Creating Real-Time Applications with Vue.js and WebSockets
In today’s fast-paced digital world, users expect real-time interactions and updates from web applications. Whether it’s a live chat, collaborative editing, or real-time notifications, integrating real-time functionality can greatly enhance user experience. Vue.js, with its simplicity and reactivity, is an excellent choice for building modern web applications. When combined with WebSockets, a bidirectional communication protocol, you can easily create real-time applications that deliver information instantly to users.
Table of Contents
In this blog, we’ll explore the world of real-time applications and delve into the integration of Vue.js with WebSockets. We’ll walk you through the process of building a real-time chat application to demonstrate the concepts and practical implementation. So, let’s get started!
1. Understanding Real-Time Applications
1.1. What are Real-Time Applications?
Real-time applications are software programs that provide instantaneous data updates and interactions to users without requiring manual refreshes. These applications enable seamless two-way communication between the server and clients, ensuring that changes are propagated in real-time.
1.2. Benefits of Real-Time Applications
- Enhanced User Experience: Real-time updates create dynamic and interactive user interfaces, making the application feel more responsive and engaging.
- Improved Collaboration: Real-time collaboration features facilitate seamless communication and cooperation among users, promoting productivity and efficiency.
- Instantaneous Updates: Users receive immediate updates, eliminating the need to constantly check for new information manually.
- Better Data Synchronization: Real-time applications ensure that data across clients remains in sync, avoiding conflicts and data discrepancies.
2. Introduction to Vue.js
2.1. Vue.js Overview
Vue.js is a progressive JavaScript framework that excels in building user interfaces. Its core library is focused on the view layer, making it lightweight and easy to integrate with other projects and existing applications. Vue.js is known for its simplicity, performance, and reactivity, as it efficiently manages the data flow and updates the DOM in real-time.
2.2. Why Choose Vue.js for Real-Time Apps?
Vue.js’ reactivity and component-based architecture make it an ideal choice for building real-time applications. The framework’s reactive data-binding mechanism automatically updates the UI when data changes, ensuring seamless real-time updates to users. Additionally, Vue.js allows for the creation of custom components that can be reused throughout the application, making the development process more efficient.
3. Introduction to WebSockets
3.1. WebSockets Explained
WebSockets is a protocol that enables bidirectional communication between the client and the server over a single, long-lived TCP connection. Unlike traditional HTTP requests, WebSockets facilitate real-time data transfer, allowing the server to push data to connected clients instantly. This persistent connection reduces latency and overhead, making it perfect for real-time applications.
3.2. Advantages of Using WebSockets
- Real-Time Communication: WebSockets enable instant communication, ensuring data updates reach clients as soon as they are available.
- Lower Latency: The persistent connection eliminates the need to repeatedly establish connections, reducing latency and improving responsiveness.
- Bi-Directional Data Transfer: Both the server and clients can send data, making real-time collaboration and updates possible.
- Scalability: WebSockets can handle a large number of concurrent connections, making them suitable for scalable real-time applications.
4. Setting Up the Development Environment
4.1. Installing Vue.js
To begin building our real-time application, let’s set up the development environment by installing Vue.js. Here’s how you can do it using npm:
bash npm install -g @vue/cli
4.2. Configuring a WebSocket Server
Next, we need a WebSocket server to handle real-time communication. There are several options available, such as Socket.io, ws, or uWebSockets. For simplicity, we’ll use the popular Socket.io library:
bash npm install socket.io
With the development environment ready, we can now proceed to build our real-time chat application.
5. Building a Real-Time Chat Application
5.1. Creating the Vue.js Project
Let’s start by creating a new Vue.js project using the Vue CLI:
bash vue create real-time-chat-app cd real-time-chat-app
5.2. Implementing WebSocket Connection
In the Vue.js project, we’ll establish a connection to the WebSocket server using Socket.io. First, import the library and connect to the WebSocket server in the main Vue component:
javascript // main.js import Vue from 'vue'; import App from './App.vue'; import io from 'socket.io-client'; const socket = io('http://localhost:3000'); // Replace with your WebSocket server URL Vue.config.productionTip = false; new Vue({ render: (h) => h(App), }).$mount('#app');
5.3. Managing Real-Time Data with Vue.js
Now that we have established the WebSocket connection, let’s manage real-time data in our Vue.js components. For this example, we’ll create a simple chat component that displays incoming messages:
vue <!-- Chat.vue --> <template> <div> <div v-for="message in messages" :key="message.id"> <strong>{{ message.sender }}:</strong> {{ message.text }} </div> </div> </template> <script> export default { data() { return { messages: [], }; }, mounted() { socket.on('message', (message) => { this.messages.push(message); }); }, }; </script>
6. Enhancing the Real-Time Chat Application
6.1. Implementing User Authentication
To enhance security and prevent unauthorized access, we can implement user authentication. Users will need to log in before participating in the chat. For simplicity, let’s use a basic login component:
vue <!-- Login.vue --> <template> <div> <input type="text" v-model="username" placeholder="Enter your username" /> <button @click="login">Log In</button> </div> </template> <script> export default { data() { return { username: '', }; }, methods: { login() { // Implement authentication logic // Once authenticated, emit the "login" event to the server socket.emit('login', this.username); }, }, }; </script>
6.2. Adding User Presence Indicators
To make the chat more interactive, we can display user presence indicators. Let’s update the Chat.vue component to show when users are online:
vue <!-- Chat.vue (updated) --> <template> <div> <div v-for="message in messages" :key="message.id"> <strong :style="{ color: isUserOnline(message.sender) ? 'green' : 'black' }" >{{ message.sender }}:</strong> {{ message.text }} </div> </div> </template> <script> export default { // ... methods: { isUserOnline(username) { // Implement a method to check user presence based on the server data // For example, if the server maintains an array of online users: // return onlineUsers.includes(username); }, }, }; </script>
6.3. Handling Typing Indicators
Let’s add typing indicators to show when a user is currently typing a message:
vue <!-- Chat.vue (updated) --> <template> <div> <div v-for="message in messages" :key="message.id"> <strong :style="{ color: isUserOnline(message.sender) ? 'green' : 'black' }" >{{ message.sender }}:</strong> {{ message.text }} </div> <div v-if="isTyping" class="typing-indicator">Someone is typing...</div> </div> </template> <script> export default { // ... data() { return { isTyping: false, }; }, mounted() { socket.on('typing', (isTyping) => { this.isTyping = isTyping; }); }, }; </script>
7. Deploying the Real-Time Chat Application
7.1. Preparing for Production
Before deploying the application, ensure that you’ve optimized the Vue.js project for production:
bash npm run build
7.2. Choosing a Hosting Service
To make the real-time chat application accessible to users, deploy it to a hosting service like Heroku, AWS, or Netlify.
Conclusion
In this blog, we explored the exciting world of real-time applications and learned how to create one using Vue.js and WebSockets. The combination of Vue.js’ reactivity and WebSocket’s real-time communication brings dynamic and seamless interactions to your web applications. By following the steps and code examples provided, you can now build your own real-time applications with ease, enhancing user experiences and enabling efficient collaboration.
So, go ahead and start building your real-time applications with Vue.js and WebSockets to join the league of modern, responsive, and interactive web developers! Happy coding!
Table of Contents
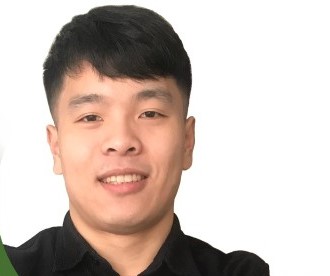
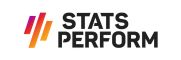