Vue.js Authentication and Authorization: Securing Your Web App
In today’s digital world, security is paramount for any web application. Whether it’s an e-commerce platform, a social media site, or an enterprise-level application, user authentication and authorization are crucial aspects of safeguarding sensitive data and providing personalized experiences. Vue.js, a progressive JavaScript framework, offers robust tools and libraries to implement secure authentication and authorization in your web app.
Table of Contents
In this blog post, we will dive into the world of Vue.js authentication and authorization, understanding the fundamental concepts, and learning how to implement them effectively. By the end of this guide, you’ll have the knowledge and skills to build a secure web app that ensures users have access to the right resources and data, and unauthorized users are kept at bay.
1. Introduction to Authentication and Authorization
Authentication and authorization are two fundamental security concepts in web applications. Authentication involves verifying the identity of a user, making sure they are who they claim to be. Once a user is authenticated, authorization comes into play. It determines what resources and actions a user is allowed to access based on their role or permission level.
For instance, in an e-commerce website, a regular customer might have access to view and purchase products, while an admin might have additional privileges to manage products and users. Vue.js makes it easy to implement these security measures using various libraries and techniques.
2. Setting Up a Vue.js Project
Before we dive into authentication and authorization, let’s set up a new Vue.js project to work with. We assume you have Node.js and npm (Node Package Manager) installed on your system. If not, please visit Node.js website to download and install the latest stable version.
To create a new Vue.js project, open your terminal and execute the following commands:
bash npm install -g @vue/cli vue create secure-web-app cd secure-web-app npm run serve
Now, your Vue.js project should be running on http://localhost:8080. Open your browser and visit the URL to see the default Vue.js welcome page.
3. User Registration and Login
3.1. Creating the Registration Form
To allow users to register on our web app, we need a registration form. In this example, we’ll keep the registration simple with just a username and password. Later, you can extend it to include additional fields like email, name, etc.
Let’s create a new Vue component for the registration form:
vue <template> <div> <h2>Registration</h2> <form @submit.prevent="registerUser"> <label for="username">Username:</label> <input type="text" id="username" v-model="username" required> <label for="password">Password:</label> <input type="password" id="password" v-model="password" required> <button type="submit">Register</button> </form> </div> </template> <script> export default { data() { return { username: '', password: '' }; }, methods: { registerUser() { // Your registration logic here } } }; </script>
In this code snippet, we’ve created a Vue component with a registration form that captures the username and password entered by the user. We’ve also added a submit event listener to the form, which will call the registerUser method when the form is submitted.
3.2. Handling User Registration
In a real-world scenario, the user registration data would be sent to a backend server for processing. For simplicity, let’s use a fake API using the JSONPlaceholder service. We’ll use the axios library to make HTTP requests.
First, install the axios library by running:
bash npm install axios
Now, let’s modify our registerUser method to send the registration data to the server:
vue <script> import axios from 'axios'; export default { // ... (previous code) methods: { async registerUser() { try { const response = await axios.post('https://jsonplaceholder.typicode.com/users', { username: this.username, password: this.password }); console.log('User registered successfully!', response.data); } catch (error) { console.error('Error registering user:', error); } } } }; </script>
With this update, when the user submits the registration form, the registerUser method will send the data to the JSONPlaceholder API. While this is a fake API and doesn’t store the data permanently, in a real-world scenario, you’d save the user information in a database.
3.3. Implementing User Login
Now that we have user registration in place, let’s implement the user login functionality. We’ll create another component for the login form and use a similar approach as we did for registration.
vue <template> <div> <h2>Login</h2> <form @submit.prevent="loginUser"> <label for="username">Username:</label> <input type="text" id="username" v-model="username" required> <label for="password">Password:</label> <input type="password" id="password" v-model="password" required> <button type="submit">Login</button> </form> </div> </template> <script> import axios from 'axios'; export default { data() { return { username: '', password: '' }; }, methods: { async loginUser() { try { const response = await axios.get('https://jsonplaceholder.typicode.com/users', { params: { username: this.username, password: this.password } }); if (response.data.length === 1) { console.log('User logged in successfully!', response.data[0]); } else { console.log('Invalid username or password.'); } } catch (error) { console.error('Error logging in:', error); } } } }; </script>
In this code snippet, we’ve created a Vue component for the login form. The loginUser method sends a GET request to the JSONPlaceholder API with the entered username and password as parameters. The API will respond with an array of users that match the given credentials. If the array length is 1, we consider the user as logged in successfully; otherwise, we display an error message.
