Vue.js and Firebase Integration: Building a Full-Stack Application
In today’s web development landscape, building dynamic and real-time applications is essential to meet user expectations. Combining the power of Vue.js, a popular front-end JavaScript framework, with Firebase, a real-time backend-as-a-service platform, can help developers create efficient and scalable full-stack applications. In this tutorial, we will explore the integration of Vue.js with Firebase and build a full-stack application step-by-step. We’ll cover everything from setting up Firebase to creating a real-time database, implementing authentication, and deploying the application. Let’s get started!
Table of Contents
1. Setting Up Firebase:
1.1. Creating a Firebase Project:
To start using Firebase services, you need to create a Firebase project. Go to the Firebase Console (https://console.firebase.google.com/) and click on “Add Project.” Follow the on-screen instructions to set up your project. Once your project is ready, you’ll receive a Firebase configuration object containing API keys and other essential information.
1.2. Configuring Firebase in Vue.js:
In your Vue.js project, install the Firebase SDK by running the following command:
bash npm install firebase
Then, initialize Firebase in your main application file (e.g., main.js):
javascript import firebase from 'firebase/app'; import 'firebase/firestore'; import 'firebase/auth'; const firebaseConfig = { apiKey: 'YOUR_API_KEY', authDomain: 'YOUR_PROJECT_ID.firebaseapp.com', databaseURL: 'https://YOUR_PROJECT_ID.firebaseio.com', projectId: 'YOUR_PROJECT_ID', storageBucket: 'YOUR_PROJECT_ID.appspot.com', messagingSenderId: 'YOUR_MESSAGING_SENDER_ID', appId: 'YOUR_APP_ID', }; // Initialize Firebase firebase.initializeApp(firebaseConfig); // Optional: You can also set up Firebase Authentication Persistence firebase.auth().setPersistence(firebase.auth.Auth.Persistence.LOCAL);
2. Real-Time Database with Firebase Firestore:
2.1. Creating a Firestore Database:
Firestore is Firebase’s real-time NoSQL database. To create a Firestore database, go to the Firebase Console and navigate to “Firestore Database” from the left sidebar. Click on “Create Database” and choose a location for your data.
2.2. Reading and Writing Data:
Firestore uses collections and documents to store data. Here’s an example of how to add data to Firestore:
javascript // Assuming you have already initialized Firebase const db = firebase.firestore(); // Add a new document to a collection const collectionRef = db.collection('users'); collectionRef.doc('user1').set({ name: 'John Doe', email: 'john@example.com', });
To read data from Firestore:
javascript // Get a specific document from a collection const docRef = db.collection('users').doc('user1'); docRef.get().then((doc) => { if (doc.exists) { console.log(doc.data()); } else { console.log('No such document!'); } });
2.3. Real-Time Data Sync:
One of the powerful features of Firestore is its real-time data synchronization. It allows you to listen for changes in the data and update the application automatically when data changes on the server.
javascript // Real-time data sync with Firestore collectionRef.onSnapshot((snapshot) => { snapshot.docChanges().forEach((change) => { if (change.type === 'added') { // Handle added data } if (change.type === 'modified') { // Handle modified data } if (change.type === 'removed') { // Handle removed data } }); });
3. User Authentication with Firebase Authentication:
3.1. Setting Up Firebase Authentication:
Firebase Authentication provides various authentication methods like email/password, Google, Facebook, etc. To enable authentication for your project, go to the Firebase Console, and from the left sidebar, navigate to “Authentication.” Then, follow the instructions to set up your preferred authentication providers.
3.2. Implementing User Sign Up and Login:
In your Vue.js application, you can now implement user sign-up and login functionality using Firebase Authentication:
javascript // Sign up a new user firebase.auth().createUserWithEmailAndPassword(email, password) .then((userCredential) => { // New user successfully registered const user = userCredential.user; }) .catch((error) => { // Handle sign-up errors const errorCode = error.code; const errorMessage = error.message; }); // Login an existing user firebase.auth().signInWithEmailAndPassword(email, password) .then((userCredential) => { // User successfully logged in const user = userCredential.user; }) .catch((error) => { // Handle login errors const errorCode = error.code; const errorMessage = error.message; });
3.3. Securing Routes and Authenticated Actions:
To protect certain routes and actions in your application, you can implement route guards based on the user’s authentication status:
javascript // Example of a route guard using Vue Router import { firebase } from '@firebase/app'; const router = new VueRouter(/* ... */); router.beforeEach((to, from, next) => { const requiresAuth = to.matched.some((record) => record.meta.requiresAuth); const currentUser = firebase.auth().currentUser; if (requiresAuth && !currentUser) { next('/login'); } else { next(); } });
4. Building the Vue.js Front-End:
4.1. Creating Components:
Organize your Vue.js application into components for better maintainability. Create components that represent different parts of your application, such as header, sidebar, and main content.
html <!-- Example component: Header.vue --> <template> <header> <!-- Header content goes here --> </header> </template> <script> export default { // Component logic goes here }; </script>
4.2. Implementing Real-Time Data Binding:
Vue.js provides two-way data binding, which makes it easy to update data in real-time as the user interacts with the application.
html <!-- Example: Real-time data binding --> <template> <div> <input v-model="message" type="text" /> <p>{{ message }}</p> </div> </template> <script> export default { data() { return { message: '', }; }, }; </script>
4.3 Styling the Application:
Use CSS or CSS frameworks like Bootstrap or Vuetify to style your application. Apply styles to your components to make the UI visually appealing and user-friendly.
5. Deploying the Full-Stack Application:
5.1 Preparing for Deployment:
Before deploying your application, make sure to build the Vue.js project for production using:
bash npm run build
This will generate a dist folder containing optimized and minified files ready for deployment.
5.2 Deploying the Vue.js Front-End:
You can deploy the Vue.js front-end using various hosting services like Netlify, Vercel, or Firebase Hosting. Simply upload the contents of the dist folder to the hosting service, and your front-end will be live.
5.3 Deploying Firebase Functions:
If your application requires server-side logic, you can use Firebase Cloud Functions. Deploy your functions to Firebase using the Firebase CLI:
bash firebase deploy --only functions
Conclusion:
Congratulations! You’ve successfully built a full-stack application by integrating Vue.js with Firebase. You’ve learned how to set up Firebase, create a real-time database, implement user authentication, and deploy the application. Vue.js and Firebase together provide a powerful combination for creating dynamic, real-time applications with ease. With the knowledge gained from this tutorial, you can now embark on building your own feature-rich full-stack applications. Happy coding!
Table of Contents
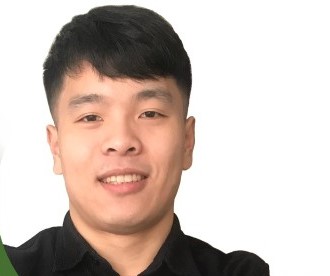
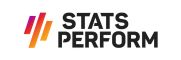