate a Sitemap
A sitemap is a file that lists all the pages on your website, helping search engine crawlers discover and index your content effectively. Generating and submitting a sitemap to popular search engines like Google and Bing is an essential step in optimizing the SEO of your Vue.js app.
Example Sitemap:
xml <?xml version="1.0" encoding="UTF-8"?> <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>https://www.example.com/</loc> <lastmod>2023-07-15</lastmod> <changefreq>daily</changefreq> <priority>1.0</priority> </url> <url> <loc>https://www.example.com/about</loc> <lastmod>2023-07-14</lastmod> <changefreq>weekly</changefreq> <priority>0.8</priority> </url> <!-- Add more URLs here --> </urlset>
Ensure that your sitemap is regularly updated to reflect any changes or new content on your Vue.js app. Submitting an up-to-date sitemap to search engines enhances the crawlability of your site and improves its chances of being indexed quickly and accurately.
5. Utilize Schema Markup
Schema markup is structured data that you can add to your web pages to help search engines understand the content better. This markup can provide additional context and details about your Vue.js app, such as its type, author, ratings, and more.
Schema Markup Code Sample:
html <!-- Inside the head tag of your Vue.js components --> <head> <!-- Other meta tags... --> <script type="application/ld+json"> { "@context": "http://schema.org", "@type": "Article", "mainEntityOfPage": { "@type": "WebPage", "@id": "https://www.example.com" }, "headline": "My Awesome Vue.js App", "description": "A concise and compelling description of your page's content", "image": "url-to-your-image", "author": { "@type": "Person", "name": "Your Name" }, "datePublished": "2023-07-15", "dateModified": "2023-07-15", "publisher": { "@type": "Organization", "name": "Your Organization", "logo": { "@type": "ImageObject", "url": "url-to-your-logo" } } } </script> </head>
By incorporating schema markup, you provide search engines with more structured information about your Vue.js app, leading to a better understanding and higher chances of getting featured in rich snippets.
6. Optimize Page Speed
Page speed is a critical factor in both user experience and SEO. Slow-loading pages can negatively impact your search engine rankings. Optimize your Vue.js app’s performance by minimizing JavaScript and CSS files, compressing images, and enabling caching.
Code Splitting for Lazy Loading:
javascript // Inside your Vue.js component export default { // ... components: { // Lazy load heavy components HeavyComponent: () => import('./HeavyComponent.vue'), }, };
Lazy load heavy components using dynamic imports and code splitting to ensure that only the necessary resources are loaded initially. This significantly reduces the initial page load time and improves overall performance, both of which are crucial for SEO.
7. Manage Duplicate Content
When using client-side rendering in Vue.js, there’s a possibility of generating duplicate content due to multiple URLs pointing to the same content. Duplicate content can harm your search engine rankings.
To avoid this, use the “canonical” tag to indicate the preferred URL that you want search engines to index for a particular page.
Canonical Tag Code Sample:
html <!-- Inside the head tag of your Vue.js components --> <head> <!-- Other meta tags... --> <link rel="canonical" href="https://www.example.com/preferred-url"> </head>
By specifying the canonical URL, you signal to search engines which version of the content should be indexed, eliminating duplicate content issues and potential SEO penalties.
Conclusion
Optimizing the SEO of your Vue.js applications is essential to increase their search engine visibility and drive organic traffic. By following these best practices, such as implementing Server-Side Rendering, optimizing meta tags, managing dynamic routes, generating a sitemap, utilizing schema markup, optimizing page speed, and managing duplicate content, you can improve your Vue.js app’s chances of ranking higher on search engine results pages (SERPs). Keep in mind that SEO is an ongoing process, and staying up-to-date with the latest SEO trends and best practices will help you maintain a competitive edge in the digital landscape. Happy coding!
Remember, the success of SEO relies on continuous monitoring and adapting to changing algorithms and trends. Keep learning and experimenting to stay ahead in the ever-evolving world of search engine optimization.
Table of Contents
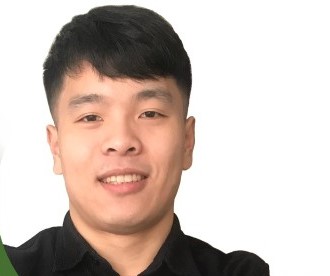
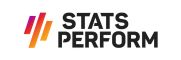