With the registration and login forms ready, users can now create accounts and log in to your web app. However, at this point, there’s no actual authentication or authorization in place. Anyone can access any route without restrictions. In the next section, we’ll dive into JSON Web Tokens (JWT) to implement secure user authentication.
4. User Authentication with JWT
JSON Web Tokens (JWT) provide a secure and compact way to represent information between parties as a JSON object. In the context of user authentication, once a user logs in, the server will generate a JWT and send it back to the client. The client will then include this JWT in the headers of subsequent requests to prove its identity.
4.1. Understanding JSON Web Tokens (JWT)
A JWT consists of three parts separated by dots:
plaintext xxxxx.yyyyy.zzzzz
- Header: Contains metadata about the type of token and the signing algorithm used.
- Payload: Contains the claims (statements about an entity) and user data.
- Signature: Used to verify the integrity of the token and ensure it wasn’t tampered with.
The header and payload are Base64 encoded, and the signature is created by hashing the encoded header, encoded payload, and a secret key known only to the server. When the client sends the JWT in the headers of subsequent requests, the server can verify the token’s authenticity by recreating the signature using the same secret key and comparing it with the sent signature.
4.2. Generating JWT on the Server
To generate JWT on the server, you’ll need a library to handle this process. One popular choice is jsonwebtoken for Node.js. To install it, run the following command:
bash npm install jsonwebtoken
Now, let’s create a simple Express.js route to generate a JWT when a user logs in:
javascript // Import required libraries const express = require('express'); const jwt = require('jsonwebtoken'); const users = require('./users'); // Replace this with your database // Create an Express app const app = express(); // Secret key to sign JWT const secretKey = 'your_secret_key_here'; // Login route app.post('/login', (req, res) => { const { username, password } = req.body; // Replace this with your database query to fetch user data const user = users.find((u) => u.username === username && u.password === password); if (user) { // Generate JWT const token = jwt.sign({ userId: user.id, role: user.role }, secretKey, { expiresIn: '1h' }); res.json({ token }); } else { res.status(401).json({ message: 'Invalid username or password' }); } }); // Start the server const port = 3000; app.listen(port, () => console.log(`Server running on http://localhost:${port}`));
In this code snippet, we’ve created a simple Express.js server with a /login route. When a user sends a POST request with their username and password to this route, the server will look for the corresponding user in the users array (replace this with your database query). If the user is found, a JWT will be generated and sent back as the response.
4.3. Verifying and Using JWT in Vue.js
With the server generating JWT upon successful login, we now need to handle it on the Vue.js client side. For this purpose, we’ll use the vue-router library to manage routes and implement route guards to restrict access to certain routes for authenticated users only.
First, let’s install the vue-router library:
bash npm install vue-router
Next, create a new file called router.js in the src folder and configure the Vue Router:
javascript import Vue from 'vue'; import Router from 'vue-router'; import Home from './views/Home.vue'; import Login from './views/Login.vue'; import Dashboard from './views/Dashboard.vue'; Vue.use(Router); const routes = [ { path: '/', component: Home }, { path: '/login', component: Login }, { path: '/dashboard', component: Dashboard, meta: { requiresAuth: true } } ]; const router = new Router({ mode: 'history', routes }); // Route guard to check if the user is authenticated before accessing protected routes router.beforeEach((to, from, next) => { const token = localStorage.getItem('token'); if (to.matched.some((route) => route.meta.requiresAuth)) { if (token) { // User is authenticated next(); } else { // User is not authenticated; redirect to login next('/login'); } } else { // Allow access to non-protected routes next(); } }); export default router;
In this code snippet, we’ve set up three routes: / (Home), /login (Login), and /dashboard (Dashboard). The Dashboard route is protected and requires authentication to access it.
The router.beforeEach function acts as a route guard, which will be executed before each route change. If the route requires authentication (to.matched.some((route) => route.meta.requiresAuth)), the guard checks if a JWT is present in the browser’s local storage. If the token is found, the user is considered authenticated and allowed to access the protected route; otherwise, the user is redirected to the login page.
Now, let’s create the Login.vue component to handle user login and store the JWT in the browser’s local storage:
vue <template> <div> <h2>Login</h2> <form @submit.prevent="loginUser"> <label for="username">Username:</label> <input type="text" id="username" v-model="username" required> <label for="password">Password:</label> <input type="password" id="password" v-model="password" required> <button type="submit">Login</button> </form> </div> </template> <script> import axios from 'axios'; export default { data() { return { username: '', password: '' }; }, methods: { async loginUser() { try { const response = await axios.post('http://localhost:3000/login', { username: this.username, password: this.password }); if (response.data.token) { // Store the token in local storage localStorage.setItem('token', response.data.token); // Redirect to dashboard this.$router.push('/dashboard'); } else { console.log('Invalid username or password.'); } } catch (error) { console.error('Error logging in:', error); } } } }; </script>
In this code snippet, the Login.vue component handles the user login and sends a POST request to the server’s /login route. If the server responds with a JWT, it’s stored in the browser’s local storage using localStorage.setItem(‘token’, response.data.token). Then, the user is redirected to the dashboard using this.$router.push(‘/dashboard’).
The Dashboard.vue component can be a simple placeholder for now. We’ll later secure it using route guards:
vue <template> <div> <h2>Dashboard</h2> <p>Welcome to your dashboard!</p> </div> </template> <script> export default { // Add any dashboard logic or user-specific data here }; </script>
Now, when a user successfully logs in, the JWT will be stored in the local storage, and they will be redirected to the dashboard. However, the dashboard route is still not protected. In the next section, we’ll implement role-based access control (RBAC) to restrict access based on user roles.
5. Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) is a strategy that grants access to resources based on a user’s role. With RBAC, you can define different roles (e.g., admin, user, moderator) and assign different permissions to each role. Users are then assigned one or more roles, determining what they can or cannot do in the application.
In this example, we’ll implement a simple RBAC system with two roles: user and admin. The user role will have limited access to certain resources, while the admin role will have full access.
5.1. Designing User Roles and Permissions
First, let’s define the roles and their corresponding permissions. In a real-world scenario, you’d likely store this information in a database, but for simplicity, we’ll define them as constants:
javascript // roles.js const ROLES = { USER: 'user', ADMIN: 'admin' }; const PERMISSIONS = { [ROLES.USER]: ['read'], [ROLES.ADMIN]: ['read', 'write', 'delete'] }; module.exports = { ROLES, PERMISSIONS };
In this code snippet, we’ve defined two roles: user and admin. The USER role has the permission to read, while the ADMIN role has permissions to read, write, and delete.
5.2. Restricting Access Based on Roles
Now that we have roles and permissions defined, let’s update our router.js file to restrict access to the dashboard based on user roles.
javascript import Vue from 'vue'; import Router from 'vue-router'; import Home from './views/Home.vue'; import Login from './views/Login.vue'; import Dashboard from './views/Dashboard.vue'; import { ROLES } from './roles'; Vue.use(Router); const routes = [ { path: '/', component: Home }, { path: '/login', component: Login }, { path: '/dashboard', component: Dashboard, meta: { requiresAuth: true, allowedRoles: [ROLES.ADMIN] } } ]; const router = new Router({ mode: 'history', routes }); // ... (previous code) // Route guard to check if the user is authenticated and has the required role router.beforeEach((to, from, next) => { const token = localStorage.getItem('token'); if (to.matched.some((route) => route.meta.requiresAuth)) { if (token) { // Decode the JWT to get user data const decodedToken = jwt.decode(token); if (decodedToken.role === ROLES.ADMIN) { // User has the required role next(); } else { // User doesn't have the required role; redirect to home next('/'); } } else { // User is not authenticated; redirect to login next('/login'); } } else { // Allow access to non-protected routes next(); } }); export default router;
In this updated code, we’ve added an allowedRoles property to the meta field of the Dashboard route. This property holds an array of roles that are allowed to access the dashboard route. In our example, we’ve specified [ROLES.ADMIN], meaning only users with the admin role can access the dashboard.
In the router.beforeEach function, we now decode the JWT using jwt.decode(token). This provides us with the user data stored in the token, including the role. If the user’s role matches one of the allowed roles for the current route, they are granted access; otherwise, they are redirected to the home page.
6. Protecting Vue Router Routes
With the RBAC implemented, users are now restricted from accessing certain routes based on their roles. However, we need to protect these routes from being accessed directly via URL changes or by typing the route in the browser’s address bar.
We can achieve this by implementing route guards at the Vue Router level, similar to how we implemented the global route guard earlier. These guards will check the user’s role against the allowed roles for the specific route and either allow or deny access accordingly.
javascript // router.js // ... (previous code) // Route guard to check if the user is authenticated and has the required role router.beforeEach((to, from, next) => { const token = localStorage.getItem('token'); if (to.matched.some((route) => route.meta.requiresAuth)) { if (token) { // Decode the JWT to get user data const decodedToken = jwt.decode(token); if (decodedToken.role === ROLES.ADMIN) { // User has the required role next(); } else { // User doesn't have the required role; redirect to home next('/'); } } else { // User is not authenticated; redirect to login next('/login'); } } else { // Allow access to non-protected routes next(); } }); // Route guard to check if the user is allowed to access the route based on their role router.beforeEach((to, from, next) => { const allowedRoles = to.meta.allowedRoles; if (allowedRoles) { const token = localStorage.getItem('token'); if (token) { // Decode the JWT to get user data const decodedToken = jwt.decode(token); if (allowedRoles.includes(decodedToken.role)) { // User has the required role next(); } else { // User doesn't have the required role; redirect to home next('/'); } } else { // User is not authenticated; redirect to login next('/login'); } } else { // Allow access to routes without specified allowedRoles next(); } }); export default router;
In this updated code, we’ve added another route guard to check if the user is allowed to access a route based on their role. This guard is only applied to routes that have specified allowedRoles in their meta field.
With these two route guards in place, your Vue.js web app is now securely implementing authentication, authorization, and role-based access control.
7. Handling Authentication Errors
Error handling is an essential aspect of any web application, especially when it comes to authentication and authorization. Here are a few common scenarios to handle gracefully:
- Invalid Credentials: When a user enters incorrect credentials during login, you should display an error message indicating that the login attempt failed.
- Expired Token: JWTs have an expiration time defined in the exp claim. When the token expires, the user needs to log in again. You can handle this by checking the token’s expiration date and redirecting the user to the login page when necessary.
- Server Errors: If there are any server errors during authentication or authorization processes, you should display a user-friendly error message and possibly provide a way to retry the action.
Handling these errors ensures a smooth and user-friendly experience for your application’s users, enhancing its overall security and usability.
Conclusion
In this blog post, we explored the importance of authentication and authorization in securing web applications and maintaining user privacy. We learned how to implement user registration and login forms using Vue.js, along with user authentication using JSON Web Tokens (JWT). Additionally, we looked at role-based access control (RBAC) to restrict access to certain routes based on user roles.
By combining these techniques, your Vue.js web app can now offer a secure and personalized experience to your users. However, security is an ongoing process, and it’s essential to stay updated with the latest best practices and security measures to protect your application and its users from potential threats. Remember to keep your libraries and dependencies up-to-date and conduct regular security audits to identify and fix vulnerabilities.
Always prioritize security to gain the trust of your users and build a successful web application that can withstand various security challenges.
Table of Contents
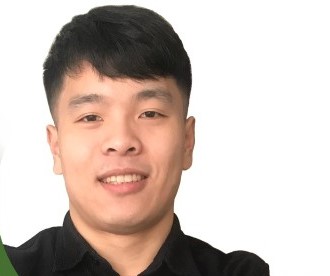
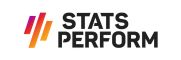